How do I convert a String to an int in Java?
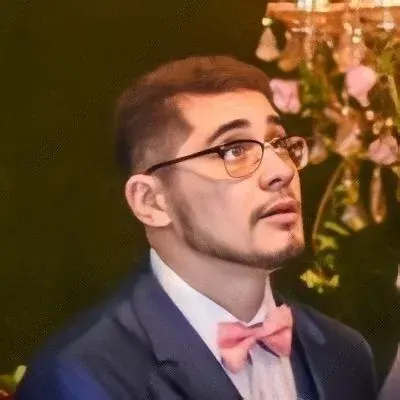
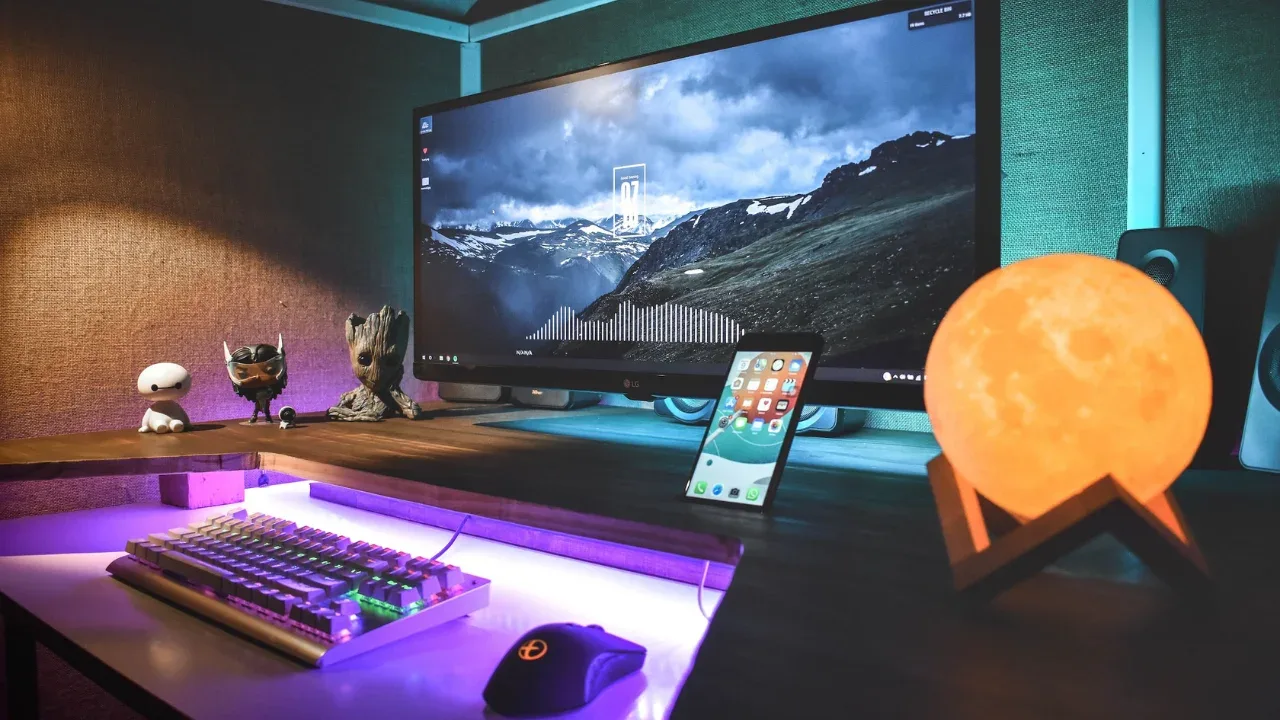
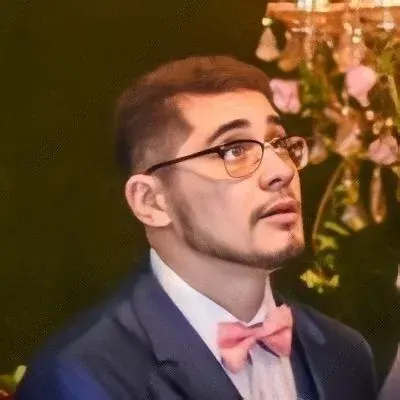
How to Convert a String to an int in Java: A Complete Guide
So, you want to convert a String
to an int
in Java, huh? Well, don't worry, because I've got you covered! 😎
The Problem
Let's say you have a String
containing a numeric value, like "1234". But you want to use this value in some mathematical operation. Uh-oh! You can't perform math on a String
! 🤔
The Solution
Luckily, Java provides a straightforward way to convert a String
to an int
. You just need to use the Integer.parseInt()
method. 🙌
Here's how you can do it:
String numberString = "1234";
int number = Integer.parseInt(numberString);
Now, the number
variable stores the integer value 1234
, which you can freely use in your code.
Common Issues
Issue #1: Invalid Input
Sometimes, the input String
may not represent a valid integer. For example, if you try to convert the String
"hello" to an int
, it won't work and will throw a NumberFormatException
. 😞
To handle this, you can wrap the conversion code in a try-catch
block to catch the exception and provide a fallback behavior. For example:
String numberString = "hello";
try {
int number = Integer.parseInt(numberString);
} catch (NumberFormatException e) {
// Handle invalid input gracefully
System.out.println("Invalid input! Please enter a valid number.");
}
Issue #2: Overflow
Another potential issue to be aware of is that the int
type in Java has a limited range of values it can represent - from -2,147,483,648 to 2,147,483,647. If the String
you're trying to convert to an int
exceeds this range, it will result in an overflow, and the conversion will not be accurate.
To handle this, you can either use a larger data type like long
or perform additional checks on the value before conversion.
Take it a Step Further
Now that you know how to convert a String
to an int
, why not challenge yourself with some additional exercises?
Write a method that takes a
String
as input and returns the sum of its digits as anint
. For example, if the input is "123", the method should return 6.Implement a program that reads a series of numbers from the user as
String
s until they enter "quit", and then calculates and displays the maximum value among those numbers.
Get creative and have fun with these exercises! Feel free to share your solutions in the comments below. Let's learn together! 😄
That's it for now! I hope this guide helped you understand how to convert a String
to an int
in Java. If you have any questions or face any issues, don't hesitate to reach out. Happy coding! 💻🚀