Set value to null in WPF binding
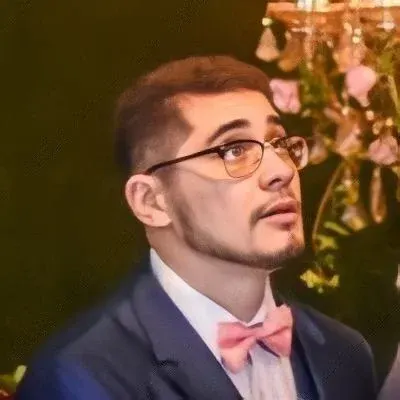
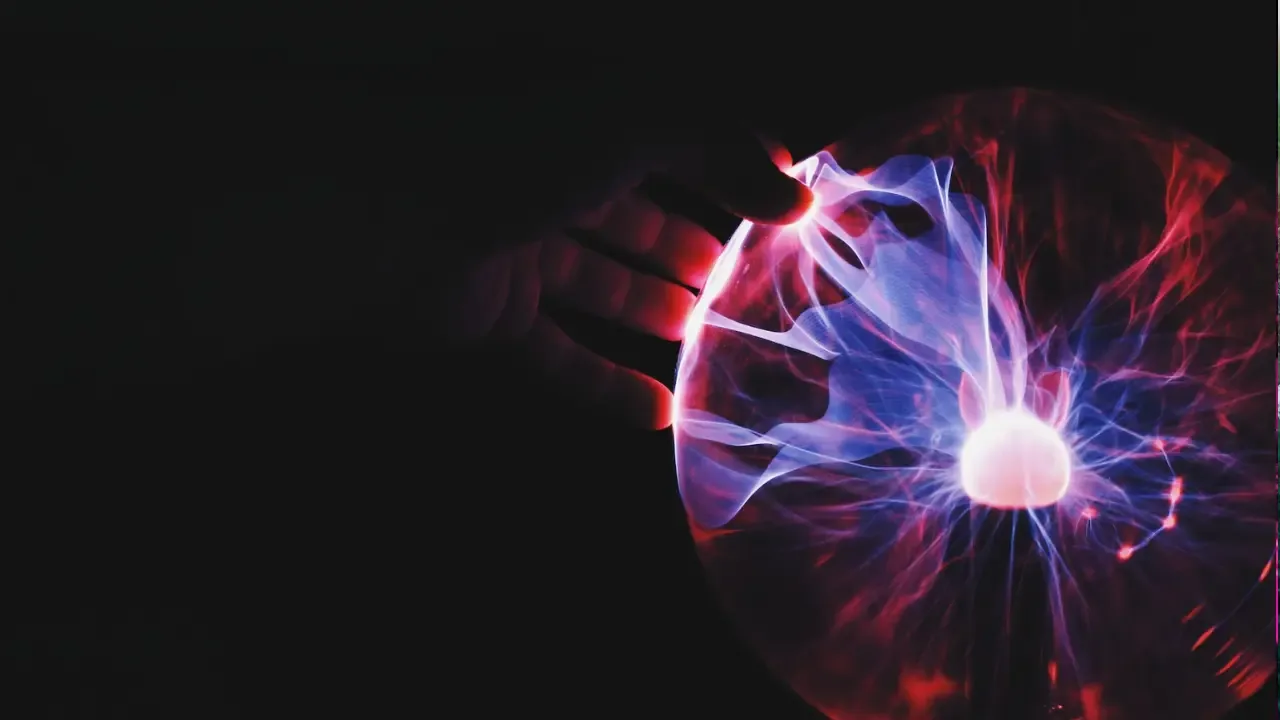
📝✨🤔 How to Set Value to Null in WPF Binding? 💭🔍
So, you want to automatically update the data source with null if the user deletes the content of a TextBox
in your WPF application? 🤔 No worries, we've got you covered! In this blog post, we'll explore how to achieve this in XAML, step-by-step! 🚀💻
✨ The Problem ✨
Let's start by understanding the problem. You have a TextBox
whose Text
property is bound to a Nullable decimal
property called "Price". However, when the user deletes the content of the TextBox
, you want the binding to update the data source with null. So, how can we accomplish this? 🤔
💡 The Solution 💡
To achieve automatic updates with null values, we can leverage the power of StringFormat
in XAML! Here's how you can do it:
1️⃣ Update your XAML code to include a StringFormat
attribute for the TextBox
:
<TextBox Text="{Binding Price, StringFormat={}{0:#.00;;null}}" />
🔍 Explanation:
The
StringFormat
is enclosed within curly braces{}
.We use
#
to specify the format for non-null values, which is#.00
to show two decimal places.We use
;
to separate the format for positive, negative, and null values.Since we want null values, we leave the null format empty
;;null
.
