How to bind inverse boolean properties in WPF?
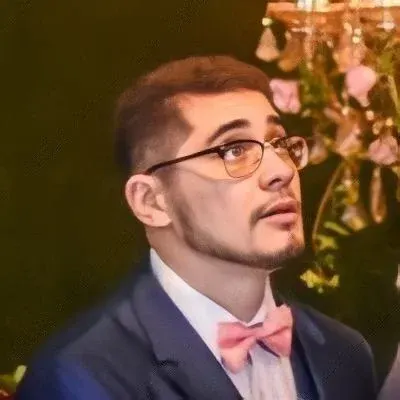
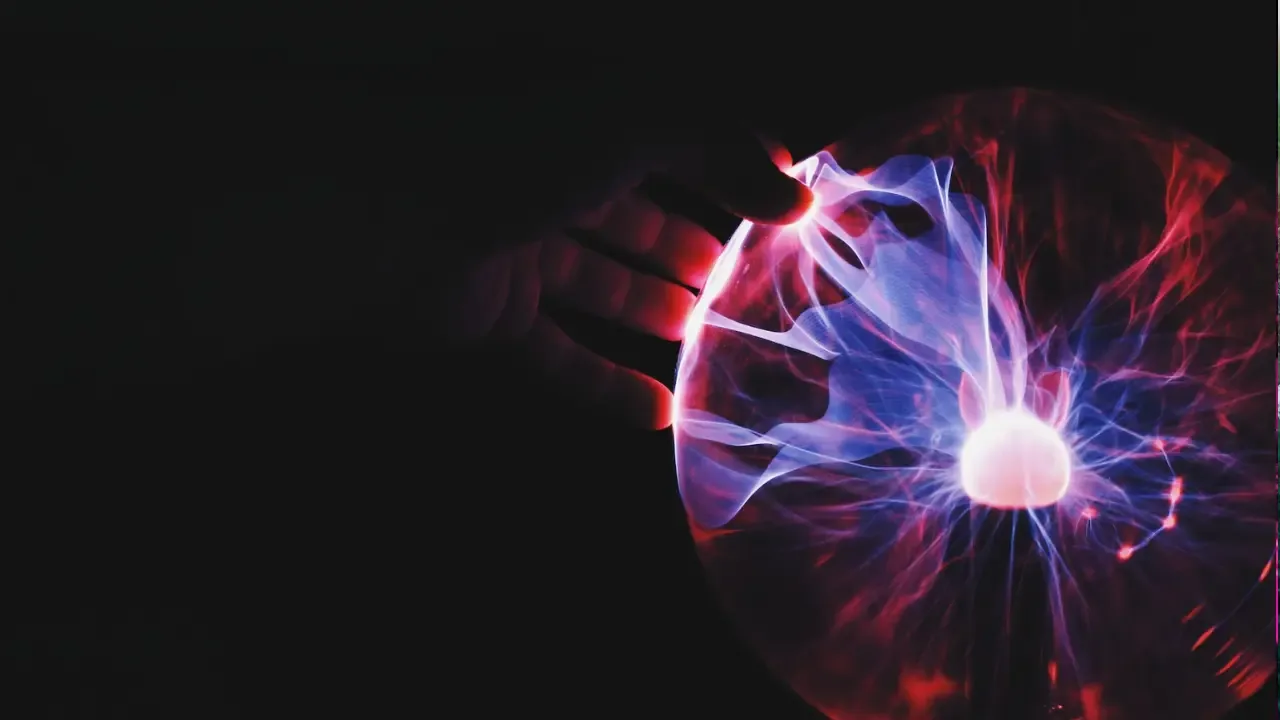
📚 How to Bind Inverse Boolean Properties in WPF? 🤔💡
Have you ever had the need to bind an inverse boolean property in WPF? Maybe you have an object with an IsReadOnly
property and you want to set the IsEnabled
property on a Button to false
when IsReadOnly
is true
. Sounds simple, right? But it's not as straightforward as you might initially think.
