Why is my Spring @Autowired field null?
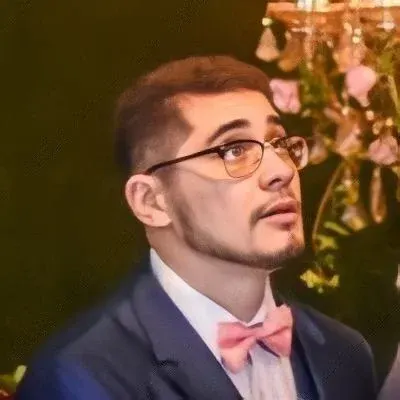
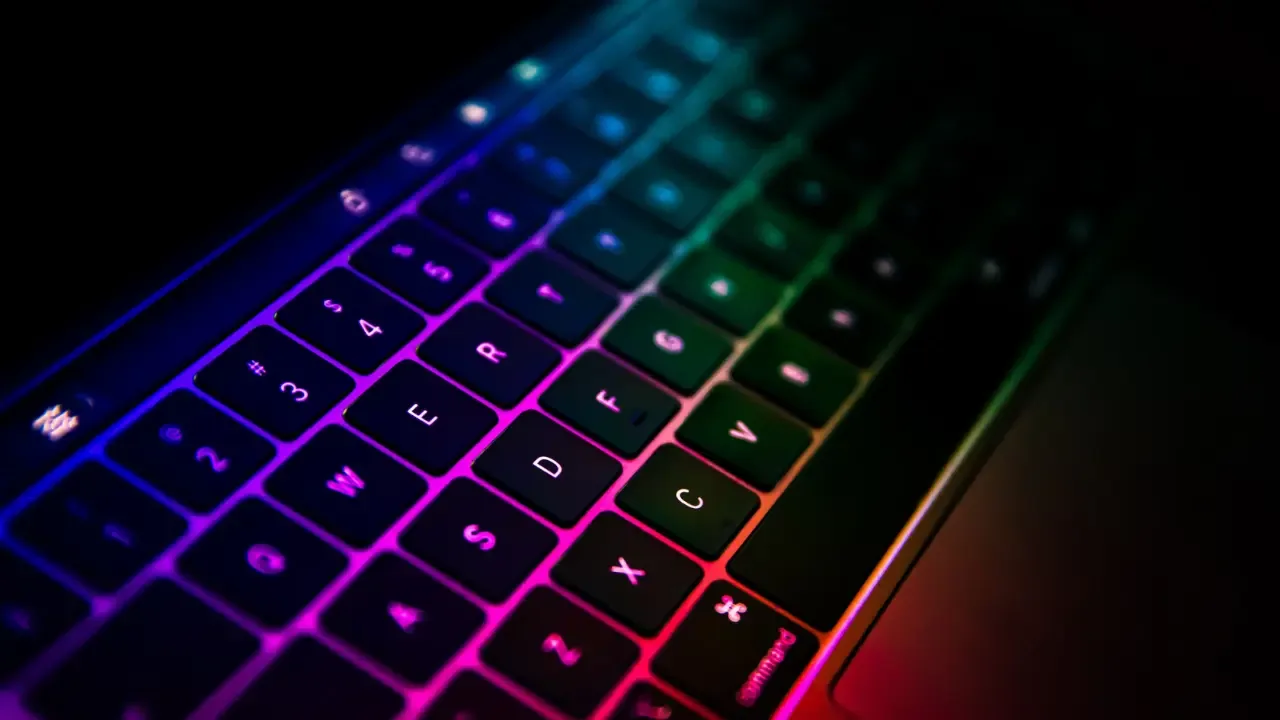
Why is my Spring @Autowired field null?
š± Welcome to another edition of our tech blog! Today, we're going to tackle a common issue that many developers face when using the Spring framework. You've probably encountered this frustrating problem before: your Spring @Autowired
field is null! š« But don't worry, we'll help you understand why this happens and provide easy solutions to get you back on track.
Understanding the Problem
In the context you've described, you have a Spring @Service
class called MileageFeeCalculator
with an @Autowired
field called rateService
. However, despite both the MileageFeeCalculator
and MileageRateService
beans being created, you're facing a NullPointerException
when invoking the mileageCharge
method on your service bean. So, what's causing this issue?
