Results for the following term searched: java
More Stories
What is a serialVersionUID and why should I use it?
# What is a serialVersionUID and why should I use it? š¤ Have you ever encountered the warning message in Eclipse that says: > The serializable class [class name] does not declare a static final serialVersionUID field of type long. And you're probably w
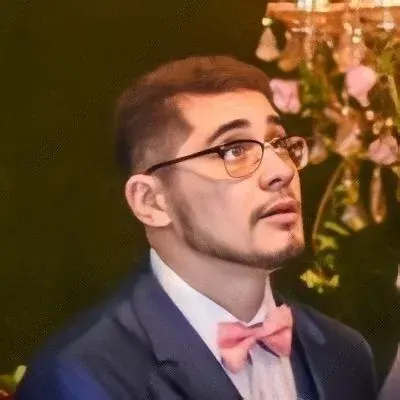
How do I test a class that has private methods, fields or inner classes?
# Testing Classes with Private Methods, Fields, or Inner Classes So, you have this awesome class you've been working on, and it has some private methods, fields, or even nested classes. Now, you're faced with the challenge of testing it using JUnit. Howev
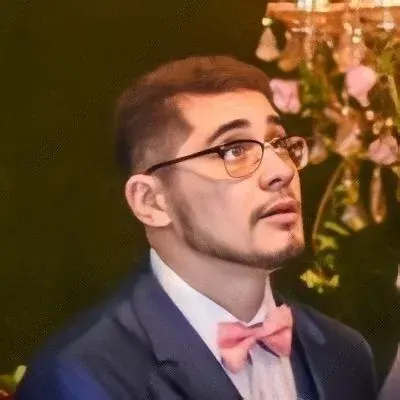
Initialization of an ArrayList in one line
# Initializing an ArrayList in One Line: The Easiest Way! š Are you tired of writing multiple lines just to initialize an ArrayList? š© Look no further! In this blog post, we will explore a super easy and concise way to initialize an ArrayList in just on
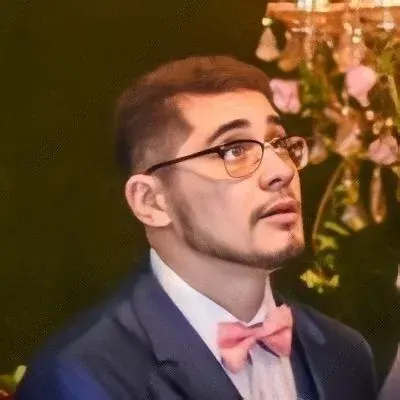
How do I convert a String to an int in Java?
# How to Convert a String to an int in Java: A Complete Guide So, you want to convert a `String` to an `int` in Java, huh? Well, don't worry, because I've got you covered! š ## The Problem Let's say you have a `String` containing a numeric value, like
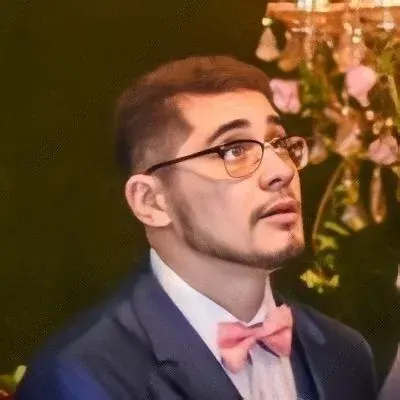
What is the difference between public, protected, package-private and private in Java?
## What's the Deal with Access Modifiers in Java? š¤ Are you diving into the realm of Java programming and find yourself confused about when to use those access modifiers? Don't worry, my tech-savvy friend, I've got you covered! In this blog post, we'll d
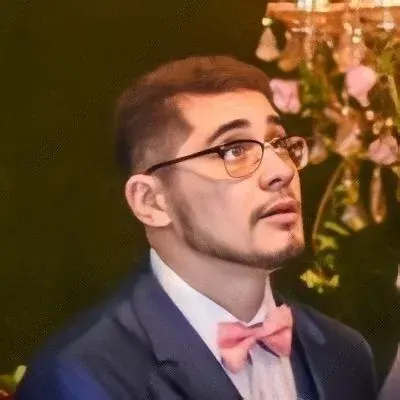
When to use LinkedList over ArrayList in Java?
