Results for the following term searched: java
More Stories
Why is my Spring @Autowired field null?
# Why is my Spring @Autowired field null? š± Welcome to another edition of our tech blog! Today, we're going to tackle a common issue that many developers face when using the Spring framework. You've probably encountered this frustrating problem before: y
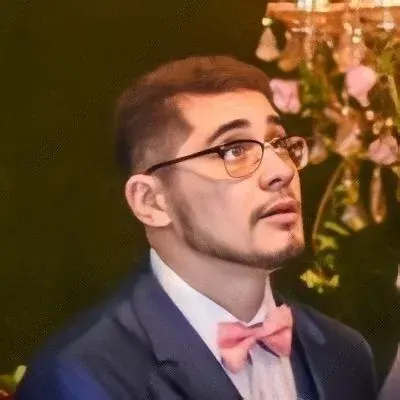
How do I invoke a Java method when given the method name as a string?
# How to Invoke a Java Method with a String Name š Hey there tech enthusiasts! Are you feeling curious about how to invoke a Java method when all you have is the method name as a string? Don't worry, you've come to the right place! In this blog post, we'
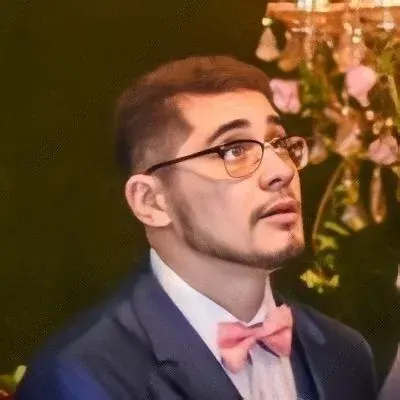
How to filter a Java Collection (based on predicate)?
# Filtering a Java Collection like a Boss šš So, you want to filter a Java Collection based on a predicate? Well my friend, you've come to the right place! š In this blog post, I'm going to show you some easy solutions to this common problem, and trust
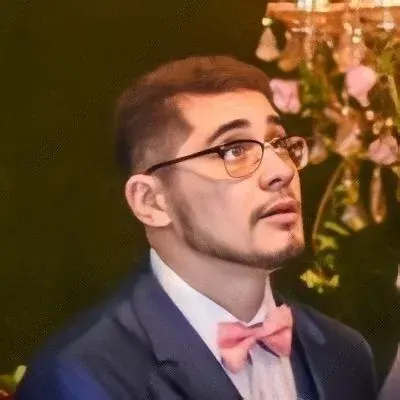
How to append text to an existing file in Java?
## Appending Text to an Existing File in Java: A Simple Guide ššš» So, you need to append text repeatedly to an existing file in Java? No worries, I got you covered! š Appending text to a file is a common requirement in many Java applications. Whether
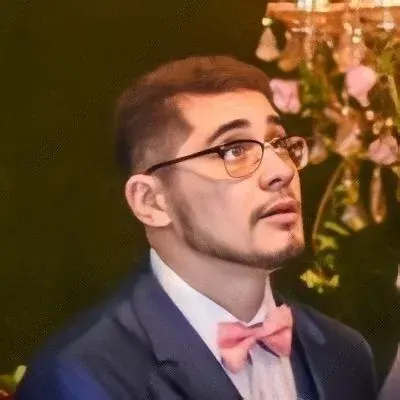
What is the difference between == and equals() in Java?
# What is the difference between == and equals() in Java? šš¤ So you want to know the difference between `==` and `equals()` in Java? š¤ Don't worry, dear reader, I've got you covered! Let's dive into this commonly misunderstood topic and shed some light
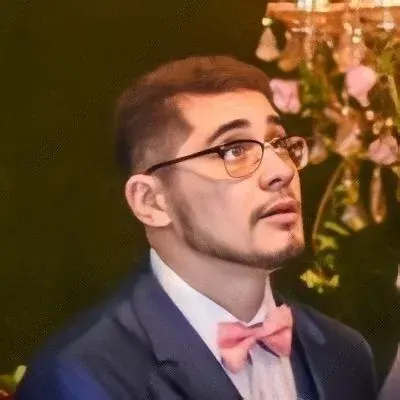
What is a stack trace, and how can I use it to debug my application errors?
