How do I test a class that has private methods, fields or inner classes?
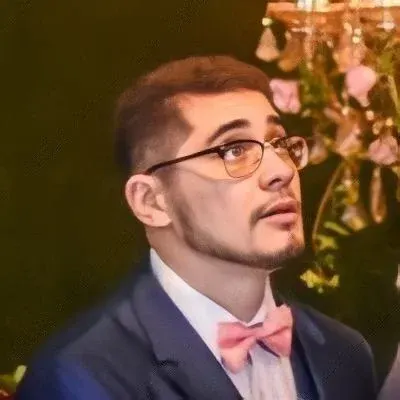
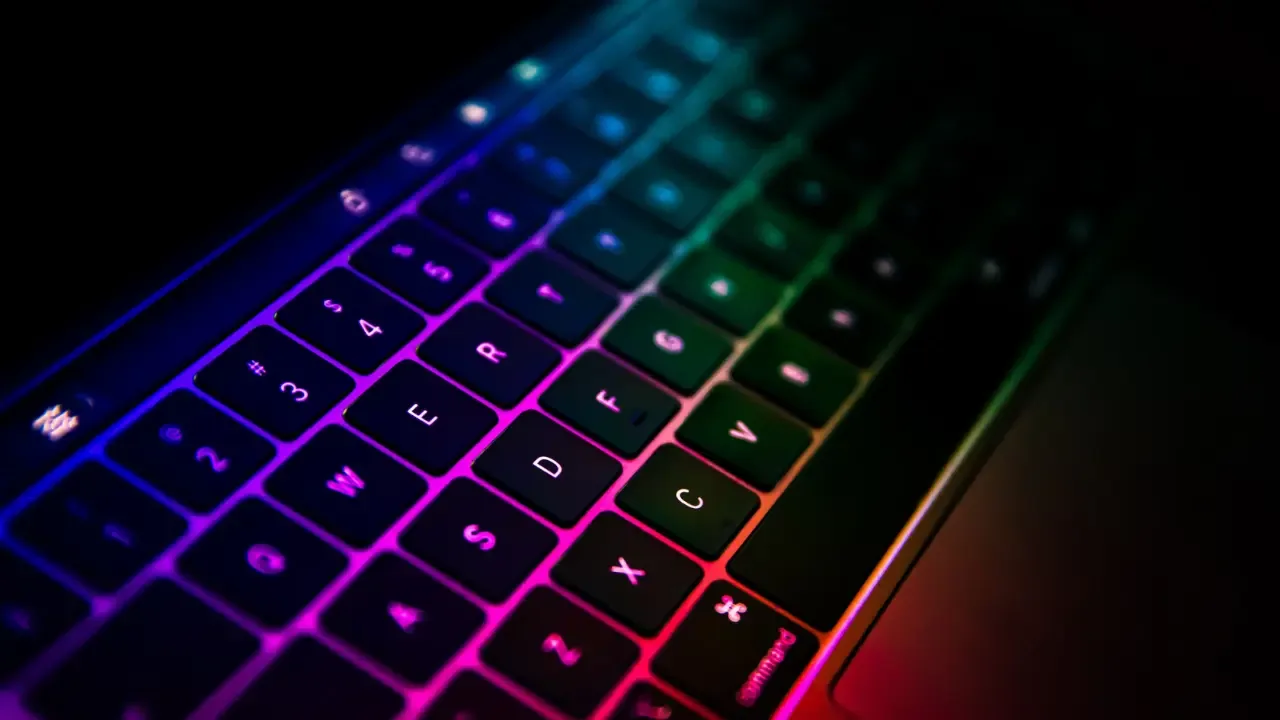
Testing Classes with Private Methods, Fields, or Inner Classes
So, you have this awesome class you've been working on, and it has some private methods, fields, or even nested classes. Now, you're faced with the challenge of testing it using JUnit. However, you don't want to compromise the integrity of your code just for the sake of testing. We totally get it, and today, we'll show you some easy solutions to this common issue.
The Problem: Private Methods, Fields, and Inner Classes
It's often considered bad practice to change the access modifier of a method, field, or inner class just to test it. Doing so can potentially weaken the encapsulation of your code and introduce unnecessary risks. Fortunately, there are better ways to go about testing such private components.
