Iterate through a HashMap
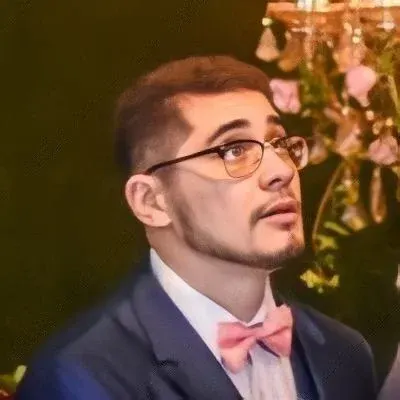
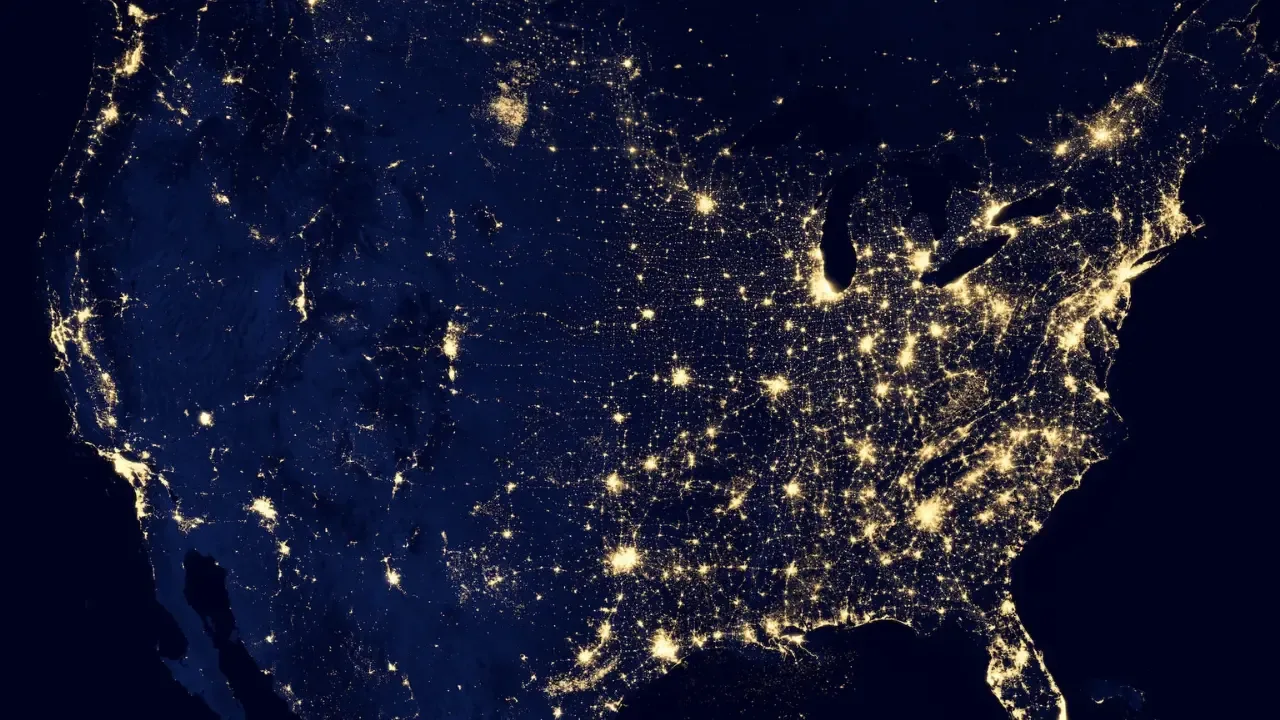
š Mastering HashMap Iteration in Java
š Hey there, tech enthusiasts! Today, we're diving into the world of HashMap iteration in Java. If you've ever found yourself scratching your head, wondering how to efficiently loop through the items in a HashMap, you've come to the right place. šÆ
The Challenge š¾
HashMaps are powerful data structures in Java, allowing you to store key-value pairs. However, when it comes to iterating through a HashMap, you might face a common challenge: how do you loop through both keys and values simultaneously and perform actions on them?