🤷♀️ The Struggle is Real
You might be thinking that you can easily achieve this by using the following binding: IsEnabled="{Binding Path=!IsReadOnly}"
. However, this doesn't work in WPF. So, what can we do?
⚙️ The Solution
To bind an inverse boolean property in WPF, we need to define a style with triggers. In this case, we'll define a style for the Button and use DataTriggers to set the IsEnabled
property based on the value of the IsReadOnly
property.
Here's an example of how you can achieve this:
<Button.Style>
<Style TargetType="{x:Type Button}">
<Style.Triggers>
<DataTrigger Binding="{Binding Path=IsReadOnly}" Value="True">
<Setter Property="IsEnabled" Value="False" />
</DataTrigger>
<DataTrigger Binding="{Binding Path=IsReadOnly}" Value="False">
<Setter Property="IsEnabled" Value="True" />
</DataTrigger>
</Style.Triggers>
</Style>
</Button.Style>
By using this approach, we can dynamically update the IsEnabled
property based on changes to the IsReadOnly
property. When IsReadOnly
is true
, the Button will be disabled, and when IsReadOnly
is false
, the Button will be enabled.
🥳 The Result
With just a few lines of code, we can effortlessly bind inverse boolean properties in WPF and achieve the desired behavior. 🎉
🔍🤝 Take it Further
Now that you know how to bind inverse boolean properties in WPF, experiment with this technique in your own projects. Enhance your user interfaces by dynamically enabling or disabling controls based on certain conditions. Share your experiences and let us know how you've used this technique to improve your applications! We would love to hear from you. 💬💡
Keep coding, keep experimenting, and keep building awesome things! Happy coding! 😄👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
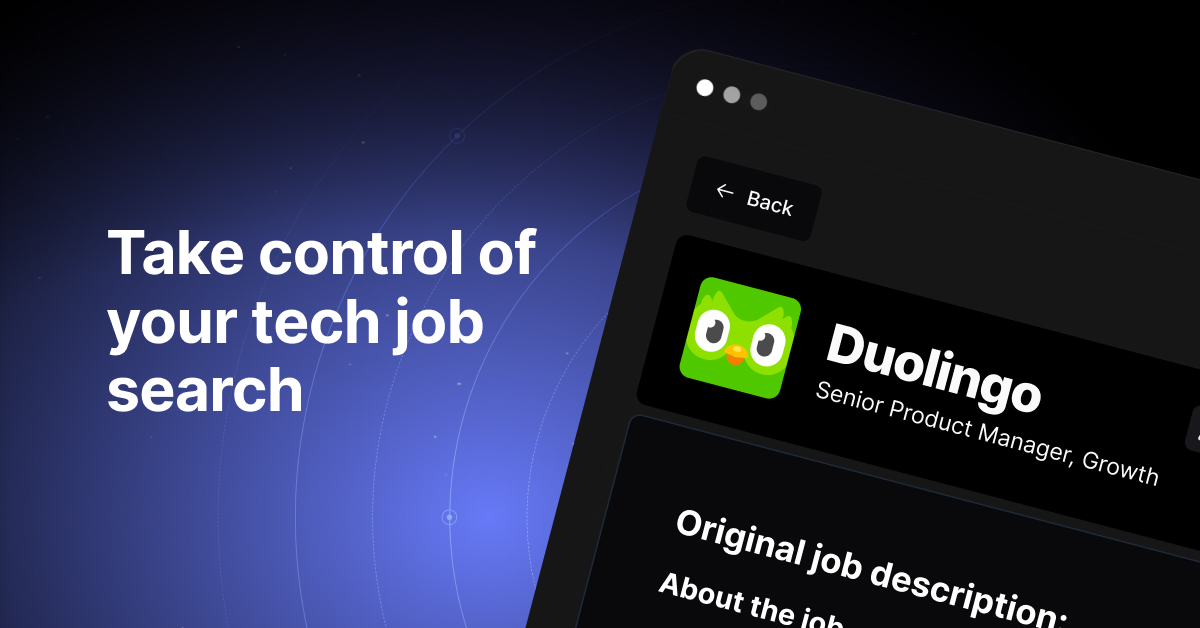