How to get last inserted row ID from WordPress database?
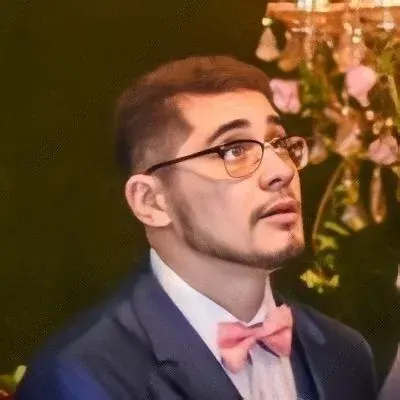
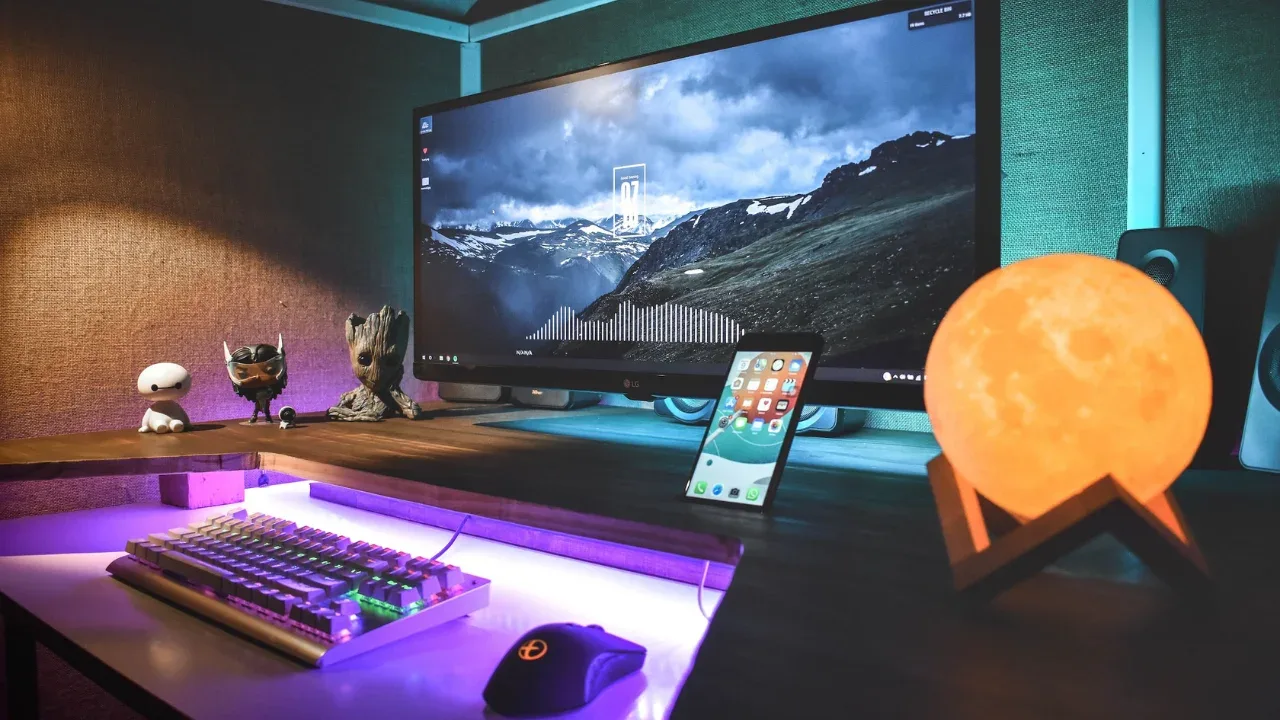
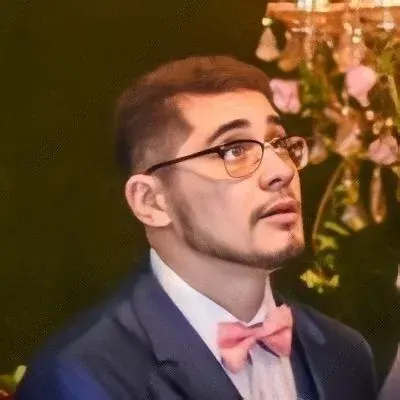
How to Get the Last Inserted Row ID from WordPress Database?
š Welcome to our tech blog! Today, we'll help you tackle the challenge of getting the last inserted row ID from a WordPress database. This is a common problem, especially when working with AJAX and multiple clients. But worry not, we've got some easy solutions for you! šŖ
Understanding the Problem
š¤ So, you have a WordPress plugin with a table that has an AUTO_INCREMENT primary key field called ID. When a new row is inserted into this table, you want to retrieve the ID value of the insertion. Makes sense!
⨠However, there's a twist! You're using AJAX to post data to the server and need to return the new row ID in the AJAX response to update the client's status. With multiple clients simultaneously posting data, you need to ensure that each AJAX request gets the exact new row ID in the response.
Solution 1: Use $wpdb's insert_id
Property
š” Good news! WordPress has a handy solution for you. Instead of relying on mysql_insert_id
, you can leverage the $wpdb
object, which is a global instance of the WordPress database class.
Here's how you can get the last inserted row ID using $wpdb
:
global $wpdb;
$wpdb->insert( 'your_table_name', $data );
$new_row_id = $wpdb->insert_id;
š Voila! By accessing the insert_id
property of $wpdb
, you can easily retrieve the ID of the last inserted row. This ensures that even in a race condition scenario, you get the correct ID for each AJAX request.
Solution 2: Execute Custom SQL Query
š” In case you prefer executing a custom SQL query, WordPress also provides a way to achieve that. Here's an example of how you can get the last inserted row ID through a custom query:
global $wpdb;
$wpdb->query( 'INSERT INTO your_table_name (column1, column2) VALUES (value1, value2)' );
$new_row_id = $wpdb->insert_id;
š With this approach, you directly execute the INSERT query using $wpdb->query
and then retrieve the last inserted row ID from the insert_id
property.
Conclusion
ā You've made it! Now you know two easy ways to get the last inserted row ID from a WordPress database, even when dealing with AJAX and multiple clients.
šŖ Next time you face a similar challenge, remember to use $wpdb
's insert_id
property or execute a custom SQL query through $wpdb->query
.
š We hope this guide has been helpful! If you have any questions or suggestions, feel free to drop a comment below. Happy coding! š©āš»šØāš»
š Don't forget to subscribe to our newsletter for more useful tech tips! š§
*[AJAX]: Asynchronous JavaScript and XML *[DB]: Database