How to append text to an existing file in Java?
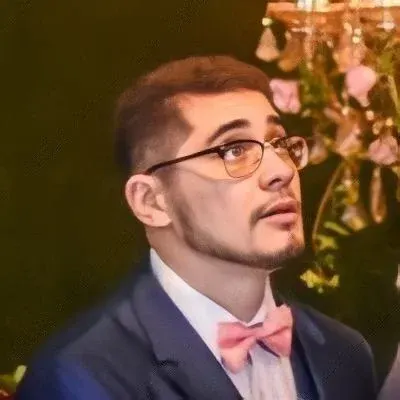
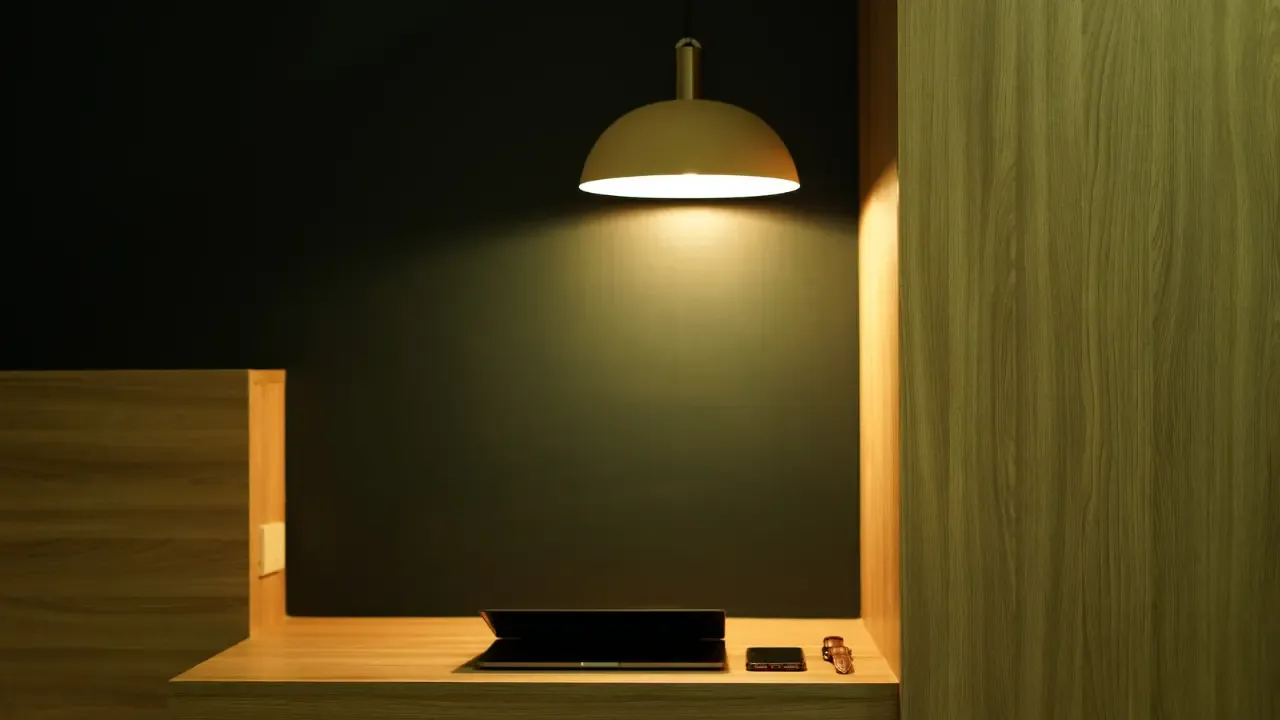
Appending Text to an Existing File in Java: A Simple Guide 📝🔗💻
So, you need to append text repeatedly to an existing file in Java? No worries, I got you covered! 🙌
Appending text to a file is a common requirement in many Java applications. Whether you're logging user interactions, storing data in a database, or just updating a configuration file, knowing how to append text is an essential skill!
Here's a step-by-step guide to help you append text to an existing file in Java, along with some common issues you might encounter and easy solutions to overcome them. Let's dive in! 💪
Step 1: Prepare your environment 🛠️🚀
Before we start appending text to a file, make sure you have a development environment set up with Java installed. You can use any integrated development environment (IDE) like Eclipse or IntelliJ IDEA, or simply a text editor and the command line. Once you're good to go, we can move on to the next step! 👍
Step 2: Create a FileWriter object 📝✨
To append text to a file, we first need to create a FileWriter
object. The FileWriter
class allows us to write characters to a file, and with a small tweak, we can use it to append text as well.
