What is a serialVersionUID and why should I use it?
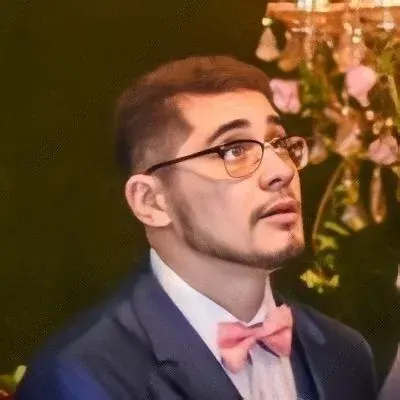
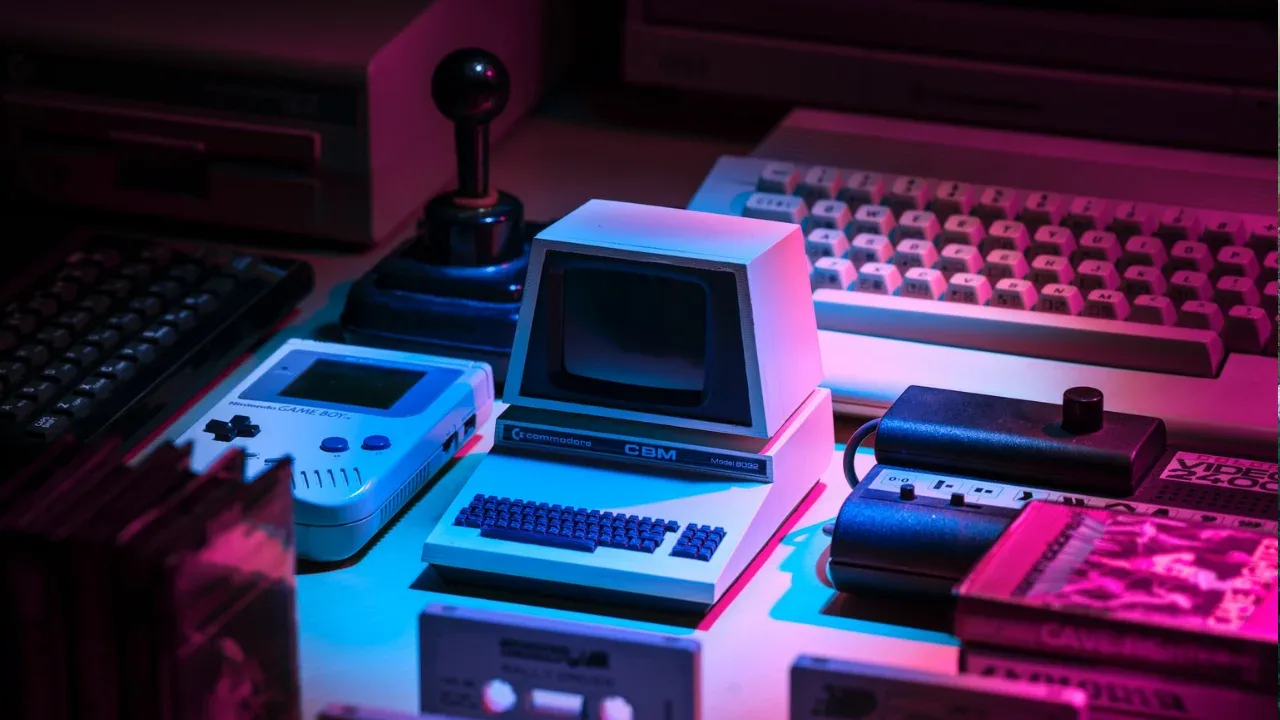
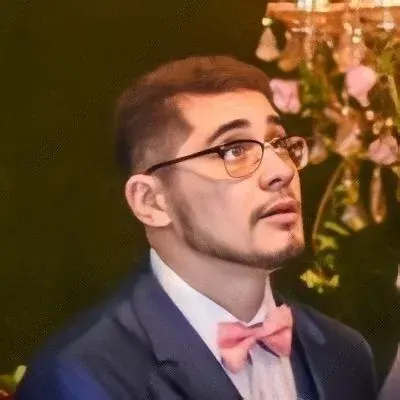
What is a serialVersionUID and why should I use it? 🤔
Have you ever encountered the warning message in Eclipse that says:
The serializable class [class name] does not declare a static final serialVersionUID field of type long.
