What is the difference between == and equals() in Java?
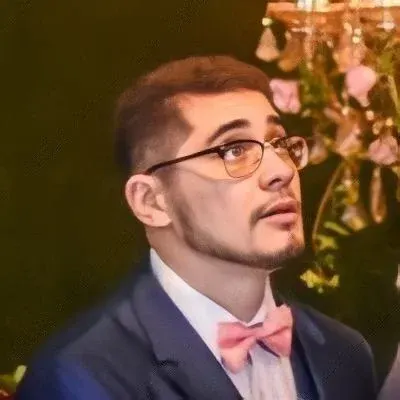
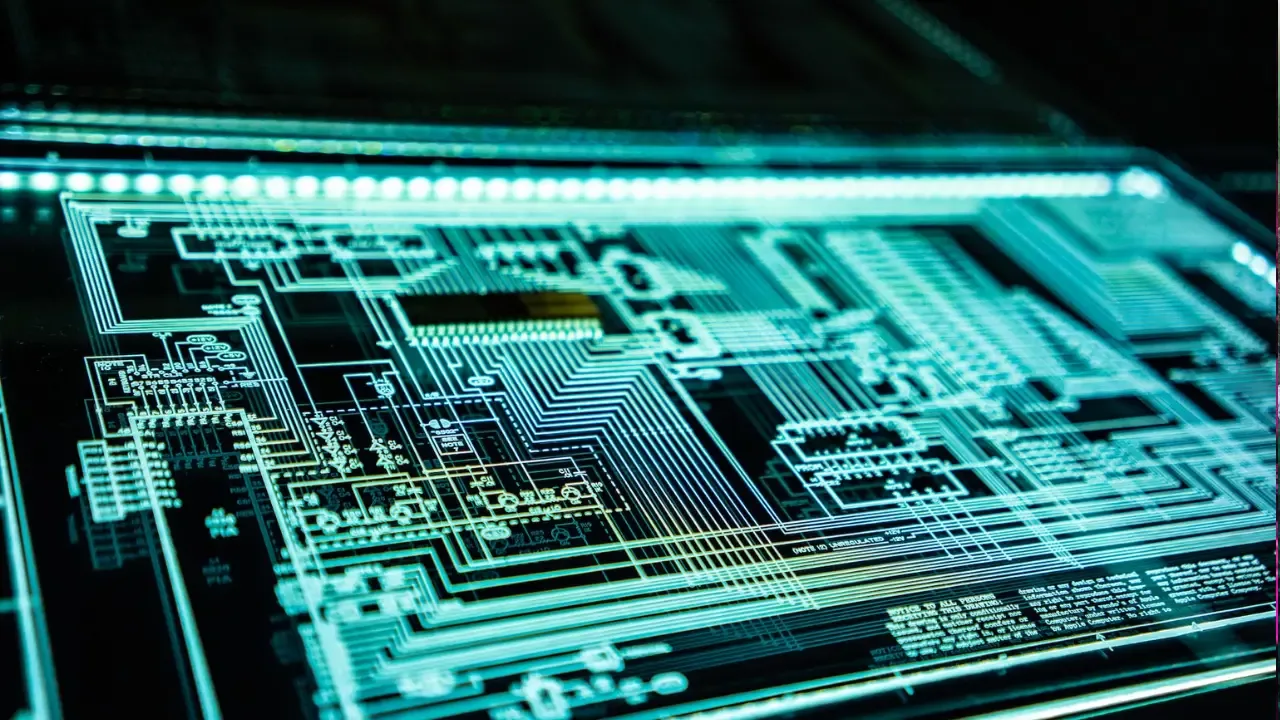
What is the difference between == and equals() in Java? 🔎🤔
So you want to know the difference between ==
and equals()
in Java? 🤔 Don't worry, dear reader, I've got you covered! Let's dive into this commonly misunderstood topic and shed some light on it. 💡
Understanding the Basics 📚
First things first! Let's clarify the basic definitions:
==
❗️: This is the equality operator in Java. It checks if two objects or variables are referring to the exact same memory location. It's like asking if two people live at the same house..equals()
❗️: This is a method provided by theObject
class in Java, used for content comparison. It checks if the values of two objects or variables are equal. It's like comparing the qualities and attributes of two people.
