How to filter a Java Collection (based on predicate)?
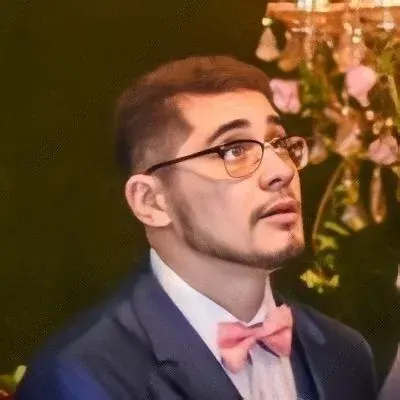
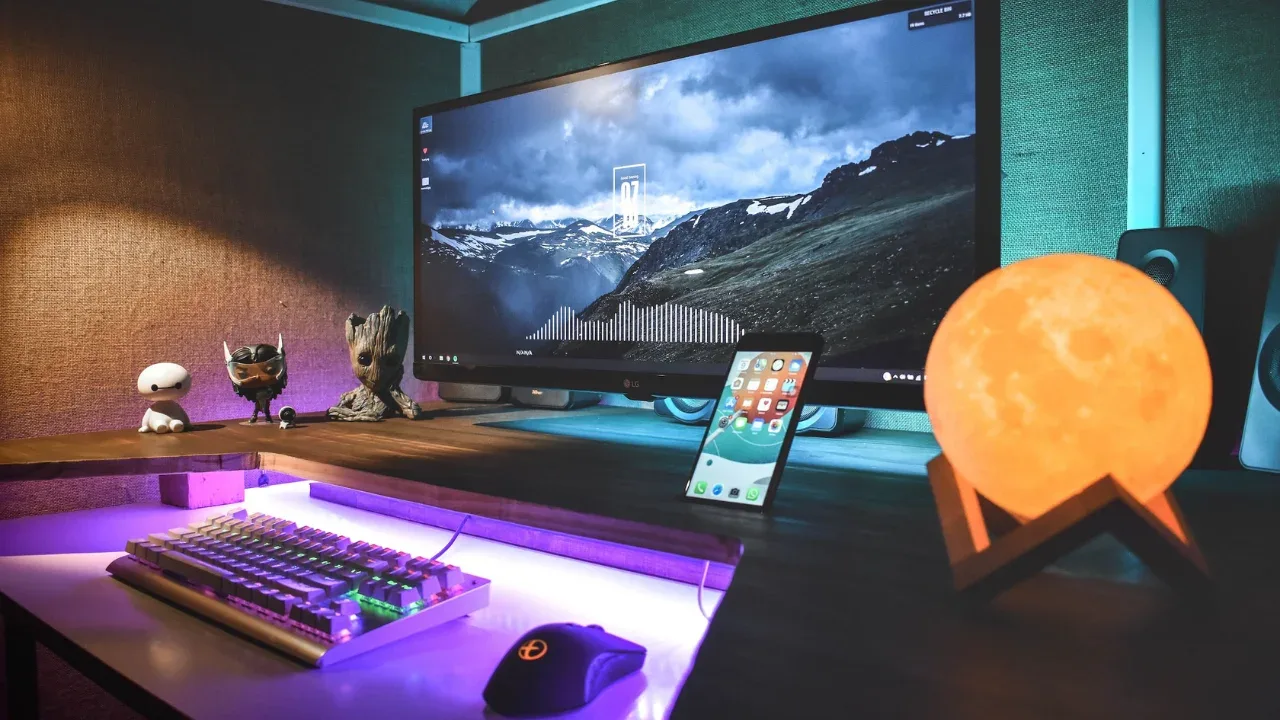
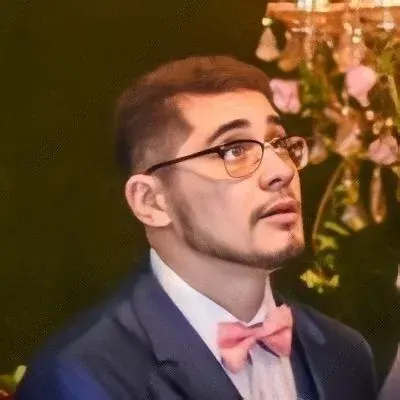
Filtering a Java Collection like a Boss 😎🔍
So, you want to filter a Java Collection based on a predicate? Well my friend, you've come to the right place! 🙌 In this blog post, I'm going to show you some easy solutions to this common problem, and trust me, it's going to be a breeze! 💨
The Problem 🧐
Let's say you have a Java Collection, whether it's a List, Set, or any other type, and you want to filter out certain elements based on a specific condition or criteria. Maybe you want to remove all the even numbers from a List, or keep only the strings that start with the letter "A" from a Set. How can you accomplish this? 🤔
The Solution 💡
Fear not, my friend, because Java has got your back! The Collection interface provides a nifty method called removeIf()
that allows you to filter elements based on a predicate. A predicate is simply a boolean-valued function that takes an element as an argument and returns true
or false
depending on whether the element satisfies the condition.
Here's how you can use the removeIf()
method to filter your Collection:
// Assuming you have a Collection called 'collection'
collection.removeIf(element -> /* your condition here */);
In the above code snippet, element
represents each individual element in your Collection, and you can replace /* your condition here */
with your own predicate implementation. For example, if you want to remove all even numbers from a List:
List<Integer> numbers = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10));
numbers.removeIf(number -> number % 2 == 0);
Simplify Your Life with Java 8 Streams 🌊💫
If you're using Java 8 or above, you can take advantage of the powerful Stream API to filter your Collection with even more elegance! 🌟
// Assuming you have a Collection called 'collection'
Collection<T> filteredCollection = collection.stream()
.filter(element -> /* your condition here */)
.collect(Collectors.toList());
In the above code snippet, filter()
is a method provided by the Stream API that takes a predicate and returns a new Stream containing only the elements that satisfy the condition. Finally, collect(Collectors.toList())
collects those filtered elements into a new List.
The Call-to-Action 🚀
Congratulations, my friend! You've now mastered the art of filtering a Java Collection like a boss! 👏 But don't stop here, there's a world of possibilities waiting for you in the realm of Java programming.
Why not try it out yourself? Take an existing Java Collection, apply one of the filtering techniques we discussed, and see the magic happen! Share your experience in the comments below and let's chat about the amazing things you can do with Java!
Happy coding! 😊💻