And you're probably wondering what on earth is serialVersionUID
and why is it important? 🤷♀️
Understanding serialVersionUID 📚
In Java, serialization is the process of converting an object into a byte stream, which can then be stored, transmitted, or reconstructed later. The serialVersionUID
is a unique identifier for each version of a serializable class. It helps in ensuring that the serialized object can be properly deserialized.
When a class is serialized, the JVM includes a version number, which is derived from the class's structure, in the serialized output. This version number is used during deserialization to verify that the serialized object is compatible with the current version of the class.
Why is it important? ⚠️
The serialVersionUID
plays a crucial role in maintaining compatibility between different versions of a class. It helps in preventing compatibility issues when serialized objects are stored or exchanged between different systems.
If the serialVersionUID
is not explicitly declared in a serializable class, the serialVersionUID
is automatically generated based on the class's structure. However, this can lead to compatibility issues if the class structure changes between different versions.
When a serialized object is deserialized, the JVM compares the serialVersionUID
of the serialized object with the serialVersionUID
of the current version of the class. If they don't match, an InvalidClassException
is thrown, indicating that the serialized object is incompatible with the current version of the class.
Example: What can go wrong without serialVersionUID ❌
Let's say you have a class Foo
that you serialized and stored in a file. Later, you made some changes to the class, such as adding or removing fields or methods, without explicitly defining the serialVersionUID
. When you try to deserialize the object stored in the file, you may encounter a MismatchedInputException
or InvalidClassException
.
To illustrate this, consider the following scenario:
import java.io.*;
public class Foo implements Serializable {
private String name;
public static void main(String[] args) {
Foo foo = new Foo();
foo.name = "John";
try (ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("foo.dat"))) {
out.writeObject(foo);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Suppose you serialize an object of Foo
and store it in a file called foo.dat
. Now, let's make a change to the Foo
class by adding a new field:
import java.io.*;
public class Foo implements Serializable {
private String name;
private int age; // Added field
public static void main(String[] args) {
try (ObjectInputStream in = new ObjectInputStream(new FileInputStream("foo.dat"))) {
Foo foo = (Foo) in.readObject();
System.out.println(foo.name + " " + foo.age);
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
When you try to deserialize the object from foo.dat
, you will encounter an InvalidClassException
:
java.io.InvalidClassException: Foo; local class incompatible: stream classdesc serialVersionUID = 7595033062022934907, local class serialVersionUID = -1006817923481521808
This exception occurs because the serialVersionUID
of the deserialized object (stored in foo.dat
) doesn't match the serialVersionUID
of the current version of the Foo
class. As a result, deserialization fails, and you won't be able to retrieve the object.
The Solution: Using serialVersionUID 🛠️
To prevent the compatibility issues described above, you should explicitly declare the serialVersionUID
in your serializable classes. By providing a static final serialVersionUID
field, you can control the versioning of your class.
Here's an updated version of the Foo
class, explicitly defining the serialVersionUID
:
import java.io.*;
public class Foo implements Serializable {
private static final long serialVersionUID = 123456789L;
private String name;
private int age; // Added field
// Rest of the code...
}
By defining the serialVersionUID
field, you ensure that the serialVersionUID
remains constant even if you make changes to the class structure. As a result, the deserialization process will be able to match the serialVersionUID
of the serialized object with the serialVersionUID
of the current version of the class, enabling a successful deserialization.
The Rule of thumb 📝
Remember to follow this rule of thumb when dealing with serialVersionUID
:
Explicitly declare
serialVersionUID
in your serializable classes.Do not modify
serialVersionUID
once it is declared, unless you intentionally want to break compatibility with previously serialized objects.
By following this simple guideline, you'll save yourself from compatibility headaches and ensure seamless object serialization and deserialization.
If you found this article helpful, don't forget to share it with your fellow developers! 👩💻👨💻
Now, go ahead and make your code serialization-friendly! 😄