Why does array[idx++]+="a" increase idx once in Java 8 but twice in Java 9 and 10?
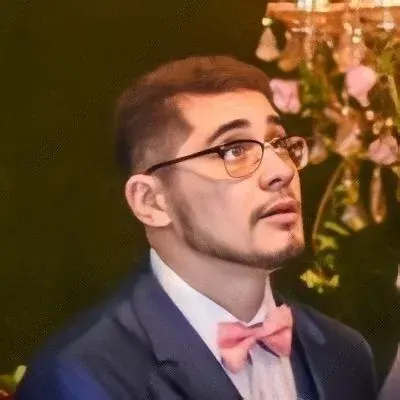
![Cover Image for Why does array[idx++]+="a" increase idx once in Java 8 but twice in Java 9 and 10?](https://images.ctfassets.net/4jrcdh2kutbq/376eUYzJAW7m4wV7PLqKoF/686c0d07d68f4a0bd05ecf1a1ec4bd01/Untitled_design__17_.webp?w=3840&q=75)
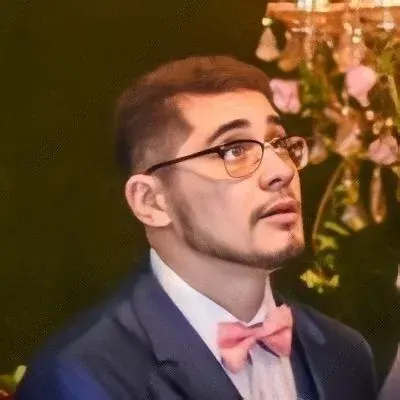
Why does array[idx++]+="a" increase idx once in Java 8 but twice in Java 9 and 10?
š Have you ever come across code that works differently in different versions of Java? In this blog post, we'll explore the mysterious behavior of the +=
operator on arrays in Java 8, Java 9, and Java 10. We'll walk you through the unexpected results and provide an easy solution. So, let's dive in!
The Challenge
š” A fellow code golfer, Kevin Cruijssen, created a challenging piece of code that involves incrementing the index while concatenating a string. Here's the code:
import java.util.*;
public class Main {
public static void main(String[] args) {
int size = 3;
String[] array = new String[size];
Arrays.fill(array, "");
for (int i = 0; i <= 100;) {
array[i++ % size] += i + " ";
}
for (String element: array) {
System.out.println(element);
}
}
}
š When running this code in Java 8, the output is as expected:
1 4 7 10 13 16 19 22 25 28 31 34 37 40 43 46 49 52 55 58 61 64 67 70 73 76 79 82 85 88 91 94 97 100
2 5 8 11 14 17 20 23 26 29 32 35 38 41 44 47 50 53 56 59 62 65 68 71 74 77 80 83 86 89 92 95 98 101
3 6 9 12 15 18 21 24 27 30 33 36 39 42 45 48 51 54 57 60 63 66 69 72 75 78 81 84 87 90 93 96 99
š But when running the same code in Java 10, the output is quite puzzling:
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50 52 54 56 58 60 62 64 66 68 70 72 74 76 78 80 82 84 86 88 90 92 94 96 98
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50 52 54 56 58 60 62 64 66 68 70 72 74 76 78 80 82 84 86 88 90 92 94 96 98 100 102
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50 52 54 56 58 60 62 64 66 68 70 72 74 76 78 80 82 84 86 88 90 92 94 96 98 100
š§ The numbering is entirely off in Java 10, while it works as expected in Java 8. So, what exactly is happening here? Is it a bug in Java 10?
Investigating the Issue
š To understand what's happening, let's take a closer look at the code. The line that requires our attention is:
array[i++ % size] += i + " ";
ā”ļø Here, the +=
operator is used to concatenate the string i + " "
to the element at the index i++ % size
in the array
.
Understanding the Difference
š To understand the difference between Java 8 and Java 9/10, we need to know how the +=
operator behaves when used on arrays.
š In Java 8, the +=
operator increments i
only once, regardless of how many times it is used on the same line. So, in our example code, i
is incremented just once, allowing us to get the expected output.
š However, starting from Java 9, the behavior of the +=
operator on arrays changed. It now increments i
multiple times if it is used on the same line. This change in behavior is the reason behind the unexpected results in Java 9 and 10.
Solutions to the Problem
š” Now, it's time to explore some solutions to the problem. Here are a couple of approaches you can follow:
Solution 1: Separate the Increment Operation
š Instead of using the +=
operator directly on the array element, you can separate the increment operation from the concatenation. Here's how the modified code looks:
for (int i = 0; i <= 100;) {
int index = i++ % size;
array[index] += i + " ";
}
š By separating the increment operation, you ensure that i
is incremented just once, resulting in the expected output in all versions of Java.
Solution 2: Use a Temporary Variable
š Another approach is to use a temporary variable to store the updated value of i
before concatenating it to the array element. Here's the modified code:
for (int i = 0; i <= 100;) {
int index = i % size;
array[index] += (i++) + " ";
}
š By storing the updated value of i
in a temporary variable, you avoid the multiple incrementations caused by the +=
operator. This solution also ensures the expected output across different versions of Java.
Wrapping Up
š We've explored the mysterious behavior of the +=
operator on arrays in Java 8, Java 9, and Java 10. We've seen that the change in behavior starting from Java 9 can lead to unexpected results when concatenating strings in a loop.
š Now that you know the difference and have two easy solutions at your disposal, you can confidently write code that works consistently across different versions of Java.
š Have you ever encountered a similar issue with code behaving differently in different Java versions? Share your experience in the comments below!
š¢ And don't forget to share this blog post with your fellow Java developers to help them understand and overcome this tricky issue!
Happy coding! š»š