Safe (bounds-checked) array lookup in Swift, through optional bindings?
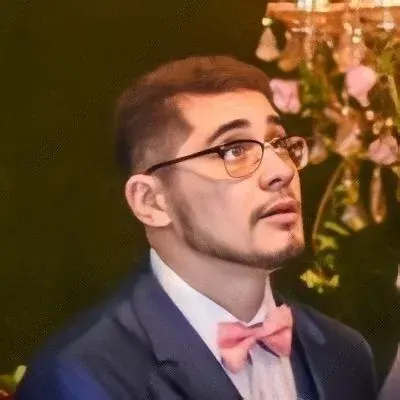
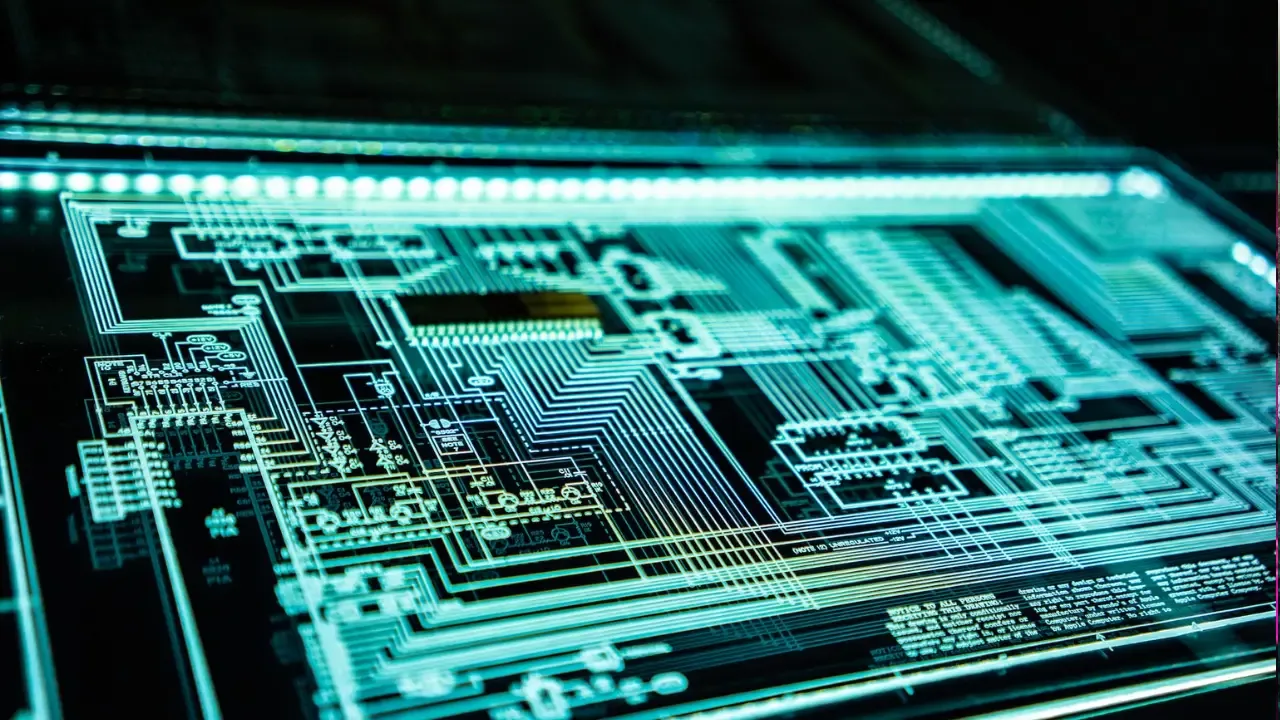
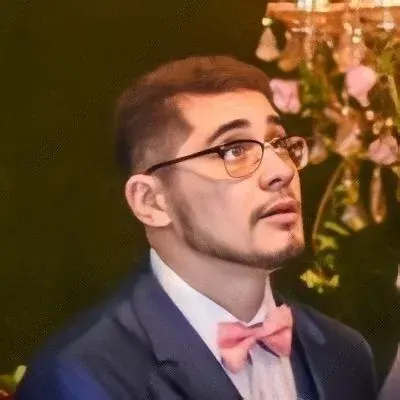
š Safe (bounds-checked) Array Lookup in Swift, through Optional Bindings
š¤ Have you ever encountered a runtime error while accessing an index that is out of bounds in Swift arrays? It can be frustrating, especially considering Swift's focus on safety and optional chaining. But fear not! In this blog post, we'll explore easy solutions to safely access array elements and prevent runtime errors.
š When you try to access an index that is out of bounds in an array, you may have seen this error message: EXC_BAD_INSTRUCTION
. Let's take a look at an example:
var str = ["Apple", "Banana", "Coconut"]
str[0] // "Apple"
str[3] // EXC_BAD_INSTRUCTION
š± As you can see, trying to access an index that doesn't exist leads to a runtime error. But wouldn't it be great if we could use optional chaining and safely check if an index is valid before accessing it? Let's explore this idea together!
š¤ Instead of relying on the "ol'" if statement to check if the index is less than str.count
, we can leverage optional bindings to handle this scenario beautifully. Let's take a look at the improved code:
let theIndex = 3
if let nonexistent = str[theIndex] { // Bounds check + Lookup
print(nonexistent)
// ...do other things with nonexistent...
}
š Ta-da! By using optional bindings, we can ensure that the index is valid before accessing it. If the index is out of bounds, the condition evaluates to false
, and the code within the if statement block doesn't execute.
⨠But wait, there's more! We can even go a step further and enhance the built-in subscript behavior of an array by creating an extension. Let's see how:
extension Array {
subscript(var index: Int) -> AnyObject? {
if index >= self.count {
NSLog("Womp!")
return nil
}
return self[index]
}
}
š In this extension, we override the subscript operator of the Array type and provide our implementation. We check if the index is out of bounds, and if so, we log a helpful message and return nil. Otherwise, we simply pass the call to the original implementation of subscript to retrieve the element at the specified index.
š¤ Now you might be wondering how to access the items (index-based) without using subscript notation within the return self[index]
line. Well, fear not! The subscript notation is the most concise and idiomatic way to access array elements, so we're already on the right track.
š To sum it up, by using optional bindings, we can safely perform bounds checking before accessing array elements in Swift. Additionally, with a little extension magic, we can enhance the subscript behavior and handle out-of-bounds indexes gracefully.
š So go ahead, give these solutions a try in your Swift projects and never worry about runtime errors due to out-of-bounds array access again! Share your thoughts and experiences in the comments below, and let's make Swift development safer and more enjoyable together! šš