Simple way to repeat a string
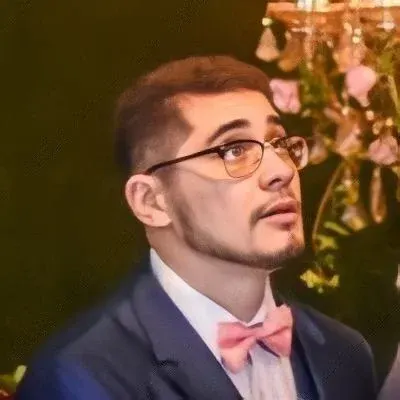

Simple Way to Repeat a String: Skip the For Loop and Stay Clever! 🔄📝
Are you tired of using for loops to repeat a string? Looking for a smarter and more direct method? Well, you've come to the right place! In this blog post, we'll explore a simple yet clever solution to the age-old problem of repeating a string without the hassle of for loops. 😎
The Common Approach: Looping It Out 🔄
Traditionally, if you wanted to repeat a string a certain number of times, you'd resort to using a for loop to achieve that. Example code snippet:
String str = "abc";
String repeated = "";
int n = 3;
for (int i = 0; i < n; i++) {
repeated += str;
}
repeated.equals("abcabcabc"); // true
While this method works perfectly fine, it has its downsides. First, for loops tend to increase the lines of code unnecessarily, even if they are tucked away in a separate function. Moreover, not everyone finds for loops intuitive. Understanding what's happening in a loop takes an extra effort, which can be a headache.
The Clever Alternative: String.repeat() 🌟
Thankfully, Java provides us with a simpler and more direct solution - the String.repeat()
method! 💡
String str = "abc";
String repeated = str.repeat(3);
repeated.equals("abcabcabc"); // true
With String.repeat(n)
, you can simply specify the number of times you want the string to be repeated. In this example, the string "abc" is repeated three times, resulting in "abcabcabc". Much cleaner, right? 😌
Benefits of Skipping the For Loop 💡
By embracing String.repeat()
, you enjoy a range of benefits:
Less code clutter: Eliminating the need for a for loop reduces the number of lines, making your code more concise and readable.
Improved readability: With
String.repeat()
, your intention is clear from the method call itself. No need to analyze for loop patterns or decipher unclear variable names.Avoiding clever bugs: We all know how tricky for loops can be. By avoiding them, you minimize the chances of introducing sneaky bugs that are challenging to find and fix.
Reduced scope-related bugs: For loops often reuse the same variables, increasing the chances of scoping bugs. With
String.repeat()
, you can avoid those pesky issues altogether.Easier debugging: By eliminating for loops, you reduce the number of places a bug hunter needs to examine, saving time and effort.
Take the Clever Route: String.repeat()! 🛤️
Now that you're armed with a smarter and more efficient way to repeat strings, it's time to put it into practice! The next time you need to repeat a string, remember the String.repeat()
method and enjoy the benefits of cleaner code, improved readability, and minimized bugs. 🚀
So, why stick to old-school for loops when you can navigate through the clever route with String.repeat()
? Give it a try and watch your code become sleeker and more elegant. Happy coding! 💻✨
📚 Related Resources:
If you want to dive deeper into this topic or seek further inspiration, check out these helpful resources:
Have fun exploring and discovering new ways to simplify your code! 🚀✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
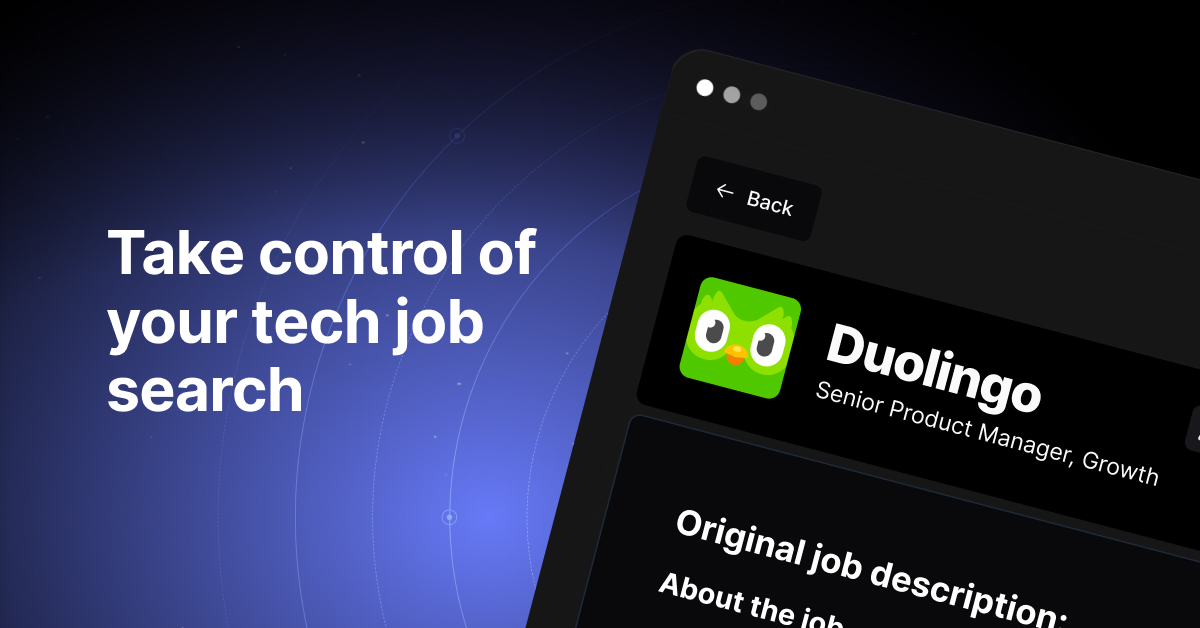