Solution 1: Reflection
One way to access private methods, fields, or inner classes is through reflection. Java's java.lang.reflect
package provides a mechanism to inspect and modify field and method access, including private members. Let's dive into an example to illustrate how this works:
public class MyClass {
private void privateMethod() {
// some implementation
}
}
To test the privateMethod()
, we can use reflection:
public class MyClassTest {
@Test
public void testPrivateMethod() throws NoSuchMethodException, IllegalAccessException, InvocationTargetException {
MyClass myClass = new MyClass();
Method privateMethod = MyClass.class.getDeclaredMethod("privateMethod");
privateMethod.setAccessible(true); // allow access to private method
privateMethod.invoke(myClass);
// assertions
}
}
By using getDeclaredMethod()
and setAccessible(true)
, we can invoke the private method and test its behavior.
While this solution works, it's worth mentioning that reflection can have performance implications and might not work well in all scenarios. It's important to gauge whether the benefits outweigh the drawbacks in your specific use case.
Solution 2: Extract Private Functionality into Separate Classes
Another approach to handling the testing of private methods and inner classes is to extract that functionality into separate classes, making them public and testable independently. Let's look at an example:
public class MyClass {
private HelperClass helper;
public MyClass() {
this.helper = new HelperClass();
}
// other public methods
private class HelperClass {
// private methods and fields
public void publicMethod() {
// some implementation
}
}
}
In the above example, we've extracted the inner class HelperClass
into a separate public class. Now, we can test it independently:
public class HelperClassTest {
@Test
public void testPublicMethod() {
HelperClass helper = new HelperClass();
// test publicMethod
}
}
By extracting the private functionality into public classes, you're able to test them directly without any need to fumble around with accessing private members.
Call-to-Action and Reader Engagement
Testing private methods, fields, or inner classes doesn't have to be a daunting task. By using either the reflection approach or extracting the functionality into separate public classes, you can confidently test your code without compromising its integrity.
Which approach do you find most appealing for testing private components? Let us know in the comments below! And don't forget to share this post with your fellow developers who might be facing the same challenge. Happy testing! ππ¬
(Disclaimer: Itβs important to note that modifying private members for testing purposes should be done judiciously, as it can impact the maintainability and reliability of your code. Use these solutions wisely and with consideration.)
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
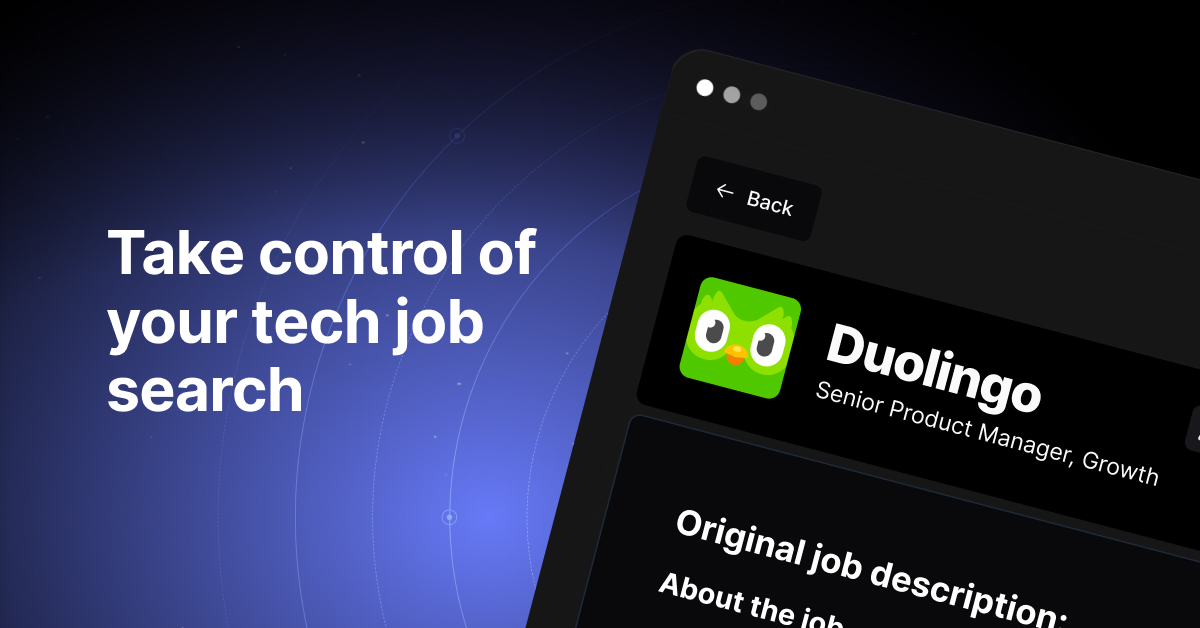