Getting attribute using XPath
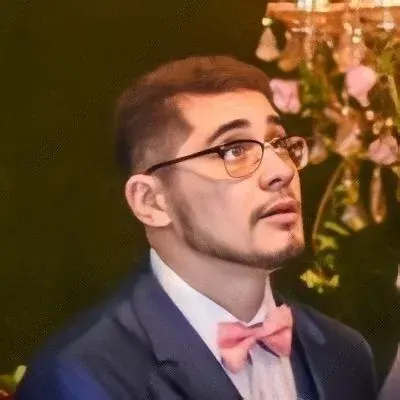
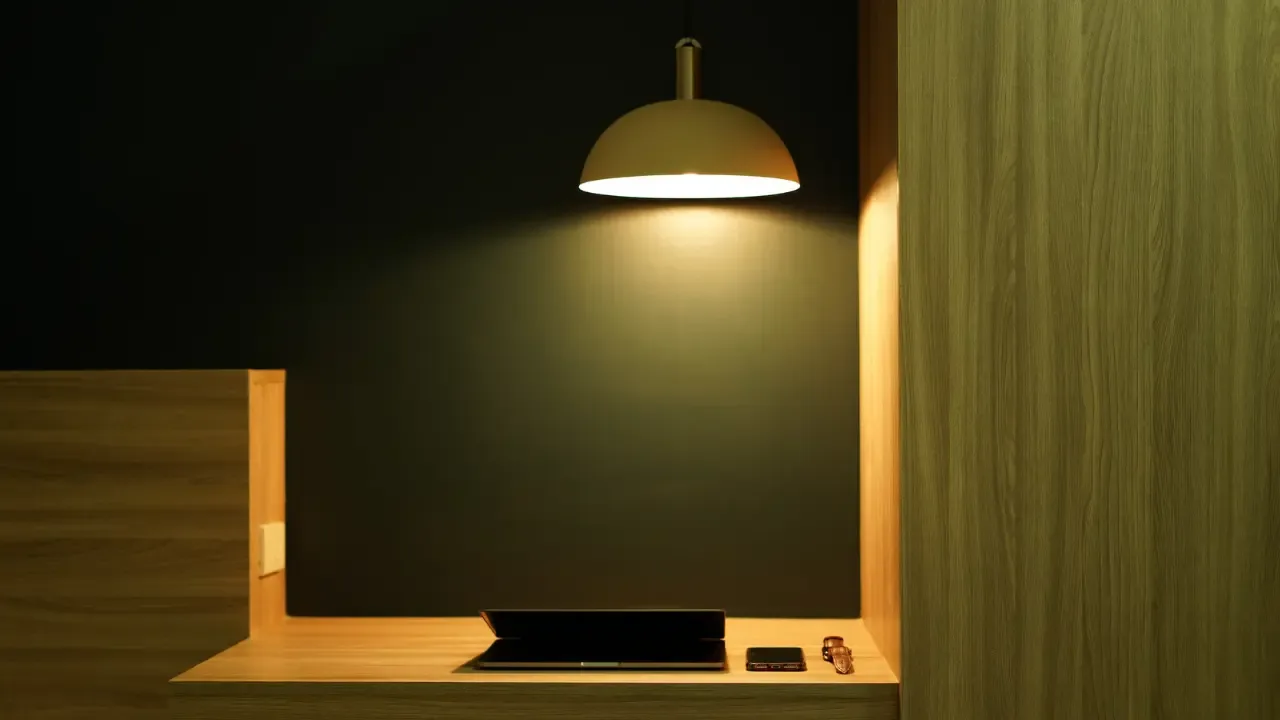
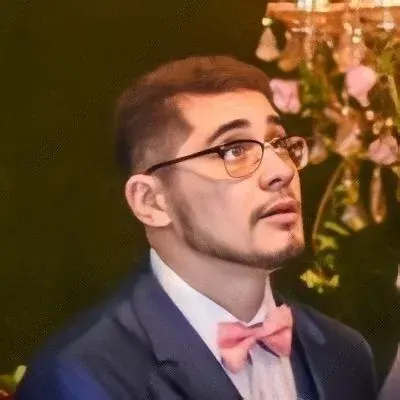
How to Get an Attribute Using XPath in XML π
Have you ever wondered how to extract specific information from an XML structure? Well, XPath is here to save the day! π¦ΈββοΈ
Today, we will tackle a common problem: getting the value of an attribute using XPath. To illustrate this, let's take a look at the following XML structure:
<?xml version="1.0" encoding="ISO-8859-1"?>
<bookstore>
<book>
<title lang="eng">Harry Potter</title>
<price>29.99</price>
</book>
<book>
<title lang="eng">Learning XML</title>
<price>39.95</price>
</book>
</bookstore>
Now, our task is to extract the value of the lang
attribute for the first <book>
element, where the language is set to "eng". Let's dive right into the solution!
Using XPath to Get the Attribute Value π―
XPath provides a concise and powerful way to navigate XML structures. To solve our problem, we can use the following XPath expression:
/bookstore/book[1]/title/@lang
In this expression, we are using the following steps:
/bookstore
selects the root element./book[1]
selects the first<book>
element./title
selects the<title>
element./@lang
selects the value of thelang
attribute.
Combining these steps, our XPath expression will precisely locate the attribute we need: eng
.
How to Use XPath in Your Code π»
To implement XPath in your code, you'll need an XPath evaluator library. Most programming languages offer XPath modules or libraries that can help you with this task. Here's an example using Python:
import xml.etree.ElementTree as ET
# Load the XML data
tree = ET.parse('your_xml_file.xml')
root = tree.getroot()
# Use XPath to get the attribute value
lang = root.find("./book[1]/title").attrib['lang']
# Print the result
print(f"The value of lang is: {lang}")
In this example, we use Python's built-in xml.etree.ElementTree
module. After loading the XML data, we can use the find()
method along with our XPath expression to access the desired attribute value. Finally, we print the result: The value of lang is: eng
.
π Share Your Experience!
XPath can be a real lifesaver when it comes to extracting information from XML. Now that you've learned how to get an attribute using XPath, why not try it out in your own code? Share your experiences, success stories, or even challenges you've faced along the way in the comments section below! Let's bring our XML knowledge together! π€
Happy coding! π»β¨