How do I invoke a Java method when given the method name as a string?
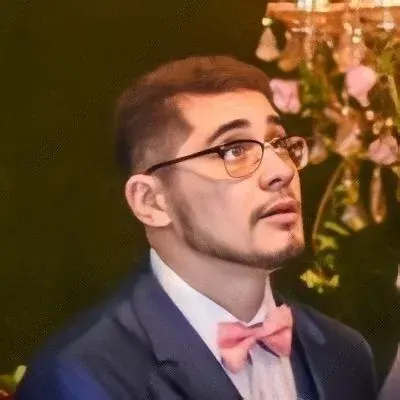
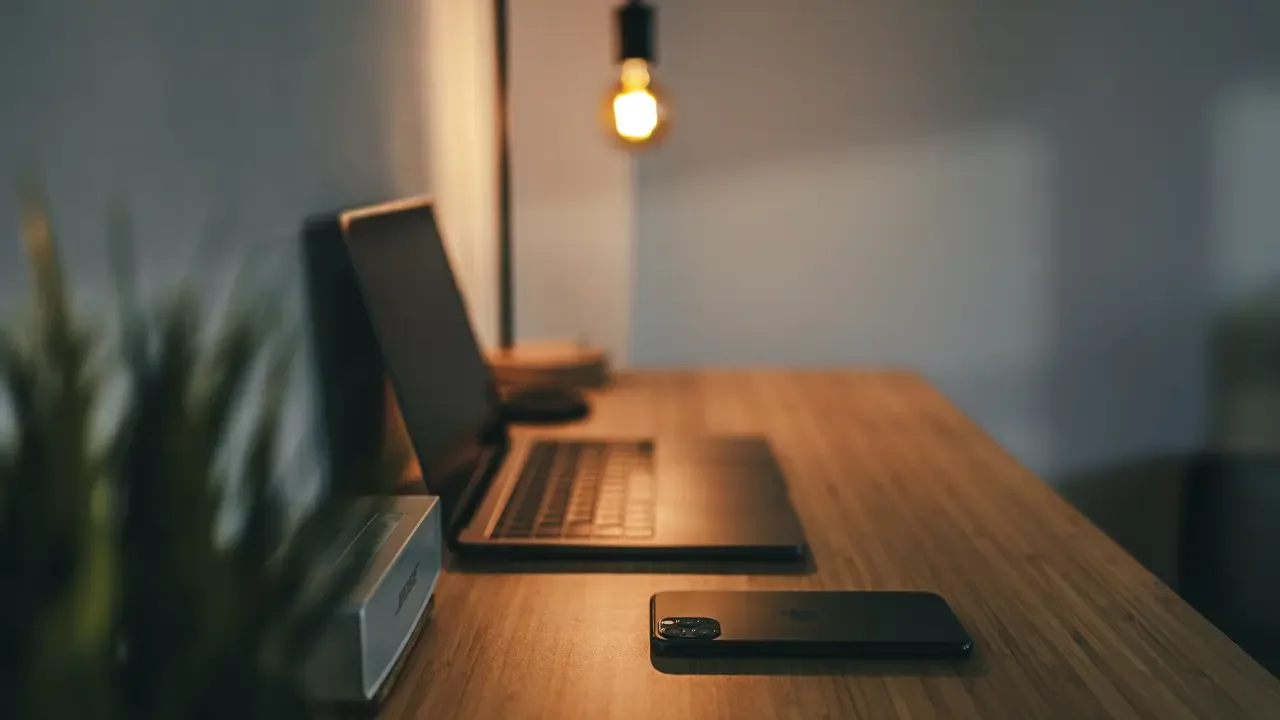
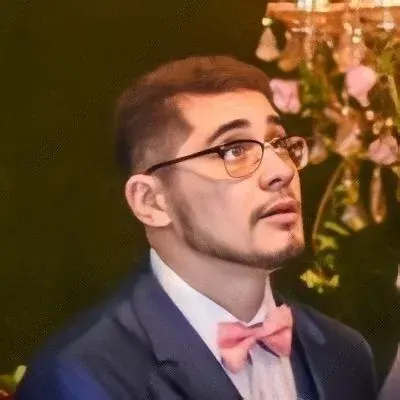
How to Invoke a Java Method with a String Name
š Hey there tech enthusiasts! Are you feeling curious about how to invoke a Java method when all you have is the method name as a string? Don't worry, you've come to the right place! In this blog post, we'll tackle this common problem and provide you with easy solutions. Let's dive right in! š
The Problem: Calling a Method Without Knowing the Object's Class
Imagine you have two variables, obj
and methodName
. The obj
variable holds an object, but you have no clue about its class. Meanwhile, methodName
stores the name of a method to be called on that object. š¤ So the million-dollar question is: How can you call a method on obj
when you don't know its class? Let's find out!
Solution 1: Reflection to the Rescue š
Fear not! Reflection is here to save the day! Using Java's reflection API, you can dynamically discover and invoke methods at runtime, even if you don't know the object's class in advance. š©
Here's an example that demonstrates how to accomplish this:
Object obj; // Your object goes here
String methodName = "getName";
try {
// Get the object's class
Class<?> objClass = obj.getClass();
// Find the desired method by name
Method method = objClass.getDeclaredMethod(methodName);
// Invoke the method on the object
Object result = method.invoke(obj);
// Do something with the method's return value
System.out.println("Returned value: " + result);
} catch (NoSuchMethodException | IllegalAccessException | InvocationTargetException e) {
// Handle any exceptions that may occur
e.printStackTrace();
}
Using the above code, you can call the method identified by methodName
on the obj
object, and even retrieve and utilize the return value. š
Solution 2: Apache Commons BeanUtils š
If you find yourself working with Java beans, Apache Commons BeanUtils library can be a great ally. It provides handy utility methods for manipulating Java bean properties, including invoking getter and setter methods by name.
To use BeanUtils, you need to include the library's dependency in your project, import the necessary classes, and then invoke the desired method like this:
import org.apache.commons.beanutils.BeanUtils;
Object obj; // Your object goes here
String methodName = "getName";
try {
// Get the return value of the method
String result = BeanUtils.getProperty(obj, methodName);
// Do something with the method's return value
System.out.println("Returned value: " + result);
} catch (IllegalAccessException | InvocationTargetException | NoSuchMethodException | NoSuchFieldException e) {
// Handle any exceptions that may occur
e.printStackTrace();
}
Conclusion and Your Next Steps! š
Congratulations! You now have two awesome solutions for invoking a Java method when you only have its name as a string. š Whether you prefer using reflection or the Apache Commons BeanUtils library, you are equipped with the knowledge needed to tackle this challenge.
Now, it's your turn to put this newfound knowledge into action! Why not try it out and see how it works in your own code? š» Don't forget to share your experiences, insights, or any other thoughts you may have in the comments section below. Let's keep the conversation flowing! š£ļø
Stay curious, keep learning, and happy coding! š©āš»šØāš»āØ