What is a stack trace, and how can I use it to debug my application errors?
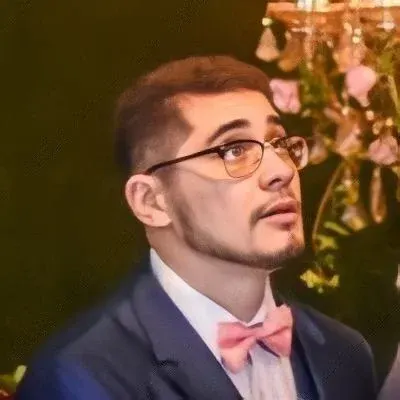
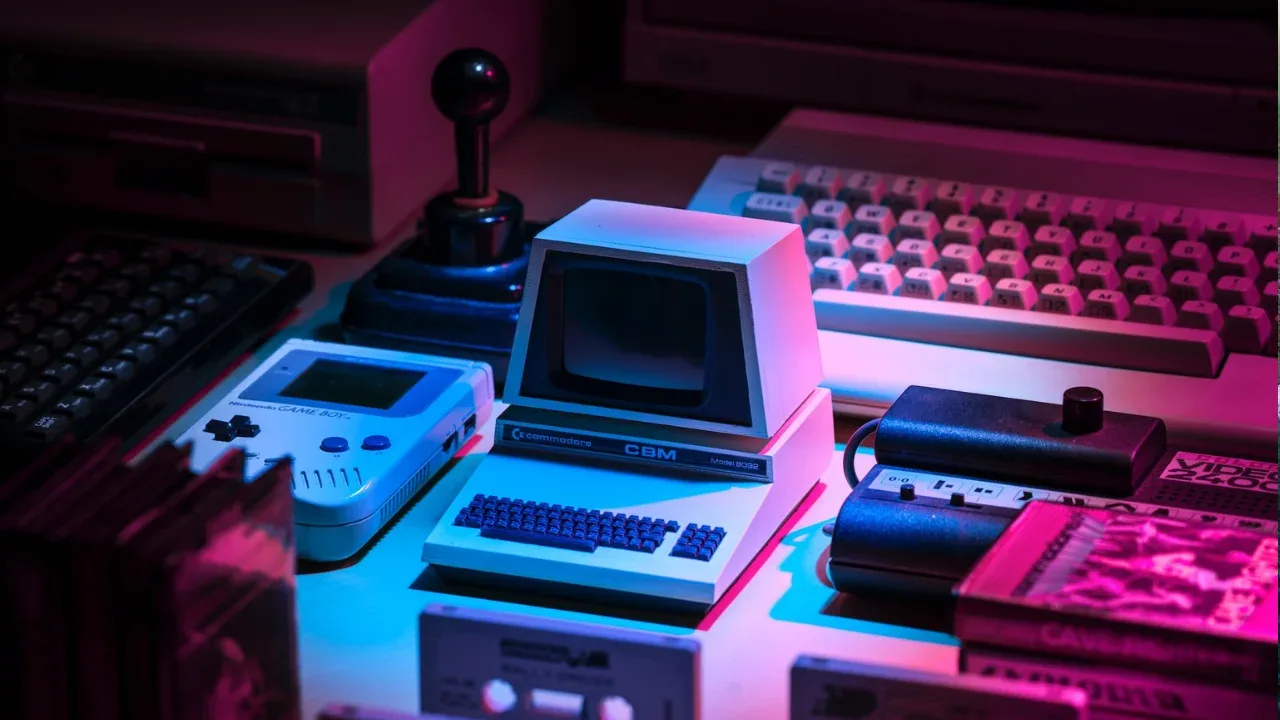
🚧 Understanding Stack Trace to Debug Application Errors 🚧
<p>Oh no! 😱 You've encountered an error in your application and you're just seeing a confusing message like this:</p>
<pre><code>Exception in thread "main" java.lang.NullPointerException at com.example.myproject.Book.getTitle(Book.java:16) at com.example.myproject.Author.getBookTitles(Author.java:25) at com.example.myproject.Bootstrap.main(Bootstrap.java:14) </code></pre>
<p>Don't worry, my friend! 🤗 This daunting message is called a **stack trace**. But what exactly does it mean? How can it help you to debug your application errors? Let's dive in and break it down! 💪</p>
🧐 What is a Stack Trace?
<p>A stack trace is a record of the sequence of method calls that led to an error or exception in your code. It provides crucial information about **where** the error occurred, so you can track it down and fix it effectively.</p>
<p>In the example above, you can see that the error is a **NullPointerException** which occurred in the **Book.java** file at line 16, inside the **getTitle()** method. This method was called by the **getBookTitles()** method in the **Author.java** file at line 25, which was in turn called by the **main()** method in **Bootstrap.java** at line 14.</p>
<p>With this information, you can see the **call chain** and pinpoint the exact location where the error occurred. 🎯</p>
🚧 How to Use a Stack Trace to Debug?