The Root of the Problem
The problem lies in your MileageFeeController
class. Let's take a closer look at your code:
@Controller
public class MileageFeeController {
@RequestMapping("/mileage/{miles}")
@ResponseBody
public float mileageFee(@PathVariable int miles) {
MileageFeeCalculator calc = new MileageFeeCalculator(); // š Problem here!
return calc.mileageCharge(miles);
}
}
As you can see, you're manually creating an instance of the MileageFeeCalculator
class instead of letting Spring handle it through dependency injection. This means that Spring isn't aware of your bean and can't autowire the rateService
field, resulting in a null value. š
The Solution
To fix this issue, you need to let Spring manage the creation of the MileageFeeCalculator
bean. Modify your MileageFeeController
class as follows:
@Controller
public class MileageFeeController {
@Autowired
private MileageFeeCalculator calc; // Use dependency injection
@RequestMapping("/mileage/{miles}")
@ResponseBody
public float mileageFee(@PathVariable int miles) {
return calc.mileageCharge(miles);
}
}
By using dependency injection with @Autowired
, Spring will create and wire the MileageFeeCalculator
bean for you, ensuring that the rateService
field is not null.
The Call-to-Action
We hope this guide helped you understand why your Spring @Autowired
field was null and how to fix it. If you found this blog post helpful, be sure to share it with your fellow developers who might be facing the same issue. Also, don't hesitate to leave a comment below if you have any questions or would like us to cover any other topics in future blog posts!
Stay tuned for more tech tips and tricks from our blog. Happy coding! šš»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
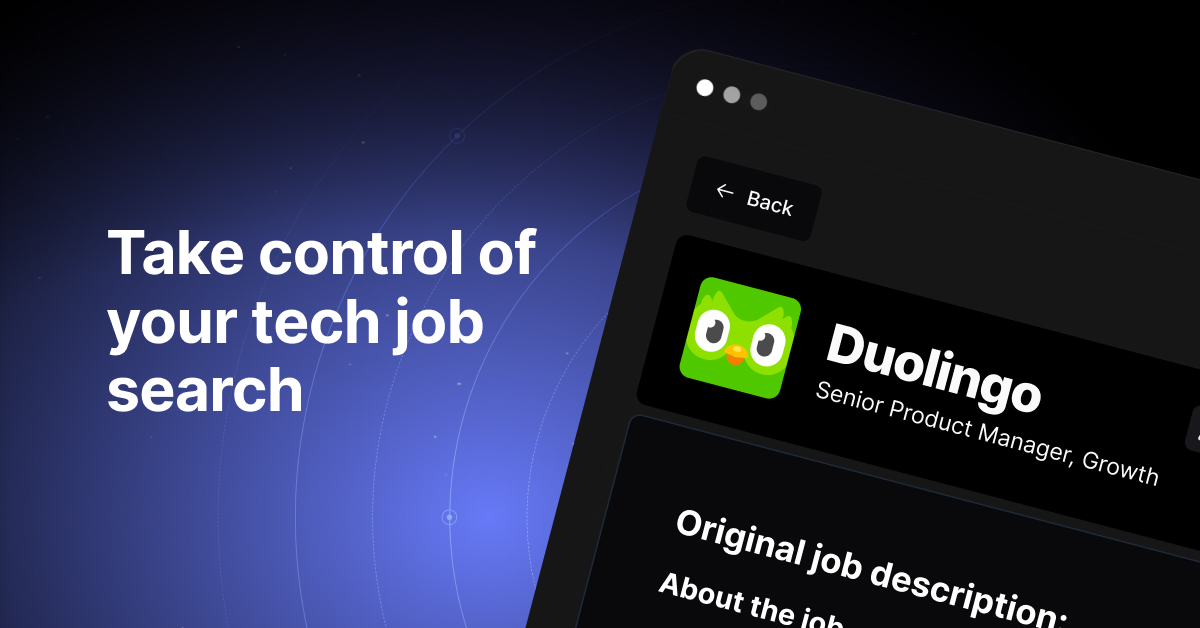