Why don"t Java"s +=, -=, *=, /= compound assignment operators require casting long to int?
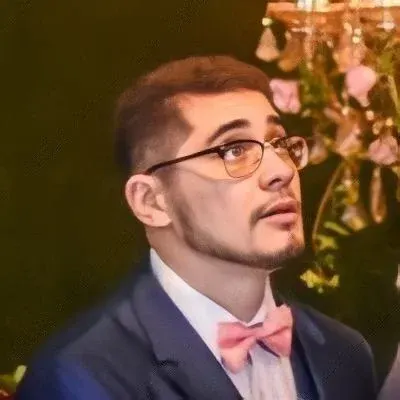
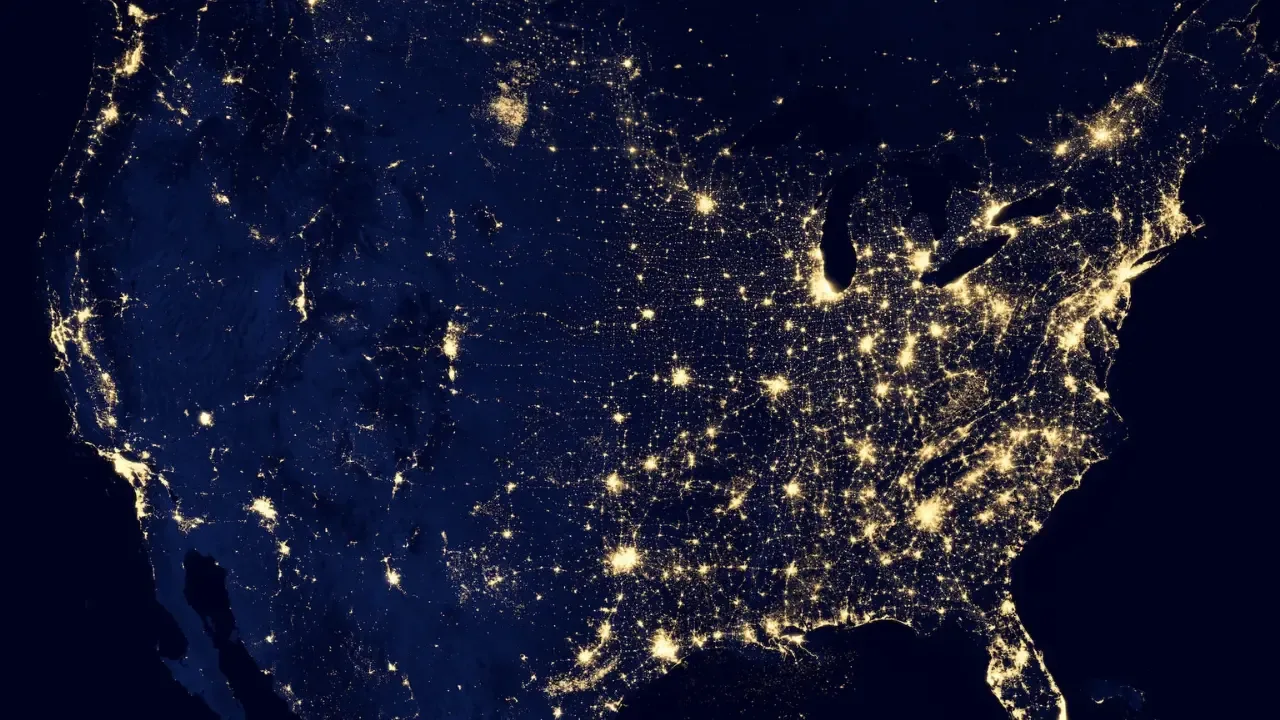
Why don't Java's +=, -=, *=, /= compound assignment operators require casting long to int?
Ever wondered why you don't need to explicitly cast a long
to an int
when using compound assignment operators like +=
, -=
? Well, buckle up, because I'm about to break it down for you in a way that even your grandma would understand 😎✨
Understanding the Basics
Before we dive in, let's quickly recap how compound assignment operators work. In Java, the syntax i += j;
is just a shortcut for i = i + j;
. It's a handy way to update the value of a variable by performing an operation on its current value.
