Passing two command parameters using a WPF binding
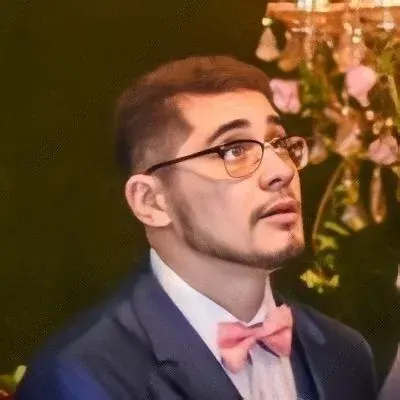
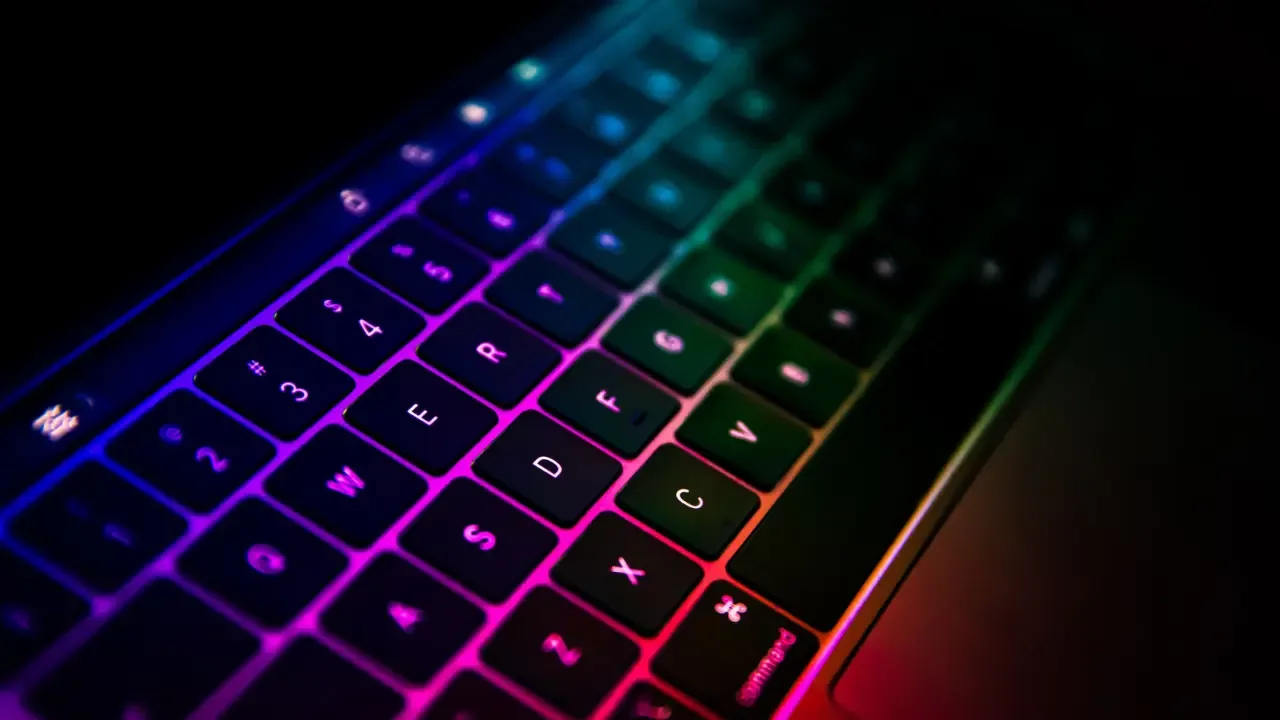
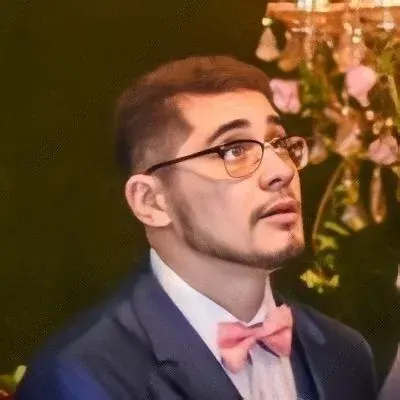
Passing Two Command Parameters using WPF Binding: A Complete Guide
š„š Hey there, tech enthusiasts! š Are you scratching your head, trying to figure out how to pass two command parameters using WPF binding? š¤ We've got you covered! In this guide, we're going to address the common issues around this challenge and provide you with easy solutions. Buckle up, and let's dive right in! šŖ
The Challenge: Need for Two Command Parameters
The scenario is straightforward. You've created a command in your ViewModel and bound it to a button in your XAML file. Everything worked fine until you realized that your Zoom operation requires two pieces of information from the view: the width and height of the canvas. š®
The Standard Approach: Single Command Parameter
In the standard approach, you would define your button with a single command parameter, like this:
<Button Content="Zoom" Command="{Binding MyViewModel.ZoomCommand}"
CommandParameter="{Binding ElementName=MyCanvas, Path=Width}" />
However, this approach falls short when you need to pass multiple command parameters. š« The CommandParameter attribute only accepts a single value, leaving you scratching your head for a solution. š¤ Fear not! We've got a workaround for you! š
The Solution: Using Attached Properties
To pass multiple command parameters using WPF binding, we're going to utilize the power of attached properties. Here's how you can do it:
Create a static class, let's call it
CommandParameters
, and define attached properties for the desired parameters:
public static class CommandParameters
{
public static readonly DependencyProperty WidthProperty =
DependencyProperty.RegisterAttached("Width", typeof(double), typeof(CommandParameters), new PropertyMetadata(default(double)));
public static readonly DependencyProperty HeightProperty =
DependencyProperty.RegisterAttached("Height", typeof(double), typeof(CommandParameters), new PropertyMetadata(default(double)));
public static void SetWidth(UIElement element, double value)
{
element.SetValue(WidthProperty, value);
}
public static double GetWidth(UIElement element)
{
return (double)element.GetValue(WidthProperty);
}
public static void SetHeight(UIElement element, double value)
{
element.SetValue(HeightProperty, value);
}
public static double GetHeight(UIElement element)
{
return (double)element.GetValue(HeightProperty);
}
}
In your XAML, bind the properties of your canvas to the attached properties:
<Canvas x:Name="MyCanvas"
local:CommandParameters.Width="{Binding Width}"
local:CommandParameters.Height="{Binding Height}">
<!-- Canvas content goes here -->
</Canvas>
Finally, update your button's binding to include both command parameters:
<Button Content="Zoom" Command="{Binding MyViewModel.ZoomCommand}"
CommandParameter="{Binding ElementName=MyCanvas,
Path=({
local:CommandParameters.Width},
{local:CommandParameters.Height}
)}" />
š” And there you have it! By utilizing attached properties, you can now pass both the width and height of your canvas as command parameters! š
The Call-to-Action: Engage with Us!
We hope this guide has solved your problem and opened new possibilities for your WPF applications! š If you found this guide useful, don't forget to share it with your friends and colleagues. And hey, we love hearing from our readers! Let us know your thoughts or any other WPF challenges you'd like us to cover in future blog posts. Drop a comment below and keep the conversation going! š¬
That's a wrap, folks! Thanks for joining us on this WPF adventure. Stay tuned for more exciting tech guides and tutorials. Until next time, happy coding! šš»