WordPress get_template_part pass variable
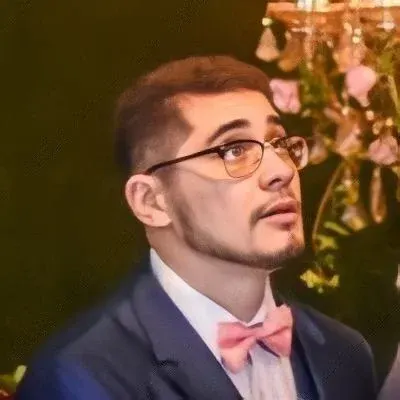
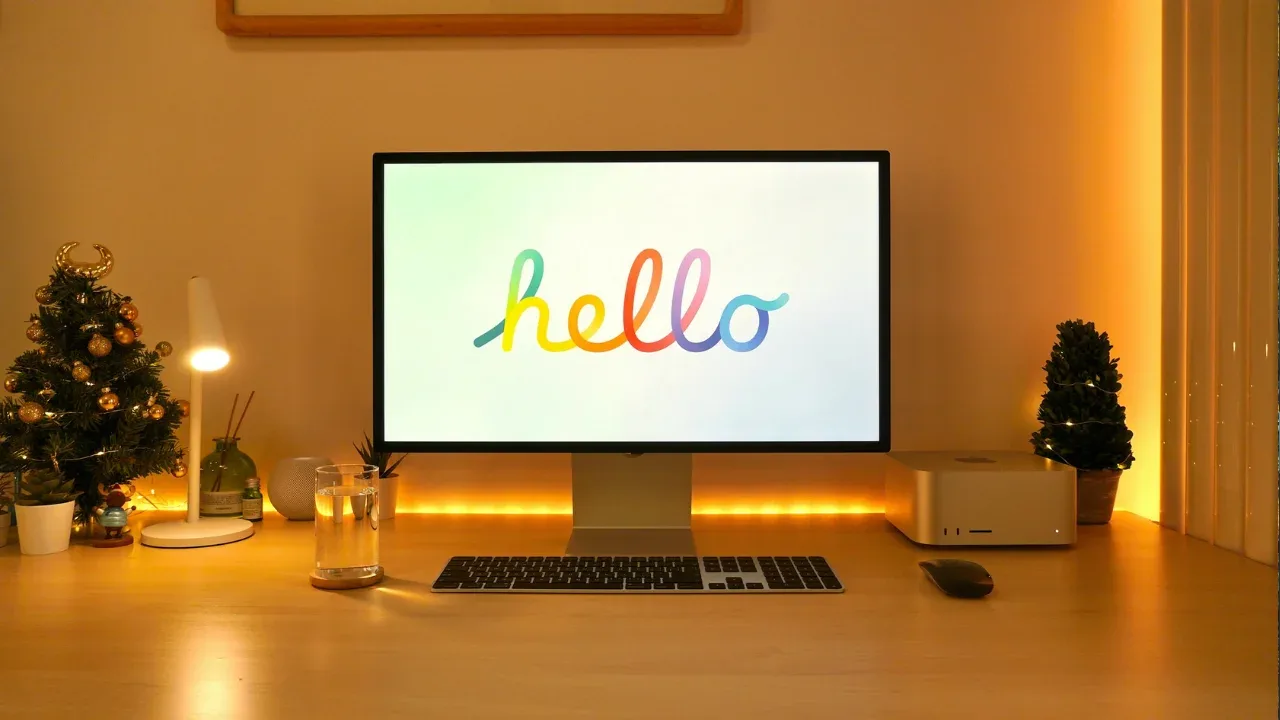
🌟 Passing Variables to get_template_part()
in WordPress 🌟
If you're a WordPress developer, you may have come across the situation where you need to pass a variable to the get_template_part()
function. This function is commonly used to include template files in your WordPress theme. However, by default, it doesn't provide a built-in way to pass variables to these template files.
But don't worry, I'm here to help you find easy solutions to this common issue! In this blog post, we'll explore a few techniques to pass variables to get_template_part()
, so you can make your template files more dynamic and flexible. Let's dive in! 💪
The Scenario
To give you a better understanding, let's consider the following code snippet:
<?php get_template_part('element-templates/front', 'top'); ?>
<?php get_template_part('element-templates/front', 'main'); ?>
In this example, we have two calls to get_template_part()
. The file being included is front-top.php
for the first call and front-main.php
for the second call. Our goal is to pass different numeric variables to each of these template files.
Solution 1: Using Global Variables
One straightforward way to pass variables to template files is by using global variables. WordPress has a predefined global variable called $GLOBALS
that allows you to store and access variables across your PHP code.
