How to bind multiple values to a single WPF TextBlock?
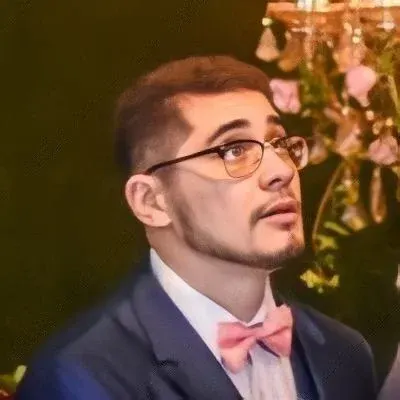

How to Bind Multiple Values to a Single WPF TextBlock? 🧩
So, you want to bind multiple values to a single WPF TextBlock? 🤔 No worries, my friend! I've got you covered! 😎
The Problem 🚫
Currently, you have a TextBlock that is successfully binding the value of a property called "Name". Here's an example of what you have:
<TextBlock Text="{Binding Name}" />
However, now you also want to bind another property called "ID" to the same TextBlock, and you're wondering if it's possible to achieve this by simple concatenation, like Name + ID
. Let's find out! 🕵️♀️
The Solution 💡
Unfortunately, you can't directly bind two or more values to a TextBlock using simple concatenation. But don't worry! There are a couple of alternative approaches you can take to achieve the desired result. Let's explore them! 🚀
1. Using a Converter 🔄
One option is to use a Value Converter. A Value Converter allows you to transform the bound values before they are displayed in the TextBlock. Here's how you can do it:
Create a new class that implements the
IValueConverter
interface. Let's call itMultiValueConverter
.
public class MultiValueConverter : IValueConverter
{
public object Convert(object[] values, Type targetType, object parameter, CultureInfo culture)
{
// Combine the values in any way you want
string combinedValue = string.Join(" ", values);
return combinedValue;
}
public object[] ConvertBack(object value, Type[] targetTypes, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
Add an instance of the
MultiValueConverter
to your XAML resources.
<Window.Resources>
<local:MultiValueConverter x:Key="MultiValueConverter" />
</Window.Resources>
Update your TextBlock markup to use the converter and bind to the desired properties.
<TextBlock>
<TextBlock.Text>
<MultiBinding Converter="{StaticResource MultiValueConverter}">
<Binding Path="Name" />
<Binding Path="ID" />
<!-- Add more bindings if needed -->
</MultiBinding>
</TextBlock.Text>
</TextBlock>
With this approach, the Convert
method in your MultiValueConverter
will receive the values of the bound properties (Name
and ID
in this case), and you can combine them using any logic you desire. The resulting text will be displayed in the TextBlock. Voila! 🎉
2. Using a ViewModel ✨
Another approach is to create a ViewModel that exposes a new property that combines both the Name
and ID
properties. Here's how it can be done:
Create a new class that represents your ViewModel. Let's call it
MyViewModel
.
public class MyViewModel : INotifyPropertyChanged
{
private string _name;
private string _id;
public string Name
{
get { return _name; }
set
{
_name = value;
OnPropertyChanged();
OnPropertyChanged(nameof(CombinedValue));
}
}
public string ID
{
get { return _id; }
set
{
_id = value;
OnPropertyChanged();
OnPropertyChanged(nameof(CombinedValue));
}
}
public string CombinedValue => $"{Name} {ID}";
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged([CallerMemberName] string propertyName = null)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
Set an instance of this ViewModel as the DataContext of your XAML.
<Window.DataContext>
<local:MyViewModel />
</Window.DataContext>
Update your TextBlock to bind to the CombinedValue property in your ViewModel.
<TextBlock Text="{Binding CombinedValue}" />
Now, whenever the Name
or ID
properties change, the CombinedValue property will automatically update, and the TextBlock will display the new combined value. Pretty nifty, right? 😉👌
Your Turn! 🙌
Try out these solutions and see which one works best for your specific scenario. Remember, depending on your requirements, one approach may be more suitable than the other.
I hope this guide helped you in binding multiple values to a single WPF TextBlock! If you have any more questions or need further assistance, don't hesitate to reach out in the comments section below. Happy coding! 🎉💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
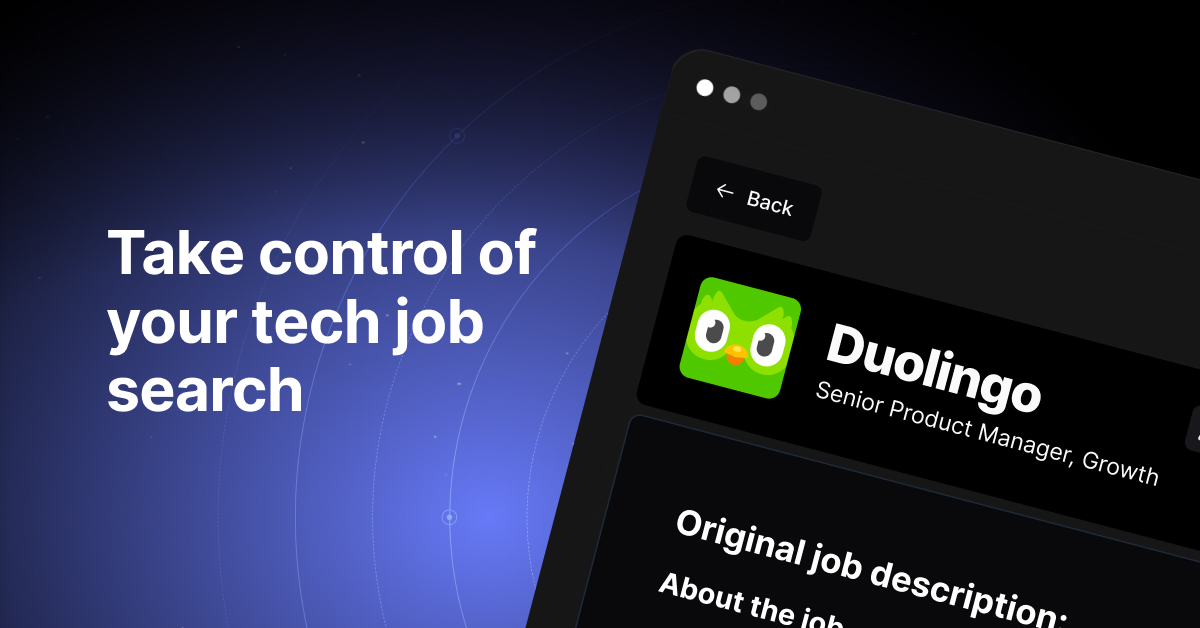