# š§ Understanding Stack Trace to Debug Application Errors š§ <p>Oh no! š± You've encountered an error in your application and you're just seeing a confusing message like this:</p> <pre><code>Exception in thread "main" java.lang.NullPointerException
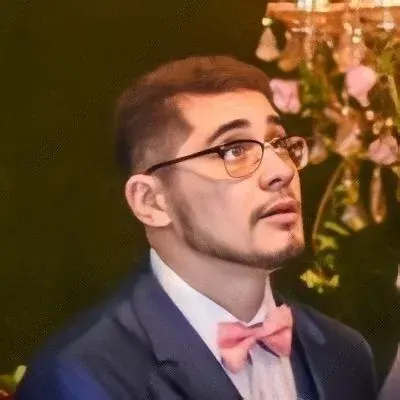
Simple way to repeat a string
# Simple Way to Repeat a String: Skip the For Loop and Stay Clever! šš Are you tired of using for loops to repeat a string? Looking for a smarter and more direct method? Well, you've come to the right place! In this blog post, we'll explore a simple yet
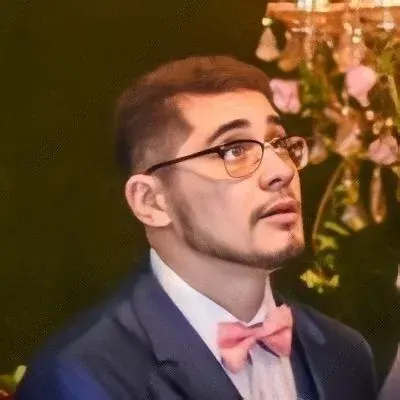
How to get the last value of an ArrayList
# How to Get the Last Value of an ArrayList šš” Are you tired of searching for a simple and effective way to retrieve the last value of an ArrayList? šš Look no further! In this blog post, we will explore the common issues and provide easy solutions to
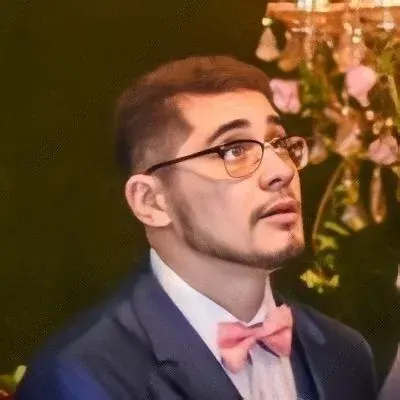
Why does array[idx++]+="a" increase idx once in Java 8 but twice in Java 9 and 10?
# Why does array[idx++]+="a" increase idx once in Java 8 but twice in Java 9 and 10? š Have you ever come across code that works differently in different versions of Java? In this blog post, we'll explore the mysterious behavior of the `+=` operator on
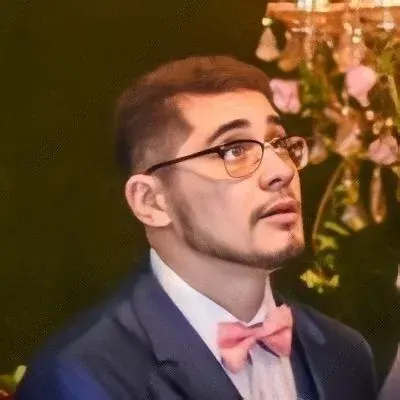
How do I convert from int to String?
# Converting from int to String: Breaking Down the Mysterious "+" Operator š§ So, you find yourself amidst a confusing project, scratching your head as you encounter this peculiar way of converting an `int` to a `String` in Java: ```java int i = 5; Strin
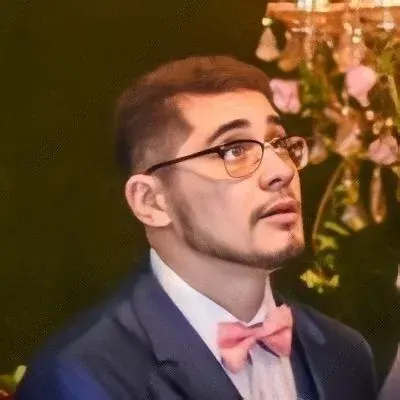