To pass a variable, you can set it as a global variable before calling get_template_part()
, like this:
<?php
$GLOBALS['my_custom_variable'] = 123;
get_template_part('element-templates/front', 'top');
?>
Then, in your front-top.php
file, you can access the variable as follows:
<?php
$custom_variable_value = isset($GLOBALS['my_custom_variable']) ? $GLOBALS['my_custom_variable'] : '';
echo "My custom variable is: " . $custom_variable_value;
?>
By using global variables, you now have the ability to pass and access variables across different template files.
Solution 2: Using extract()
Another handy technique to pass variables to get_template_part()
is by utilizing the extract()
function. This function allows you to extract variables from an associative array into the current symbol table.
Here's how you can use extract()
to achieve our goal:
<?php
$my_custom_variable = 123;
$variables_array = array(
'custom_variable' => $my_custom_variable
);
get_template_part('element-templates/front', 'top', $variables_array);
?>
In your front-top.php
file, you can extract the variables from the array using extract()
:
<?php extract($args); ?>
<p>My custom variable is: <?php echo $custom_variable; ?></p>
With this approach, you can pass multiple variables using a single array and access them easily within your template files.
Solution 3: Using WordPress Hooks
For a more advanced and flexible solution, you can leverage WordPress hooks to pass variables to get_template_part()
. WordPress provides various hooks, such as template_include
and get_template_part_{$slug}
, that allow you to modify the behavior of template files.
Here's an example of how you can use the template_include
hook to pass a variable:
<?php
function my_custom_template_include($template) {
$my_custom_variable = 123;
return get_template_part('element-templates/front', 'top', array('custom_variable' => $my_custom_variable));
}
add_filter('template_include', 'my_custom_template_include');
?>
In your front-top.php
file, you can access the variable like this:
<?php echo "My custom variable is: " . $args['custom_variable']; ?>
By using WordPress hooks, you have the flexibility to modify the behavior of get_template_part()
and pass variables in a more controlled and organized manner.
Conclusion
Passing variables to get_template_part()
in WordPress doesn't have to be a daunting task anymore. You can employ various techniques like using global variables, extract()
, or WordPress hooks to achieve the desired result.
Now it's your turn to give these solutions a try! Start experimenting with passing variables to get_template_part()
, and make your WordPress themes more adaptable and powerful. Happy coding! 🚀
Have any questions, comments, or alternative solutions? Let me know in the comments below! Let's discuss and learn from each other. 👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
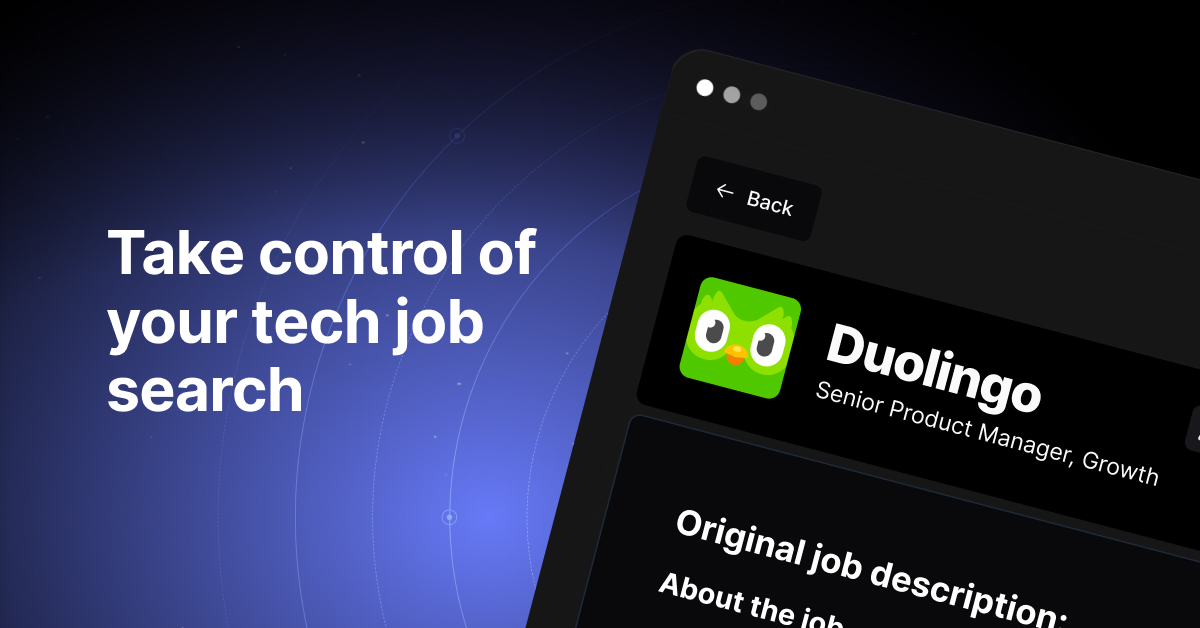