🎉 That's it! With this simple modification, your TextBox
binding will automatically update the data source with null when the user deletes the content!
🔍 Example Usage 🔍
Let's see this solution in action with an example:
1️⃣ XAML code snippet:
<TextBox Text="{Binding Price, StringFormat={}{0:#.00;;null}}" />
2️⃣ C# property:
private decimal? _price;
public decimal? Price
{
get { return _price; }
set { _price = value; }
}
In this example, if the user enters a value of "10.50" in the TextBox
, the Price
property will be updated with 10.50. However, if the user deletes the content or enters an empty string, the Price
property will be set to null.
🔥 Embrace the Null Value 🔥
With this easy solution, you can now gracefully handle null values in your WPF bindings! 🎉✨ Whether you're dealing with Nullable types or simply want to allow users to delete content, this approach will provide a seamless user experience.
So, go ahead and try it out in your WPF application! 💪💻 And if you have any other tricky tech questions, feel free to reach out to us! We love helping you conquer coding challenges! 😊
🌟💬✨ Let's hear from you: Have you ever encountered issues with null values in WPF bindings? How did you handle it? Share your experiences and insights in the comments below! Let's learn from each other! 🌟💬✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
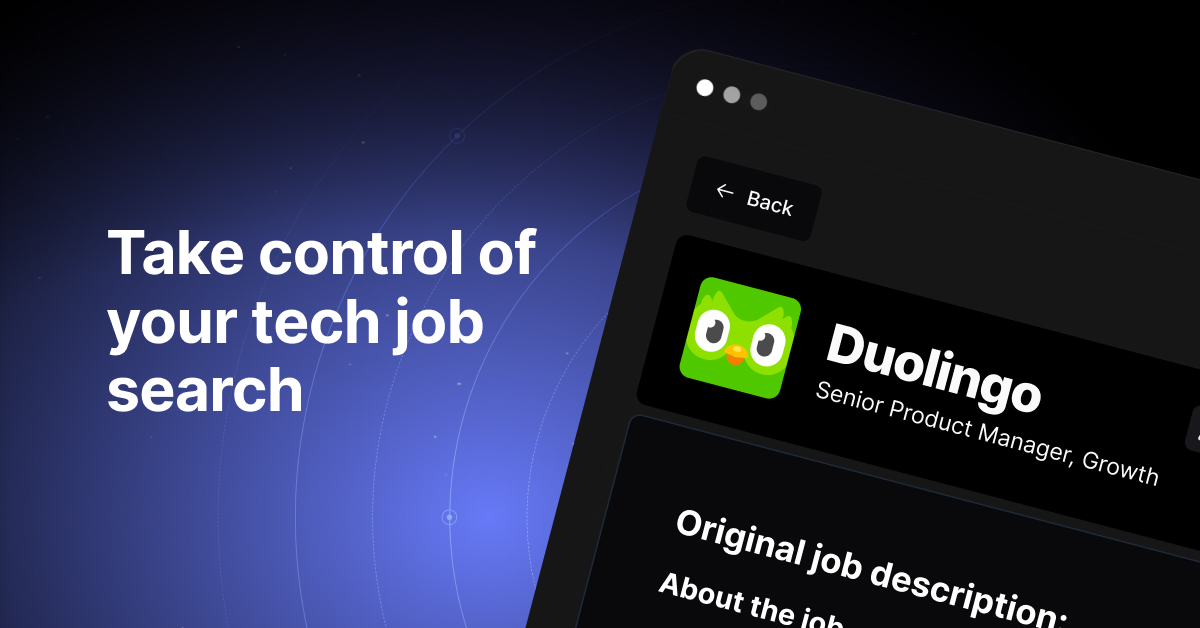