How do I negate a condition in PowerShell?
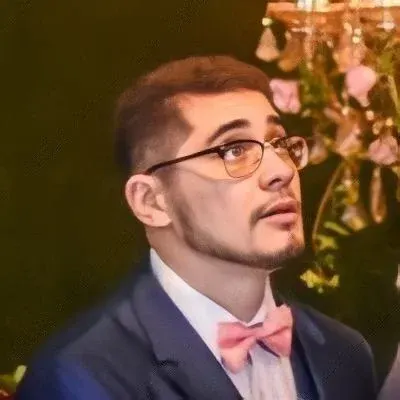
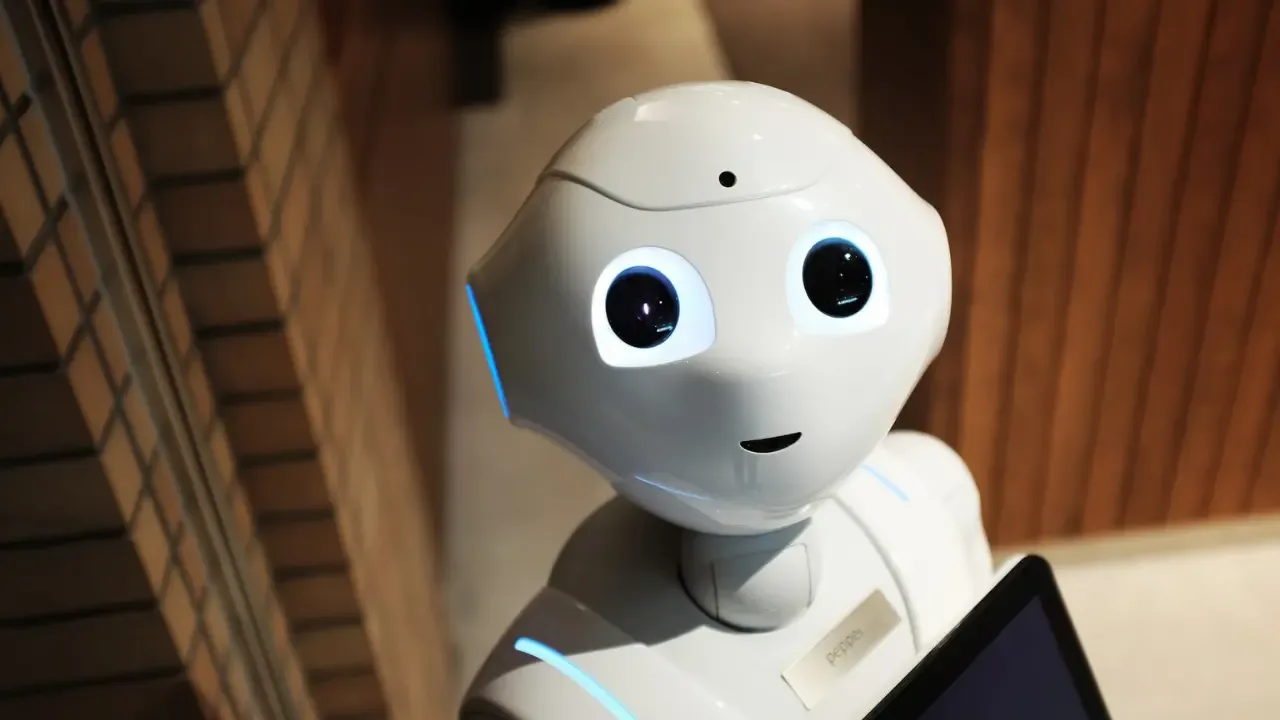
How to Negate a Condition in PowerShell: Easy Solutions for a Common Problem 😎🐍💻
So you're digging deep into the world of PowerShell and you've come across a common roadblock: how to negate a condition in your code. You want to check if a certain condition is not true and perform some action accordingly. Worry not, my fellow PowerShell enthusiast! In this blog post, I'll show you easy solutions to this problem that will leave you feeling like a PowerShell pro. Let's dive in! 💪
The Challenge 🤔
Here's the scenario you're facing. You want to check if the directory "C:\Code" exists using the Test-Path
cmdlet. If it does, you want to print "it exists!". Simple enough, right? Here's what your code might look like:
if (Test-Path C:\Code) {
Write-Host "it exists!"
