Creating SolidColorBrush from hex color value
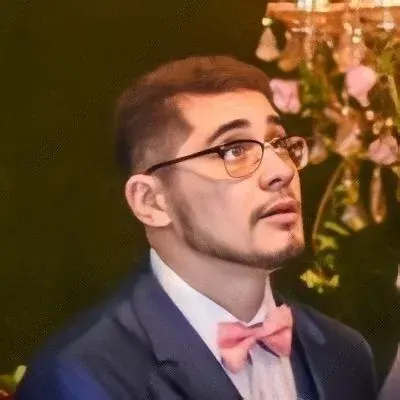
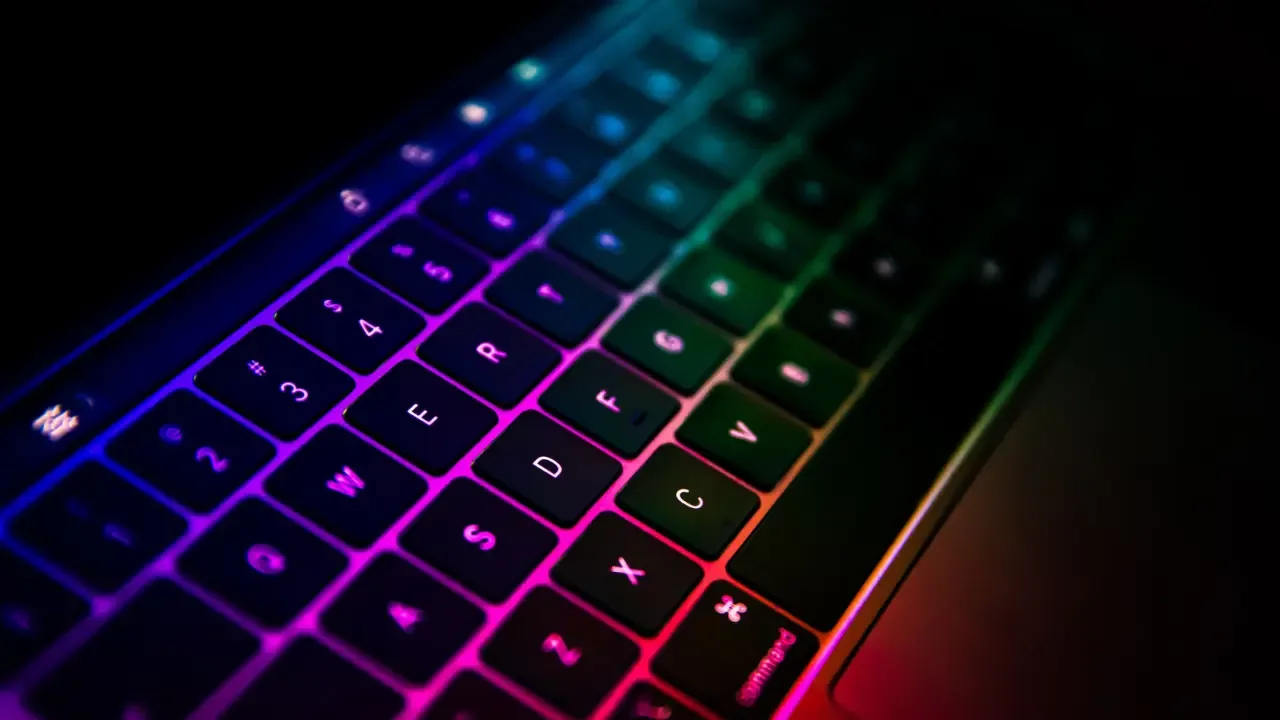
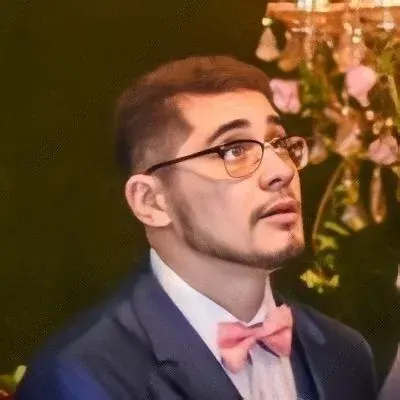
Creating SolidColorBrush from Hex Color Value: A Simple Guide 😎🎨
So you want to create a SolidColorBrush
from a Hex color value like #ffaacc
? 🎨 No worries, we've got you covered! 😄
The MSDN Solution: Color.FromArgb() 🌟
If you've searched on MSDN, you might have come across the following example:
SolidColorBrush mySolidColorBrush = new SolidColorBrush();
mySolidColorBrush.Color = Color.FromArgb(255, 0, 0, 255);
The Color.FromArgb()
method creates a Color
object with the specified Alpha, Red, Green, and Blue values. ✨
The Issue: Using Hex Values Instead 🙃
Now, you wanted to pass a color value like #ffaacc
instead of individual RGB values. So, you wrote the following code:
Color.FromRgb(
Convert.ToInt32(color.Substring(1, 2), 16),
Convert.ToInt32(color.Substring(3, 2), 16),
Convert.ToInt32(color.Substring(5, 2), 16)
);
But it gave you a compilation error saying "The best overloaded method match for 'System.Windows.Media.Color.FromRgb(byte, byte, byte)' has some invalid arguments." 😢
You also encountered three "Cannot convert int to byte" errors. 🤔
The Solution: Converting Hex to RGB 👍
To convert the Hex color code to RGB, you need to follow these steps:
Remove the '#' character from the beginning of the Hex value.
Split the remaining string into three parts: Red (2 characters), Green (2 characters), and Blue (2 characters).
Convert each part from Hexadecimal to Decimal.
Use the converted RGB values to create the
Color
object.
To implement this solution, you can use the following code snippet: 🔢
string hexColor = color.Substring(1); // Remove '#'
byte red = Convert.ToByte(hexColor.Substring(0, 2), 16);
byte green = Convert.ToByte(hexColor.Substring(2, 2), 16);
byte blue = Convert.ToByte(hexColor.Substring(4, 2), 16);
SolidColorBrush mySolidColorBrush = new SolidColorBrush(Color.FromRgb(red, green, blue));
Now you can create a valid SolidColorBrush
using the Hex color value you provided! 🎉🌈
Engage with Our Community! 💬💡
We hope this guide helped you understand how to create a SolidColorBrush
from a Hex color value. If you have any questions, feel free to drop a comment below or reach out to our friendly community. We love helping fellow tech enthusiasts! 💪✨
Don't forget to share this blog post with your friends who might find it useful. Sharing is caring! 😉📢
Happy coding! 💻🎉