<p>Now that we understand what a stack trace is, let's see how we can leverage it to debug our application errors effectively.</p>
1️⃣ Analyze the Error Message
<p>The very first step is to read the error message carefully. It usually includes helpful information about the type of error and where it occurred.</p>
<p>In our example, the error message tells us that we encountered a **NullPointerException**. This means that we're trying to access an object that is `null` instead of having a valid value assigned to it.</p>
2️⃣ Locate the Error in the Stack Trace
<p>Next, locate the error in the stack trace. It is usually marked by a line that starts with "at" followed by the class name, method name, and line number.</p>
<p>In our example, the error occurred in the **getTitle()** method inside the **Book.java** file at line 16.</p>
3️⃣ Understand the Call Chain
<p>Look at the lines above the error line in the stack trace. These lines represent the **call chain** or the series of method invocations that led to the error.</p>
<p>In our example, the **getTitle()** method was called by the **getBookTitles()** method in the **Author.java** file at line 25, and so on.</p>
4️⃣ Check the Line Numbers
<p>Keep an eye on the line numbers mentioned in the stack trace. They help you navigate to the corresponding lines of code and identify potential issues.</p>
<p>For example, if we open the **Book.java** file and go to line 16, we might find a code snippet like this:</p>
<pre><code>public String getTitle() { return bookTitle; // line 16 } </code></pre>
<p>Here, it's clear that the **bookTitle** variable is not assigned a value, leading to a **NullPointerException** when we try to return it.</p>
5️⃣ Fix the Error
<p>Once you've identified the root cause of the error, make the necessary changes to fix it. This might involve checking for null values, adding proper error handling, or reviewing your code logic.</p>
<p>Continuing with our example, we can add a null check to ensure that **bookTitle** has a valid value before returning it:</p>
<pre><code>public String getTitle() { if (bookTitle != null) { return bookTitle; } else { return "Untitled"; } } </code></pre>
<p>By following these steps, you can effectively use a stack trace to debug your application errors! 💪</p>
📣 Call to Action: Share Your Stack Trace Success Story!
<p>Now that you're armed with the knowledge of how to interpret and utilize a stack trace, it's time to put it into action! 🚀</p>
<p>Next time you encounter an error, don't panic. Follow the steps outlined in this article and see how quickly you'll be able to diagnose and fix the issue.</p>
<p>And hey, if you've successfully debugged an error using a stack trace, share your story with us! We'd love to hear how it helped you and any additional tips you have. Happy coding! 😊</p>
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
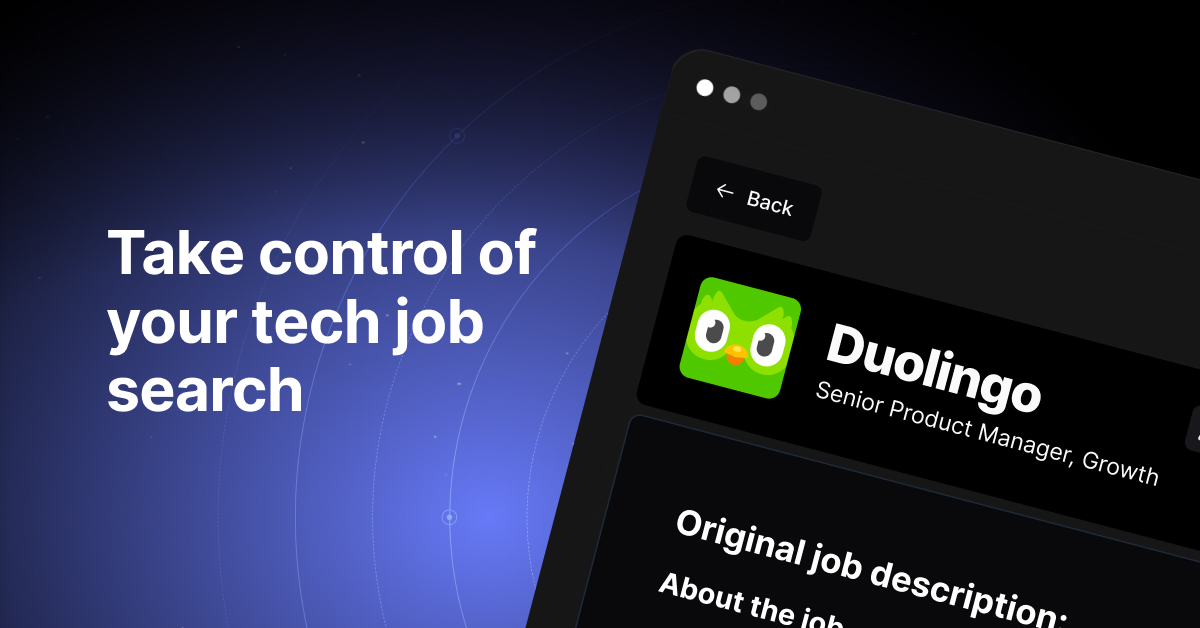