Examples to Illuminate the Difference 🌟
To better understand the difference between ==
and .equals()
, let's learn through some examples:
String str1 = "Java";
String str2 = "Java";
String str3 = new String("Java");
// Using ==
System.out.println(str1 == str2); // Prints true
System.out.println(str1 == str3); // Prints false
// Using .equals()
System.out.println(str1.equals(str2)); // Prints true
System.out.println(str1.equals(str3)); // Prints true
In the above code snippet, we declare three string variables: str1
, str2
, and str3
. Both str1
and str2
are created using string literals, while str3
is created using the new
keyword.
By using ==
to compare str1
and str2
, we get true
because they both refer to the same memory location. However, when comparing str1
and str3
, the result is false
as they are stored in different memory locations.
When using .equals()
, the comparisons focus on the content of the strings, and not their memory locations. Thus, both str1
and str3
are considered equal, resulting in true
for both comparisons.
Common Pitfalls to Avoid 😱
🚫 Pitfall 1: Forgetting to Override .equals()
🚫
Keep in mind that the default implementation of .equals()
in the Object
class checks for reference equality, much like using ==
. Therefore, if you want to perform content comparison, you need to override the .equals()
method in your own custom classes.
🚫 Pitfall 2: Null Pointer Exception 🚫
Take caution when using .equals()
with variables that could potentially be null
. Always remember to perform a null check before invoking .equals()
to avoid encountering a NullPointerException
.
Conclusion and Call-to-Action 📢
And there you have it, my tech-savvy amigos! You now understand the difference between ==
and .equals()
in Java. Remember, ==
checks for reference equality, while .equals()
compares the values of objects.
If you found this blog post helpful, don't forget to share it with your fellow developers! And if you have any further questions or want to share your own experiences, drop a comment below. Let's #TechTalk! 🤓💬
Keep coding and stay curious! Happy Java-ing! 👩💻🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
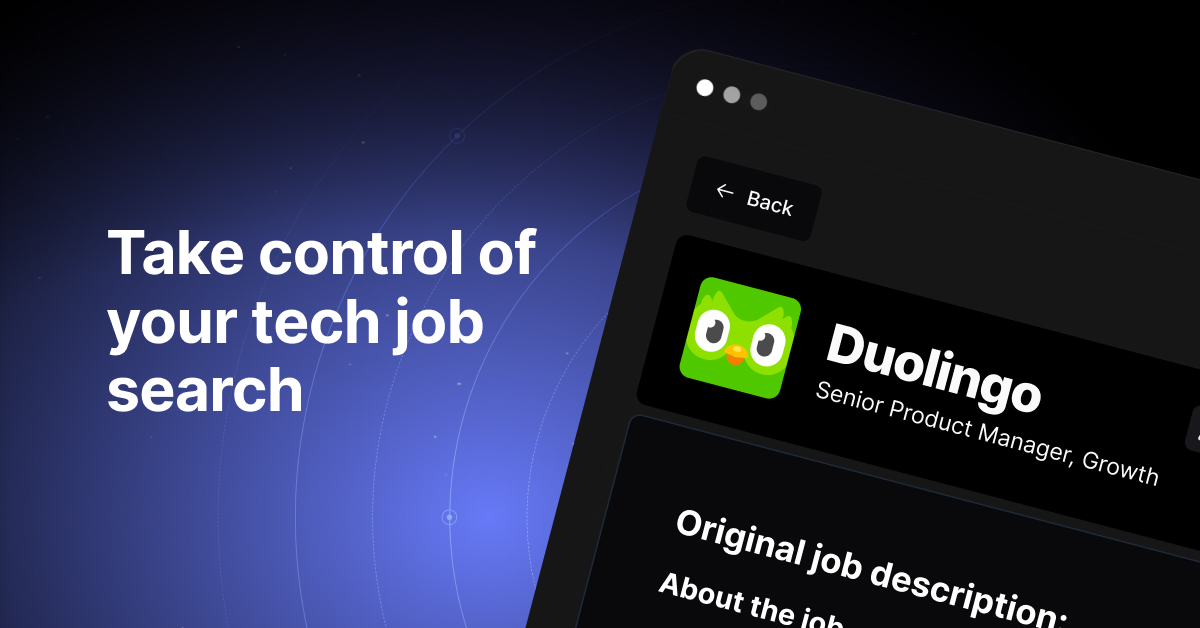