How to perform Single click checkbox selection in WPF DataGrid?
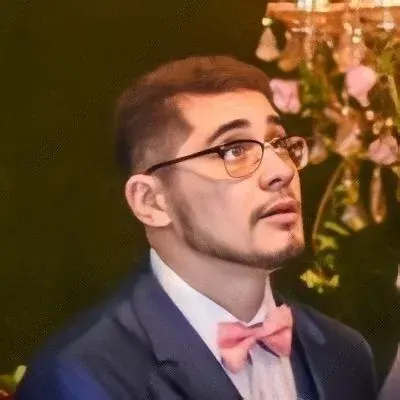
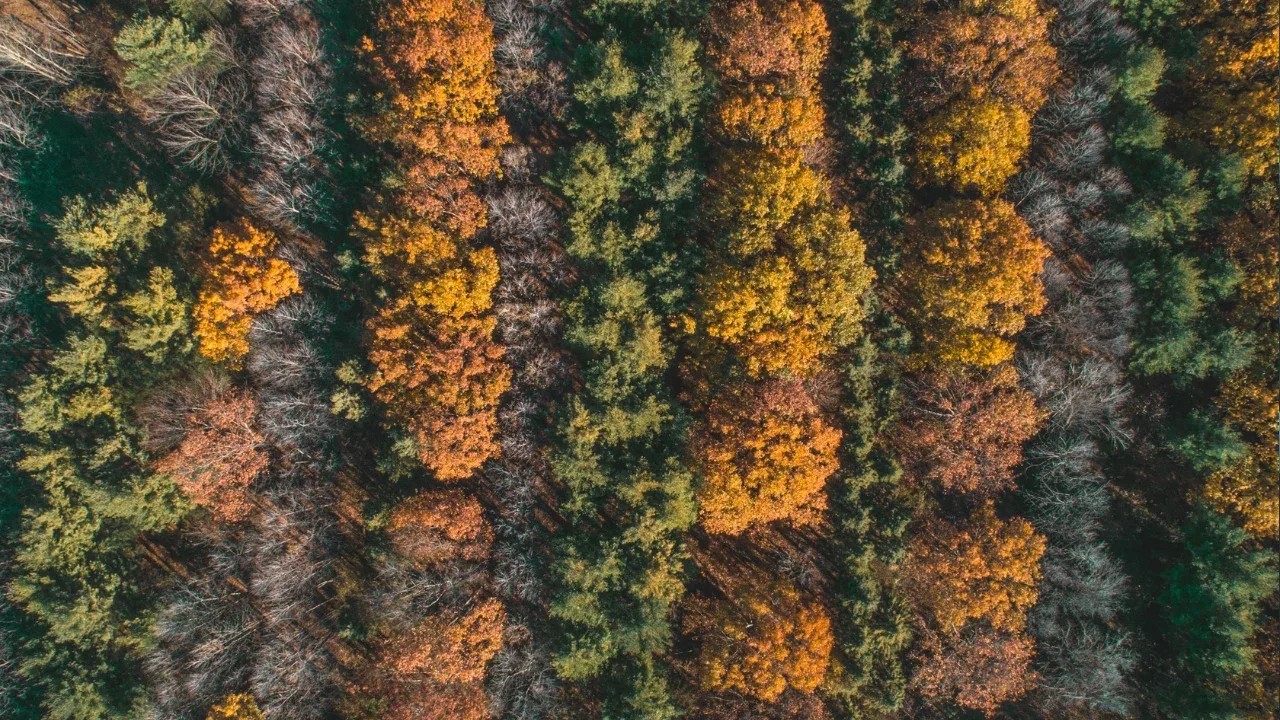
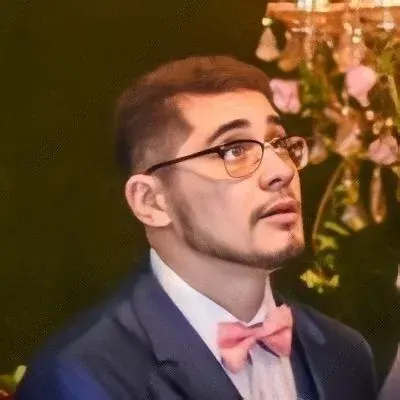
😎 Single Click Checkbox Selection in WPF DataGrid
Are you stuck with a WPF DataGrid that requires two clicks to check or uncheck a checkbox column? 😫 Don't worry, we've got you covered! In this blog post, we'll show you how to perform single-click checkbox selection in WPF DataGrid. 📝
The Problem:
The issue at hand is that the checkbox column in the DataGrid requires two clicks to get selected - the first click selects the cell, and only the second click checks the checkbox. But we want to make life easier for our users and have the checkbox get checked or unchecked with a single click. 💪
The Solution:
The solution involves handling the PreviewMouseLeftButtonDown
event of the CheckBox column and manually toggling the checked state. Here are the steps to achieve single-click checkbox selection in WPF DataGrid:
Start by adding the
PreviewMouseLeftButtonDown
event handler to the CheckBox column in the DataGrid.In the event handler, check whether the source of the event is a CheckBox. If it is, manually toggle the checked state of the CheckBox.
Finally, mark the event as handled so that the DataGrid does not perform any default behavior.
Here's a code snippet that demonstrates the solution:
private void CheckBoxColumn_PreviewMouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
var checkBox = e.OriginalSource as CheckBox;
if (checkBox != null)
{
checkBox.IsChecked = !checkBox.IsChecked;
e.Handled = true;
}
}
Don't forget to wire up this event handler to the PreviewMouseLeftButtonDown
event of the CheckBox column in your XAML code. 🧩
<DataGrid>
<DataGrid.Columns>
<DataGridTextColumn Header="First Column" Binding="{Binding PropertyName}" />
<DataGridCheckBoxColumn Header="Second Column"
PreviewMouseLeftButtonDown="CheckBoxColumn_PreviewMouseLeftButtonDown" />
</DataGrid.Columns>
</DataGrid>
Time to Take Action:
Now that you have the solution, it's time to implement it in your WPF DataGrid! 🚀 Don't let your users struggle with double-clicking checkboxes anymore. Implementing single-click checkbox selection will greatly enhance the user experience of your application. Give it a try and see the difference! 💯
If you have any questions or face any issues, feel free to leave a comment below. We're here to help you out! 💁♂️
Don't Be Selfish, Share the Knowledge:
If you found this blog post helpful, share it with your fellow WPF developers! Let's spread the word and help each other overcome common hurdles. You can also follow our blog and social media channels for more exciting tutorials and tips on WPF development. 🌟
Happy coding! 👩💻👨💻