Here's a sample code snippet to create a FileWriter
object for appending:
// Create a FileWriter object in append mode
FileWriter writer = new FileWriter("path_to_your_file.txt", true);
In the above code, make sure to replace "path_to_your_file.txt"
with the actual path to your file. The true
argument passed to the constructor enables append mode, so any text you write will be added to the existing content of the file.
Step 3: Write your text to the file 🖋️✏️
Now that we have a FileWriter
object set up, we can start appending text to the file. The process is as simple as calling the write
method on the FileWriter
object.
Here's an example of how to append text to a file:
// Append text to the file
writer.write("This is some text to append!");
In the above code, "This is some text to append!"
is the text you want to add to the file. Feel free to replace it with your desired text, or even dynamically generate the content based on your application's needs.
Step 4: Close the FileWriter object to save changes 📤💾
After appending the text, it's important to close the FileWriter
object to ensure that any changes made to the file are saved.
Here's how you can close the FileWriter
object:
// Close the FileWriter object
writer.close();
Closing the FileWriter
object also frees up system resources, so it's good practice to close it when you're done with it.
Common Issues and Easy Solutions 💡🔧
Issue 1: File not found 🚫📂
If you encounter a FileNotFoundException
while trying to append text to a file, double-check the file path. Make sure the file exists in the specified location and that the path is correct. Also, ensure that your application has the necessary read and write permissions for that file.
Issue 2: Text overwritten instead of appended 🔄📝
If you notice that the text you write is overwriting the existing content of the file rather than being appended, make sure you pass true
as the second argument to the FileWriter
constructor, as shown in Step 2. This enables append mode and ensures the text is added at the end of the file.
Your Turn! ✍️👨💻
Appending text to an existing file in Java is now within your grasp! Just follow the steps outlined above, and you'll be appending text like a pro in no time. 🚀
Now it's your turn to try it out! Grab your favorite Java IDE or text editor, and experiment with appending text to a file. Use the code snippets provided and don't forget to close the FileWriter
object. Feel free to share your experience or any questions you may have in the comments below. I can't wait to hear about your success! 😄🎉
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
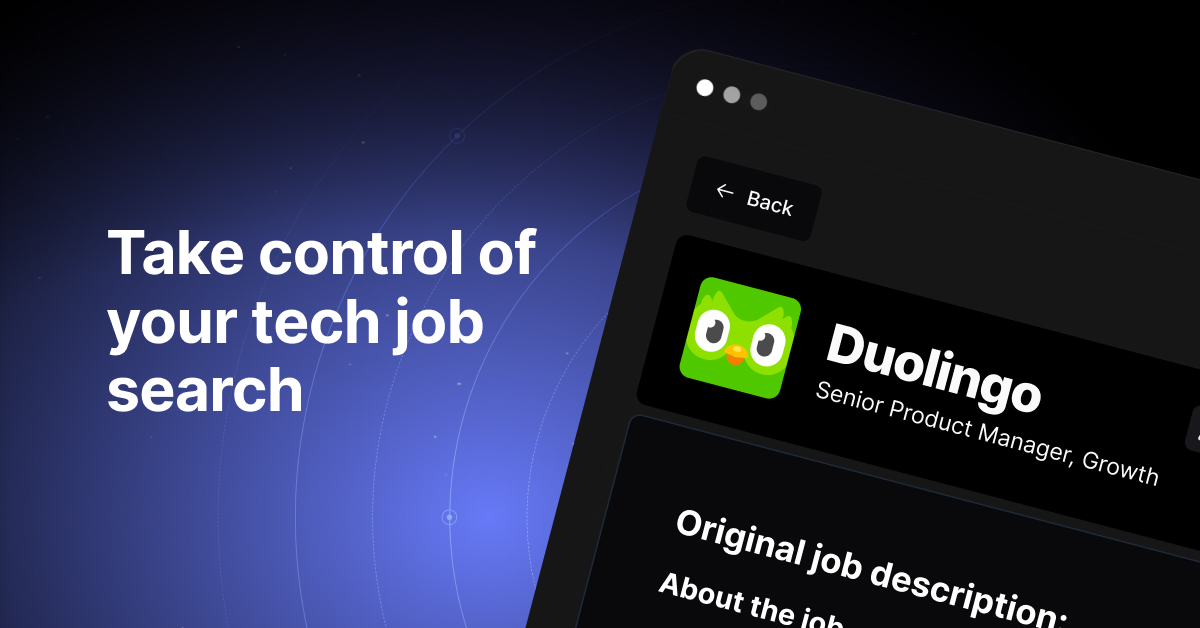