WPF: Setting the Width (and Height) as a Percentage Value
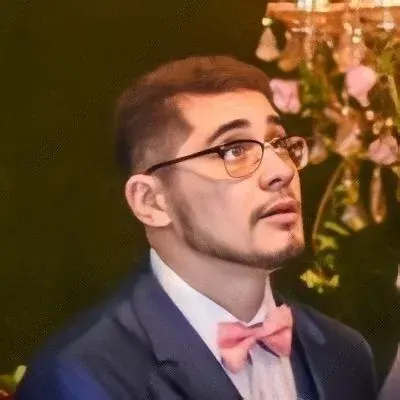
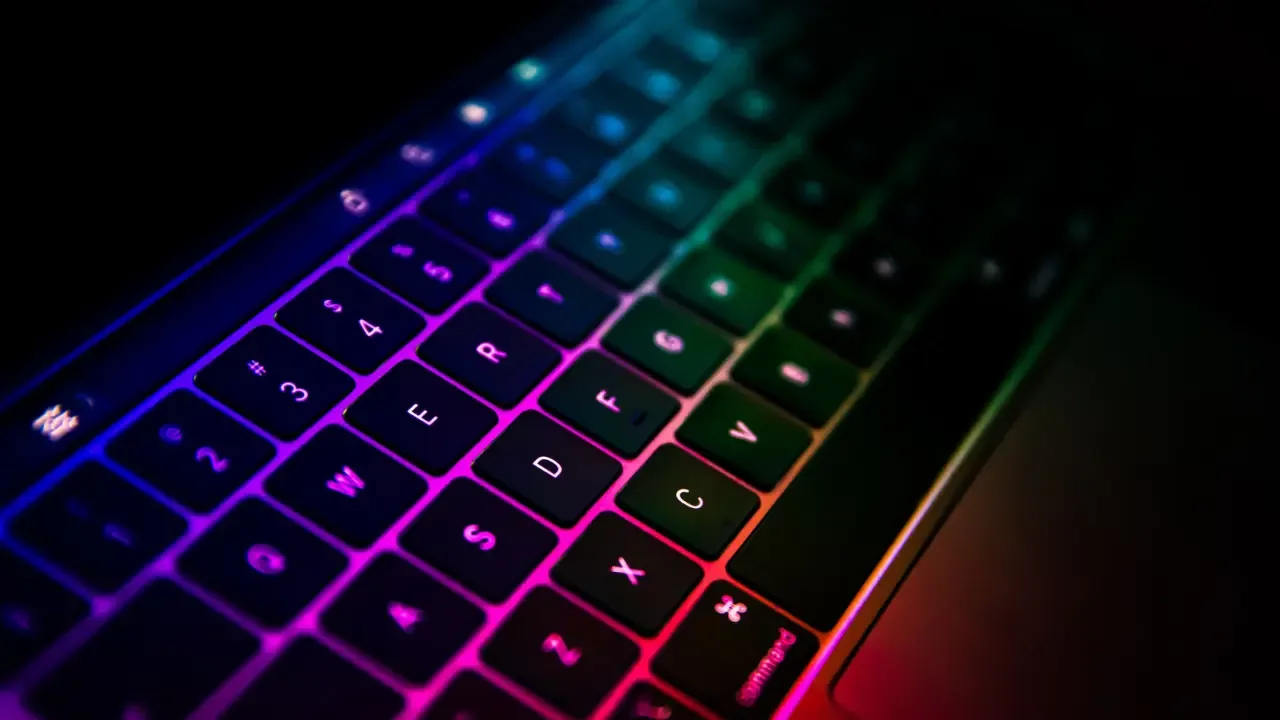
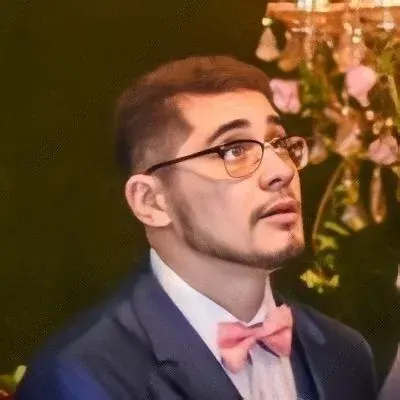
šš©āš»āØ Title: "WPF Got You Stuck? Here's How You Can Set Width (and Height) as a Percentage Value!"
Introduction: š Hey there, fellow tech enthusiasts! Have you ever found yourself scratching your head over how to set the width (and height) of elements in WPF using percentage values? š¤ Well, fret not! In this blog post, we'll dive into this exact conundrum and provide easy solutions that will make your life as a WPF developer a breeze. So, let's get started, shall we?
Problem: Matching Parent Container's Width (or Percentage) with Dynamic Expansion š¤·āāļø You want a TextBlock (or any other element) to stretch from side to side, either matching its parent container's width or taking up a percentage of it. You also want the child elements to automatically expand if the parent container's width is increased, just like how it's done in HTML and CSS. But how do you accomplish this in XAML without specifying absolute values?
Solution: Utilizing the Power of Grid! ā”ļø Luckily, WPF provides a powerful layout control called Grid that allows us to achieve this effortlessly. Here's how you can do it:
Set up the Grid layout:
<Grid> <!-- Add your parent container elements here --> <TextBlock HorizontalAlignment="Stretch" VerticalAlignment="Center" Text="Hello, WPF!" /> </Grid>
Configure the column widths:
<Grid> <Grid.ColumnDefinitions> <ColumnDefinition Width="*" /> <!-- This sets the column width to the remaining space --> </Grid.ColumnDefinitions> <!-- Add your parent container elements here --> <TextBlock Grid.Column="0" HorizontalAlignment="Stretch" VerticalAlignment="Center" Text="Hello, WPF!" /> </Grid>
Expand the parent container:
Method 1: Through Code-Behind
// Inside your code-behind file private void ExpandParentContainer() { // Assuming you have a variable named "parentGrid" representing your parent container parentGrid.ColumnDefinitions[0].Width = new GridLength(1, GridUnitType.Star); }
Method 2: Data Binding
<Grid> <Grid.ColumnDefinitions> <!-- Bind the width to a property in your data context --> <ColumnDefinition Width="{Binding ParentContainerWidth}" /> </Grid.ColumnDefinitions> <!-- Add your parent container elements here --> <TextBlock Grid.Column="0" HorizontalAlignment="Stretch" VerticalAlignment="Center" Text="Hello, WPF!" /> </Grid>
Call-to-Action: Engaging Readers & Building Community š” Woohoo! You're now well-versed in dynamically setting the width (and height) of elements as a percentage value in WPF. As you continue exploring the vast world of WPF, don't hesitate to experiment and share your findings with our tech community. We'd love to hear your thoughts, questions, or even your own tricks and tips!
š Leave a comment below āļø and let us know how your WPF journey is going! Have you encountered any other challenges? We're here to help! Help us spread the knowledge by sharing this blog post with your fellow WPF enthusiasts. Let's grow our community and support each other! šš
Conclusion: š Congratulations on mastering the art of setting width (and height) as a percentage value in WPF! Remember, with the power of Grid and a little bit of creativity, you can achieve wonders in your WPF designs. Keep exploring, experimenting, and pushing the boundaries of what's possible. Together, we'll conquer any WPF challenge that comes our way! šŖ
Happy coding! šš©āš»š„