The Easy Solutions š”
Luckily, Java provides a couple of easy-to-understand solutions to conquer this challenge. Let's explore them one by one:
Solution 1: Using the entrySet() method
One way to iterate through a HashMap is by using the entrySet()
method. This method returns a Set
of Map.Entry
objects, which represent the key-value pairs in the HashMap. Here's an example:
HashMap<String, Integer> myHashMap = new HashMap<>();
// Populate our HashMap with key-value pairs...
for (Map.Entry<String, Integer> entry : myHashMap.entrySet()) {
String key = entry.getKey();
Integer value = entry.getValue();
// Perform actions on key and value...
}
In this example, we use a for-each loop to iterate over the entrySet()
. We retrieve the key and value using getKey()
and getValue()
respectively, and then perform any desired actions.
Solution 2: Using keySet() and get() methods
Another approach is to use the keySet()
and get()
methods. The keySet()
method returns a Set
of all the keys in the HashMap. We can use these keys to retrieve their corresponding values using the get()
method. Here's an example:
HashMap<String, Integer> myHashMap = new HashMap<>();
// Populate our HashMap with key-value pairs...
for (String key : myHashMap.keySet()) {
Integer value = myHashMap.get(key);
// Perform actions on key and value...
}
In this example, we iterate over the keys in the HashMap using a for-each loop. We then use get(key)
to retrieve the corresponding value and perform any desired actions.
š Time to Take Action!
Now that you're armed with the knowledge of HashMap iteration, it's time to put it into practice. Whether you're building a robust backend system or tinkering with personal projects, iterating through HashMaps is a fundamental skill that'll come in handy. šŖš»
Share this post with your fellow developers and drop a comment below with your thoughts. Let's rock the coding world together! šš„
Happy coding! š
Markdown Reference:
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
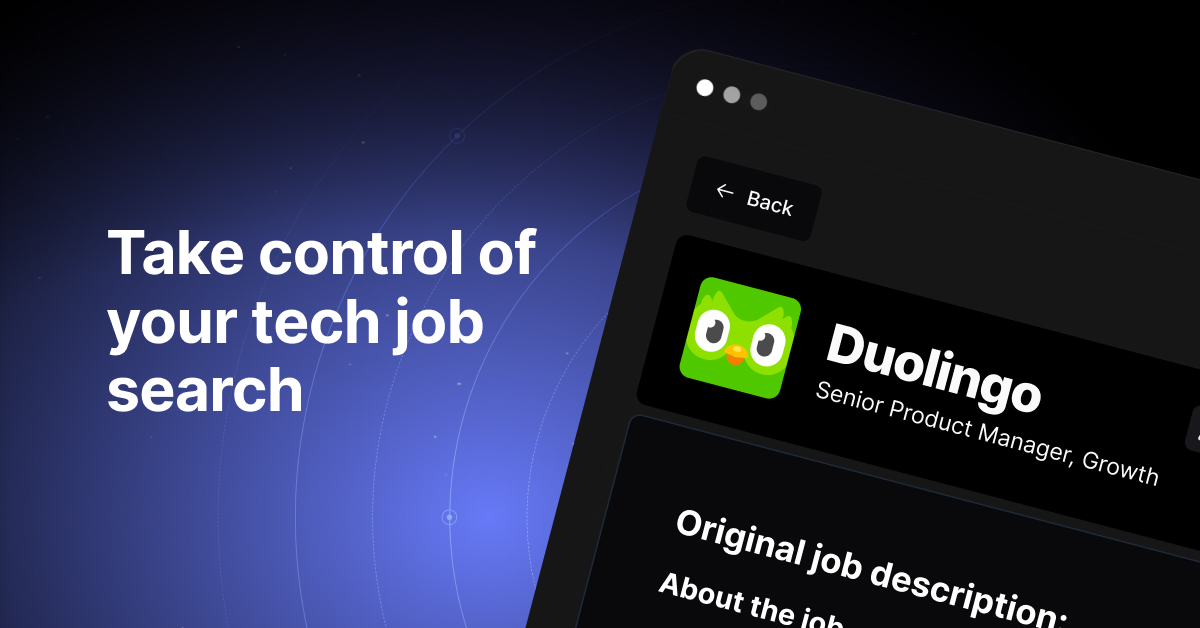