How to get the last value of an ArrayList
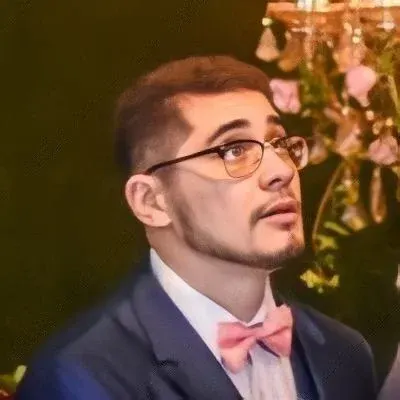

How to Get the Last Value of an ArrayList 😎💡
Are you tired of searching for a simple and effective way to retrieve the last value of an ArrayList? 🔄💭 Look no further! In this blog post, we will explore the common issues and provide easy solutions to help you get the job done quickly. So, let's dive right in! 🏊♀️🚀
The Problem 😓❓
Imagine you have an ArrayList full of values, and you need to access the last one for further processing or display purposes. 📊🔍 However, you find yourself scratching your head, wondering how to achieve this without writing complex code or resorting to lengthy iterations. 🤔🔄
The Traditional Approach 📜🔢
One way to approach this problem is by using traditional methods such as looping and indexing. You could iterate through the ArrayList and keep track of the last value encountered. However, this approach involves writing more code, and it might not be the most efficient solution. 🐢📝
The Solution 💡🔧
Luckily, Java provides a straightforward solution to this problem using built-in ArrayList methods. 😄🎉
Using the get() Method 🎯🤓
The get() method in the ArrayList class allows us to access a specific element based on its index. By using the size of the ArrayList minus one as the index, we can easily retrieve the last value. Here's a code snippet to demonstrate this: 📝✨
ArrayList<String> myList = new ArrayList<>();
myList.add("Value 1");
myList.add("Value 2");
myList.add("Value 3");
String lastValue = myList.get(myList.size() - 1);
System.out.println("The last value is: " + lastValue);
In the code snippet above, we created an ArrayList called myList
and added three values. By using the get()
method, we obtained the last value by passing myList.size() - 1
as the index. Finally, we printed the last value to the console. Easy-peasy! 🎩🐇
Using Java 8 Stream API 🌊🔍
If you are using Java 8 or higher, the Stream API provides an elegant and concise approach to getting the last value from an ArrayList. Here's an example: 🌟💻
ArrayList<String> myList = new ArrayList<>();
myList.add("Value 1");
myList.add("Value 2");
myList.add("Value 3");
String lastValue = myList.stream().reduce((first, second) -> second).orElse(null);
System.out.println("The last value is: " + lastValue);
In the code snippet above, we convert the ArrayList into a Stream and use the reduce()
method to continuously update the result until we reach the last value. Finally, we print the last value to the console. Stream-sational! 🎉🌈
Wrapping Up ✨🔚
Congratulations! You've learned two simple yet effective ways to retrieve the last value of an ArrayList in Java. 💪🌟 Whether you choose the traditional approach or opt for the Stream API, you can now confidently access that elusive last value without breaking a sweat. 💃💪
Don't forget to experiment with different scenarios and share your experiences in the comments below! If you found this blog post helpful, share it with your fellow developers. Spread the love and knowledge! ❤🔗
Happy coding! 🚀💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
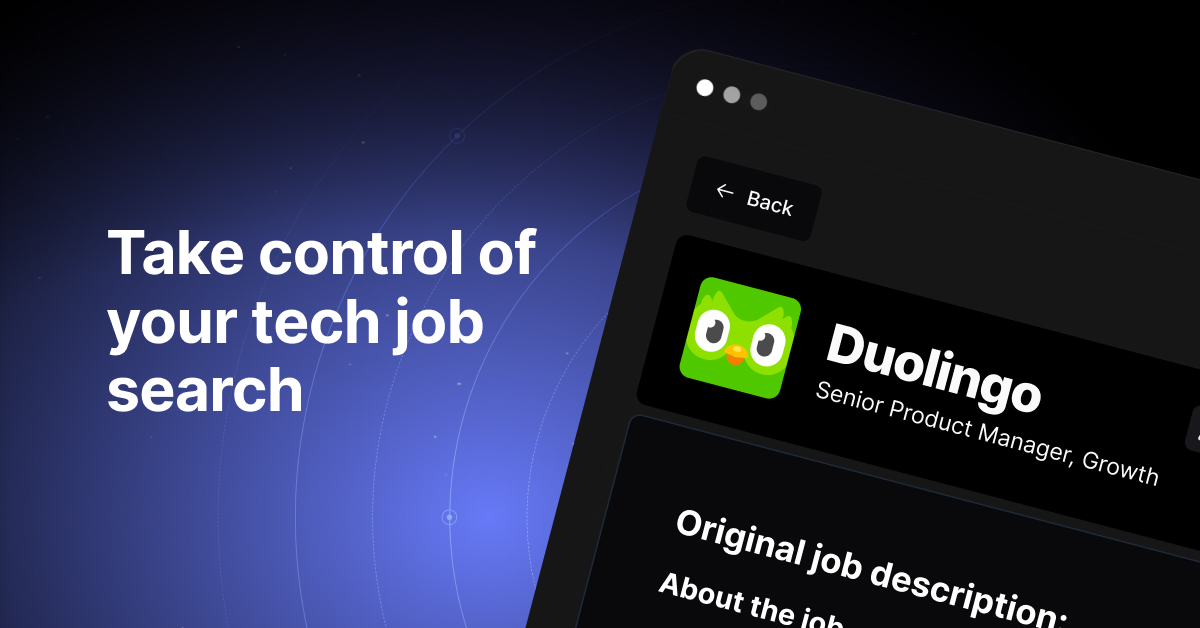