Best way to strip punctuation from a string
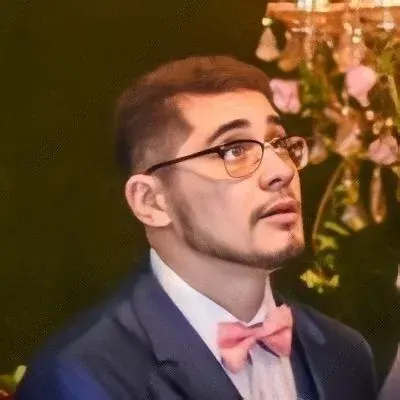
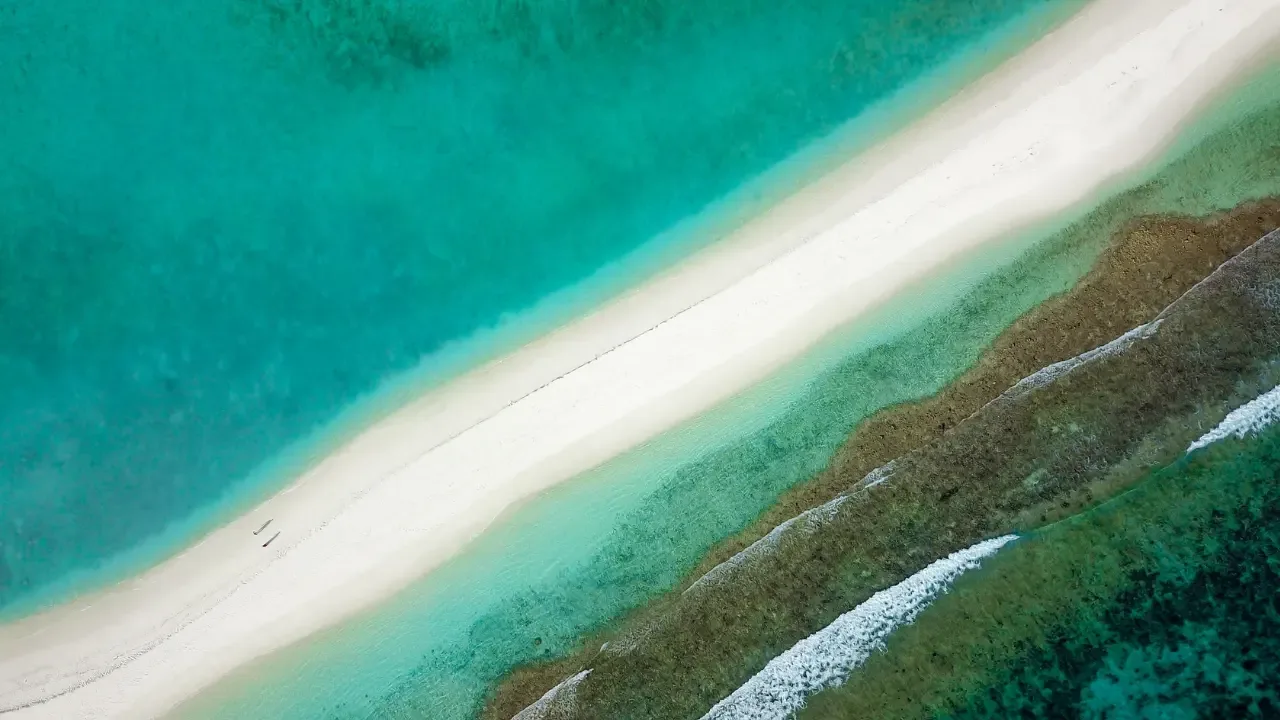
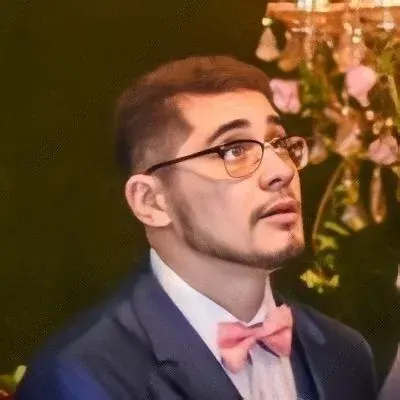
The Art of Stripping Punctuation: Simplifying Your Strings ๐ฅโ๏ธ
Are you tired of dealing with pesky punctuation marks that cause chaos in your strings? Have no fear, for we have a solution that will strip those buggers away and leave your texts clean and pristine! ๐งน๐
You might be familiar with the struggle of removing punctuation from a string. Traditionally, people have turned to the translate
function from the string
module in Python. While this method is effective, it can be a bit convoluted and confusing, leaving one to think, "There must be an easier way, right?!" ๐ญ๐ค
Well, fret not! We're here to introduce you to a simpler and more elegant solution. Imagine a world where stripping punctuation from a string is as easy as pie! ๐ฅง๐งก
The Old-school Method:
Let's quickly recap the traditional approach:
import string
# Sample string with punctuation
s = "string. With. Punctuation?"
# Stripping punctuation using translate function
out = s.translate(str.maketrans("", "", string.punctuation))
This method involves utilizing the string.punctuation
attribute and str.translate()
function to remove the punctuation marks. While it does get the job done, it requires importing the string
module and using the maketrans()
function with empty replacements. Not exactly the most intuitive solution, huh? ๐โ๏ธ
The New and Shiny Solution:
Now, let's unveil the newer, simpler, and more intuitive approach to stripping punctuation:
import re
# Sample string with punctuation
s = "string. With. Punctuation?"
# Stripping punctuation using regular expressions
out = re.sub(r'[^\w\s]', '', s)
Voila! With the power of regular expressions (re
module), you can effortlessly remove punctuation from your string. ๐๐ฅ
In the snippet above, we utilize the re.sub()
function, which stands for "substitute." By using a regular expression pattern, we can target and replace any character that's not a word character (\w
) or whitespace (\s
). This means all the punctuation marks will be obliterated from your string, leaving behind only the alphanumeric characters and spaces. ๐๐ช
A Call to Adventure:
Now that you've discovered this fantastic and straightforward method for stripping punctuation, it's time to put it to use! โจโจ
Challenge yourself to find a string with punctuation and see the magic happen. Share your experience, examples, or any questions you have in the comments section below. Let's engage in a delightful conversation about the quirks and wonders of string manipulation! ๐๐
Remember, simplicity is key! So why waste time on complicated approaches when we can do it in one fell swoop? Let your strings be free of punctuational burden! ๐๐ซ
Stay tuned for more tech tips and tricks that'll make your coding adventures more enjoyable. Until next time, happy coding! ๐ฉโ๐ป๐
โจ Do you want to learn more about Python string manipulation techniques? Check out our comprehensive guide on Python String Manipulation: Tips and Tricks! โจ