}
But what if you want to do the opposite? How do you check if the directory doesn't exist and then print "it doesn't exist!"? Your first attempt might look like this (spoiler alert: it won't work):
if (Not (Test-Path C:\Code)){
Write-Host "it doesn't exist!"
}
The Workaround 💡
Fear not, my PowerShell friend! Even though the "Not" approach doesn't work as expected in this case, there's an easy workaround that will do the job.
if (Test-Path C:\Code) {
# Do nothing
}
else {
Write-Host "it doesn't exist!"
}
By leaving the if
block empty when the condition is true, and adding an else
block to handle the case when the condition is false, we can achieve our goal. This workaround gets the job done, but what if you prefer something more concise and inline? Don't worry, I've got you covered! 😉
A Neat In-Line Solution 🌟
Instead of using the if
statement, we can leverage the power of the ternary operator to achieve a more compact and inline solution. Check it out:
Write-Host $(if (Test-Path C:\Code) { "it exists!" } else { "it doesn't exist!" })
Boom! With this elegant one-liner, you're able to perform conditional checks and print the desired message in a concise and readable way. The ternary operator (condition) ? (if true) : (if false)
allows us to achieve this neat in-line solution.
Time to Level Up! ⏫
Congratulations, PowerShell wizard! You've learned how to negate a condition in PowerShell using easy solutions. Now it's time for you to take this knowledge and apply it to your own code. 💪✨
Have you ever come across other PowerShell challenges or do you have any questions? Share your experience and let's help each other become PowerShell superheroes in the comments below! 👇🚀
Don't forget to share this blog post with your friends and colleagues who might find it helpful. Sharing is caring! 😊🔗
Happy scripting and stay PowerShellful! 💻💡
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
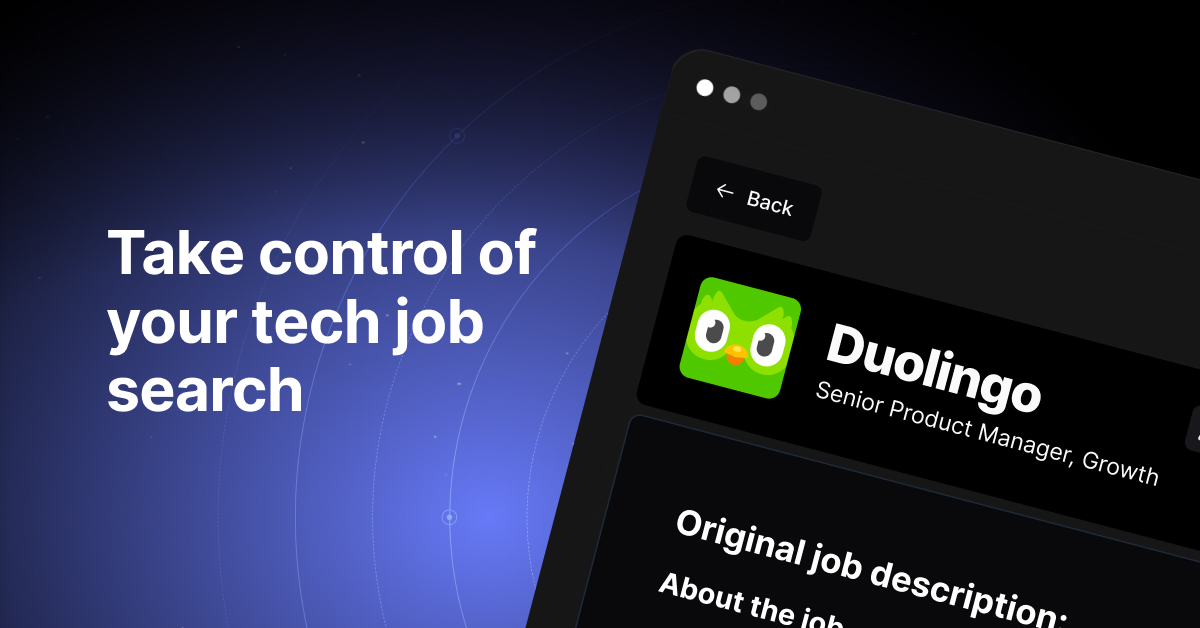