How do I convert from int to String?
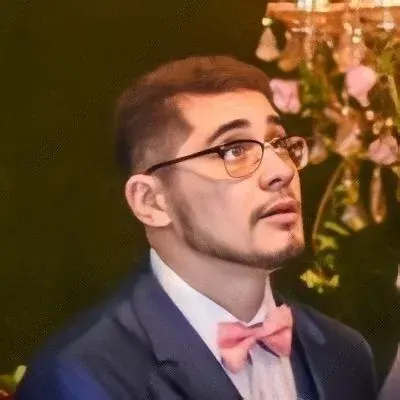
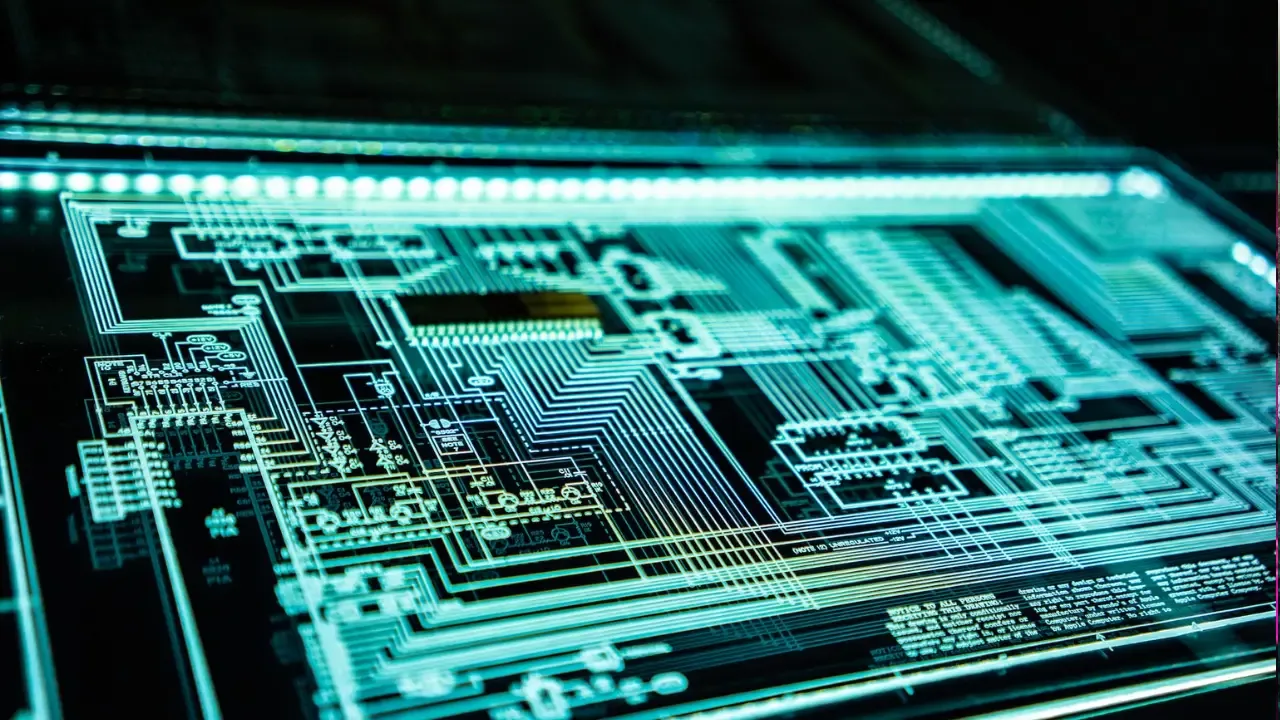
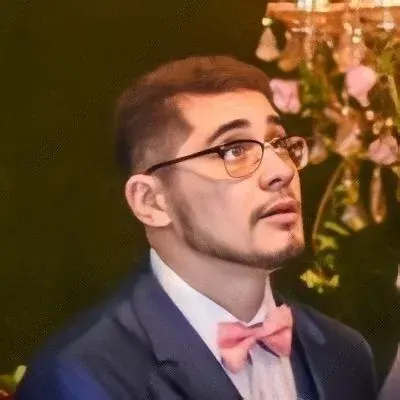
Converting from int to String: Breaking Down the Mysterious "+" Operator 🧐
So, you find yourself amidst a confusing project, scratching your head as you encounter this peculiar way of converting an int
to a String
in Java:
int i = 5;
String strI = "" + i;
Fear not, my friend! In this guide, we will dive into the depths of this mystery, unravel its secrets, and present you with simpler and more efficient solutions. 👩💻
The Curious Case of the "+" Operator 💭
Let's address your first question: Is this usual practice? Well, yes and no. The snippet you stumbled upon is a valid but not-so-ideal way to convert an int
to a String
in Java 🤔.
A Deeper Understanding of the "+" Operator 🔍
The magic behind the snippet lies in the behavior of the "+" operator when used with a String
and an int
. When one of the operands is a String
, the other operand is automatically converted to a String
as well.
In this case, the empty string ""
acts as a catalyst. When you concatenate an int
with an empty string, Java internally converts the int
into a String
. Hence, the int
value 5
is transformed into "5"
, which gets assigned to the variable strI
. 🪄
Although this approach works, it can be confusing and less efficient than other alternatives. Let's explore some better ways to handle this conversion conundrum. 🚀
Simplified Solutions for Converting from int to String 💡
1. Using the Integer.toString()
Method
One straightforward and widely accepted approach is to utilize the toString()
method provided by the Integer
class. Here's how:
int i = 5;
String strI = Integer.toString(i);
By calling Integer.toString(i)
, you explicitly convert the int
value i
into a String
representation.
2. Leveraging String Concatenation
If you prefer a more concise solution that doesn't involve direct method calls, you can use string concatenation with an empty string or another string. Check it out:
int i = 5;
String strI = String.valueOf(i);
Using String.valueOf(i)
achieves the same result as the previous approach but in a more compact manner. It also allows conversion from other primitive types or objects to a String
.
Embrace Simplicity, Embrace Efficiency! 🚀
Now that you've discovered simpler and more efficient ways to convert from int
to String
, it's time to level up your coding game! Say goodbye to the confusing "+" operator and embrace the explicit methods or string concatenation techniques shared in this guide. 🎉
If you found this guide helpful or have any other questions, don't hesitate to drop a comment below! Let's keep the conversation going. 👇