How can I create a memory leak in Java?
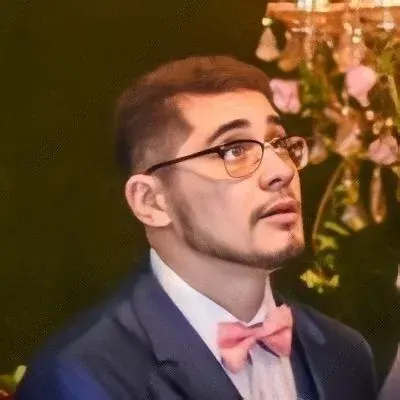
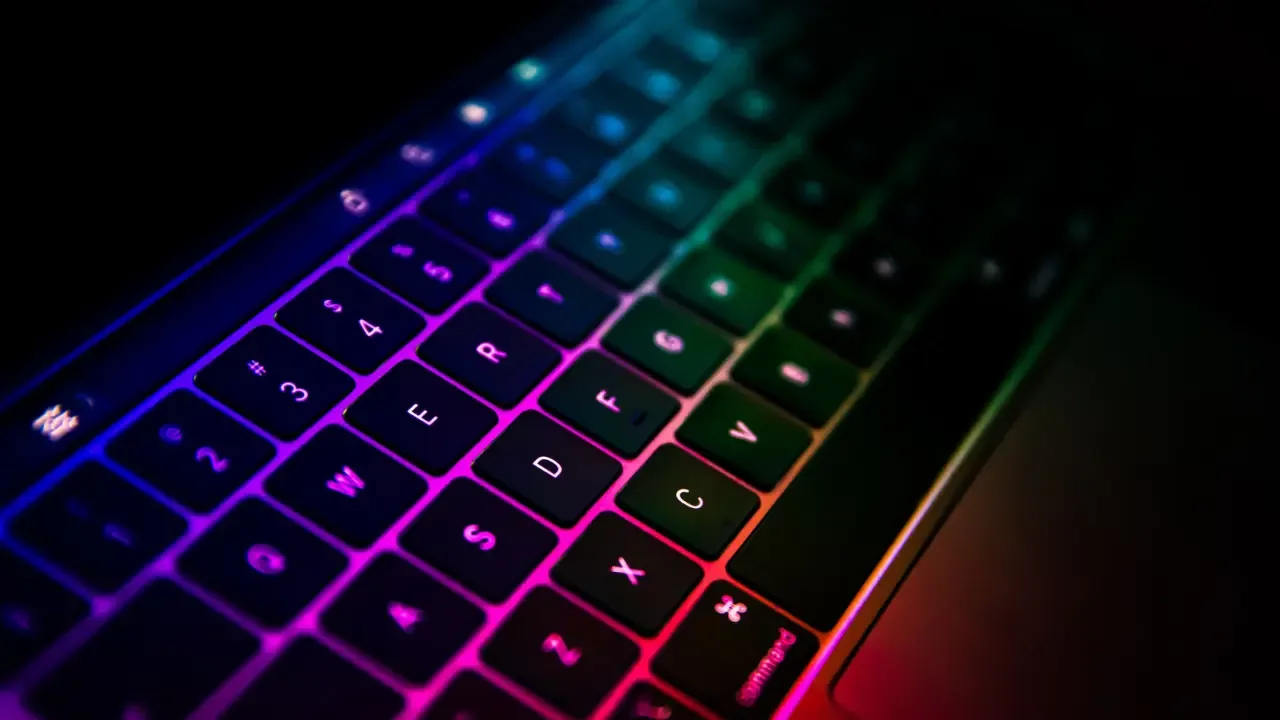
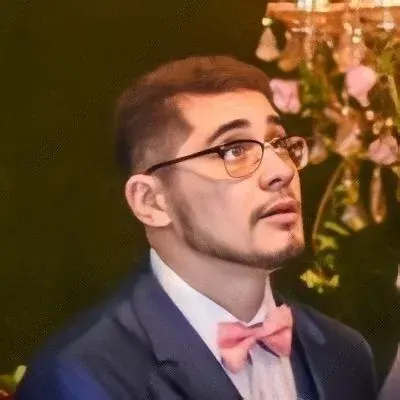
Creating a Memory Leak in Java: A Beginner's Guide 👨💻📝
So, you just had an interview and you were asked to create a memory leak in Java? Don't worry! It's not as complicated as it sounds. In this blog post, we'll break it down for you, addressing common issues and providing simple solutions.
But first, let's address the obvious question: What is a memory leak? In simple terms, a memory leak occurs when memory that is no longer needed by a program is not released, causing the program to consume more memory than necessary. This can lead to performance issues and, in extreme cases, even program crashes.
Now, let's dive into an example of how you can create a memory leak in Java:
import java.util.ArrayList;
import java.util.List;
public class MemoryLeakExample {
private static List<Object> leakList = new ArrayList<>();
public static void main(String[] args) {
while (true) {
createObjects();
deleteObjects();
}
}
private static void createObjects() {
Object object = new Object();
leakList.add(object);
}
private static void deleteObjects() {
if (!leakList.isEmpty()) {
leakList.remove(0);
}
}
}
In this example, we have a simple Java program that continuously creates and deletes objects. However, there's a catch! The leakList
object is declared as a static variable, which means it is retained in memory even when it is no longer needed. Over time, this will result in an ever-growing list of objects, consuming more and more memory until the program crashes.
Now, let's discuss a solution to this memory leak issue. In Java, you can avoid memory leaks by ensuring that objects are properly released when they are no longer needed. A common approach is to use the finalize()
method to explicitly release resources before an object is garbage collected:
import java.util.ArrayList;
import java.util.List;
public class MemoryLeakSolution {
private static List<Object> leakList = new ArrayList<>();
public static void main(String[] args) {
while (true) {
createObjects();
deleteObjects();
}
}
private static void createObjects() {
Object object = new Object();
leakList.add(object);
}
private static void deleteObjects() {
if (!leakList.isEmpty()) {
Object objectToRemove = leakList.get(0);
leakList.remove(0);
objectToRemove.finalize();
}
}
}
In this modified example, we explicitly call the finalize()
method on the object that we want to remove from the list. This ensures that any resources associated with the object are properly released, preventing a memory leak.
Now that you know how to create and solve memory leaks in Java, it's important to remember that memory management is a critical aspect of programming. Always make sure to release resources when they are no longer needed to avoid unnecessary memory consumption.
💡 Call-to-Action: Share Your Experience!
Have you ever encountered a memory leak issue in your Java projects? How did you solve it? We'd love to hear your stories and insights! Share your experiences in the comments below and let's start a discussion. 🎉🗣️
Remember, don't fear the memory leak! With the right knowledge and tools, you can conquer any programming challenge. Happy coding! 💻🚀