The "Casting" Confusion
Now, you might think that when dealing with different data types, Java would throw a fit and demand explicit casting, right? Surprisingly, that's not the case here. 🤔
Consider this example:
int i = 5;
long j = 8;
If we try i = i + j;
, Java will throw a compilation error, complaining about incompatible types. But guess what? i += j;
will compile just fine. What gives? 🤷♀️
The Hidden Casting Secret
Here's the cool part: behind the scenes, Java is cleverly taking care of the casting for us! 😎🌟 Let's take a closer look at what actually happens when we use compound assignment operators.
When you write i += j;
, Java treats it as i = (int) (i + j);
. Notice that Java automatically casts the result of (i + j)
back to int
before assigning it to i
. 🤯
This automatic casting is known as narrowing primitive conversion. It's a process where Java automatically converts larger data types (like long
) to smaller ones (like int
) without us having to explicitly cast them.
The "Why" Behind It All
So, why does Java allow this automatic casting for compound assignment operators? It all comes down to the principle of ease and convenience. Java aims to make your life as a developer easier, and these operators are designed to simplify your code and save you precious typing time. ⚡️✍️
By automatically handling the casting for you, Java eliminates the need for repetitive and potentially error-prone casting operations. It's a smart and intuitive way of working with different data types while keeping your code concise.
Your Turn! 🚀
Now that you know the secret behind Java's compound assignment operators, it's time to put that newfound knowledge into action! Replace those verbose statements with snappy compound assignments, and watch your code become cleaner and more efficient. 😉
Don't forget to share this knowledge nugget with your fellow Java enthusiasts. And if you have any other mind-boggling tech questions, drop them in the comments below. Let's decode the tech world together! 💪✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
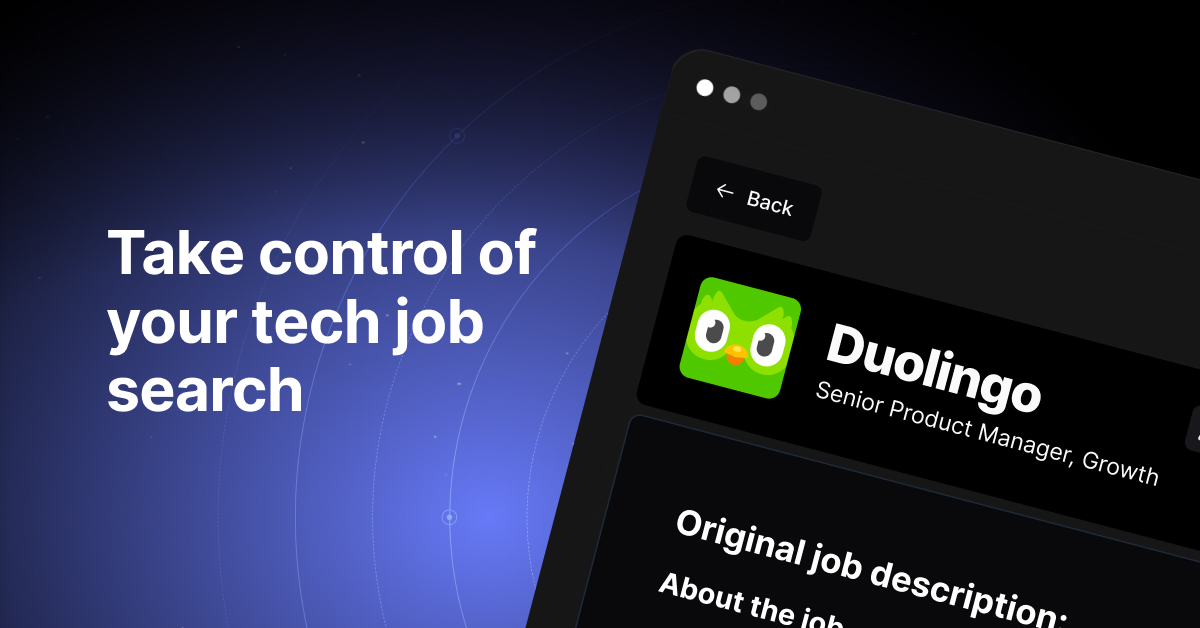