# When to use LinkedList over ArrayList in Java? š As a Java developer, you might have come across the dilemma of choosing between `LinkedList` and `ArrayList` when working with lists. Both offer similar functionality, but they have different underlying
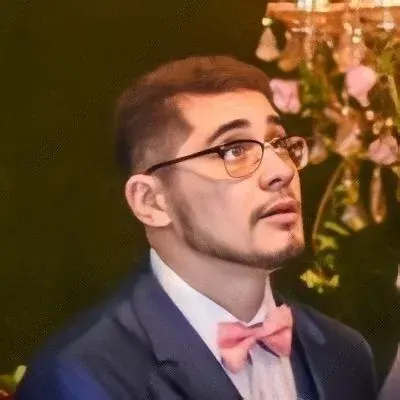
How can I create a memory leak in Java?
## Creating a Memory Leak in Java: A Beginner's Guide šØāš»š So, you just had an interview and you were asked to create a memory leak in Java? Don't worry! It's not as complicated as it sounds. In this blog post, we'll break it down for you, addressing c
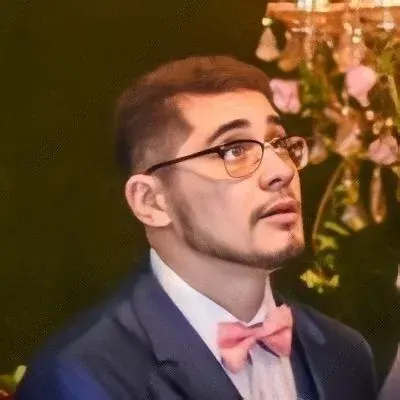
Iterate through a HashMap
# š Mastering HashMap Iteration in Java š Hey there, tech enthusiasts! Today, we're diving into the world of HashMap iteration in Java. If you've ever found yourself scratching your head, wondering how to efficiently loop through the items in a HashMap,
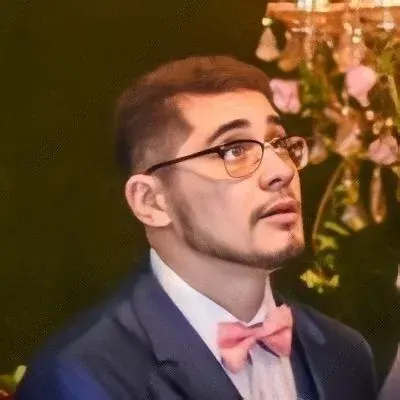
Why don"t Java"s +=, -=, *=, /= compound assignment operators require casting long to int?
# Why don't Java's +=, -=, *=, /= compound assignment operators require casting long to int? Ever wondered why you don't need to explicitly cast a `long` to an `int` when using compound assignment operators like `+=`, `-=`? Well, buckle up, because I'm a
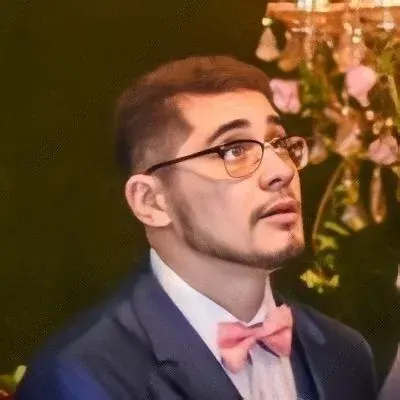
Proper use cases for Android UserManager.isUserAGoat()?
# Proper Use Cases for Android UserManager.isUserAGoat()? šš± Have you ever wondered what the `isUserAGoat()` method in the `UserManager` class in Android does? š¤ Don't worry, you're not alone. This method might seem odd and confusing at first glance, b
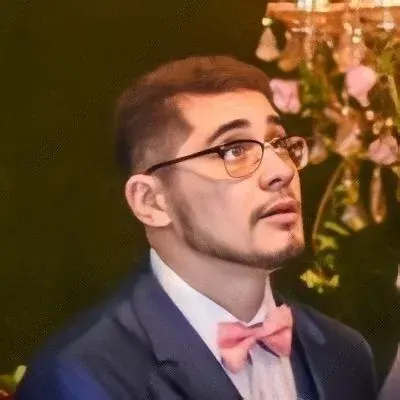