Batch file: Find if substring is in string (not in a file)
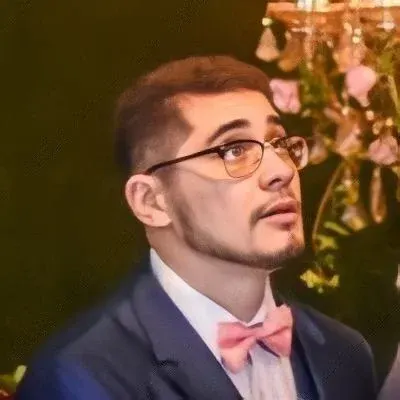
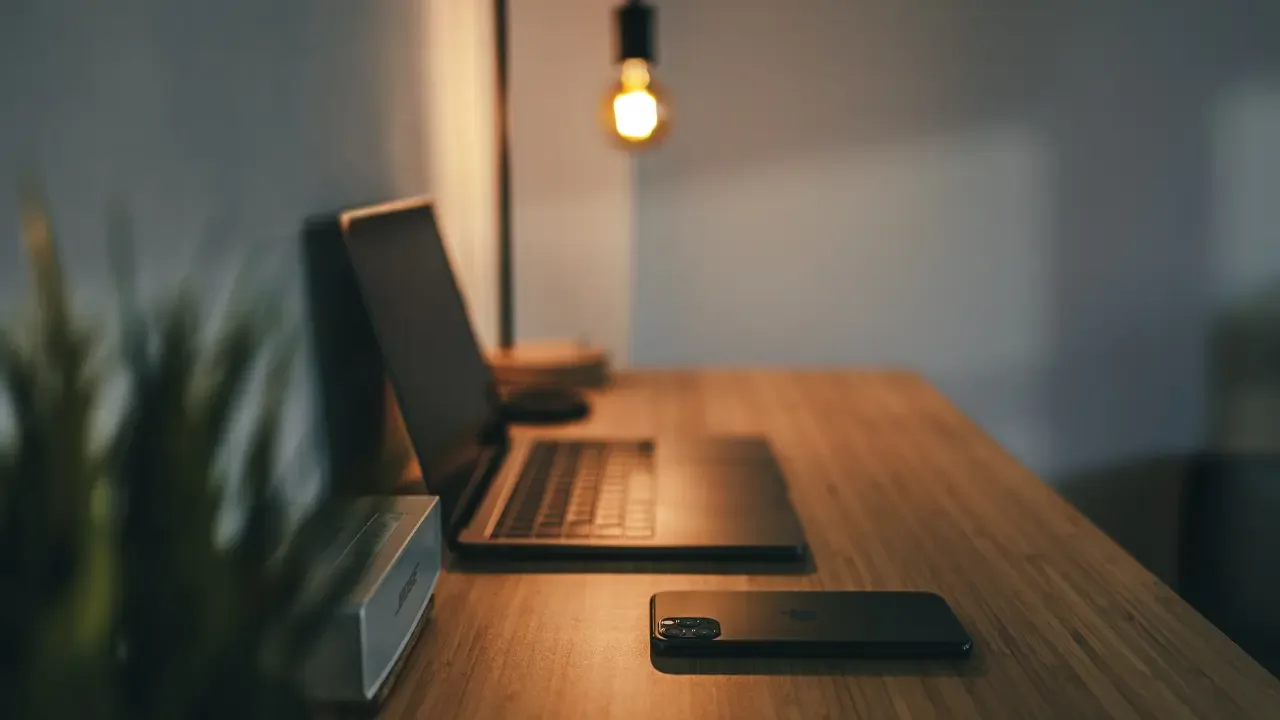
#🤖 Finding a Substring in a Batch File: Easy-Peasy Solution
So you've got a batch file and you're trying to find a substring within a string, but all the solutions you stumble upon are for searching substrings in files, not strings. Don't fret! I've got your back with an easy solution and a dash of fun! 🎉
##📝 Problem: Find Substring in String
The problem at hand is pretty straightforward. You've got a string, let's say "abcdefg"
, and you want to check if the substring "bcd"
is present within that string. But most solutions you find are tailored for searching substrings in files, not within a string. 😟
##💡 The "Greedy" Solution
Thankfully, there's a simple method to tackle this issue. Here's a batch file snippet that does just that:
@echo off
setlocal EnableDelayedExpansion
set "string=abcdefg"
set "substring=bcd"
if not "!string:%substring%=!"=="%string%" (
echo Substring found! 🎉
) else (
echo Substring not found. 😞
)
##🔍 Explanation
Let's break down this piece of genius code, shall we? 😉
setlocal EnableDelayedExpansion
enables delayed variable expansion, allowing us to work with variables inside loops or conditional statements.string=abcdefg
sets the value of our string variable to"abcdefg"
. You can replace it with your desired string.substring=bcd
sets the value of our substring variable to"bcd"
. Feel free to change it according to your needs.if not "!string:%substring%=!"=="%string%"
is where the magic happens! This line checks if the modified value ofstring
after removing thesubstring
is different from the originalstring
. If they differ, it means the substring is present, and the conditional statement echoes "Substring found! 🎉". Otherwise, it echoes "Substring not found. 😞".
##🚀 Your Turn!
Simple, right? Now it's your turn to give it a whirl! Modify the string
and substring
variables to match your desired inputs, save this snippet as a batch file, and run it. Voila! You'll get a clear verdict on whether the substring is present or not.
##🌟 Share Your Solutions!
I'd love to hear how this solution worked out for you! Feel free to drop a comment below and share your experience or any other creative solutions you've found. Let's learn from each other and make the coding world a happier place. 😄
Happy batch-file slicing! ✂️
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
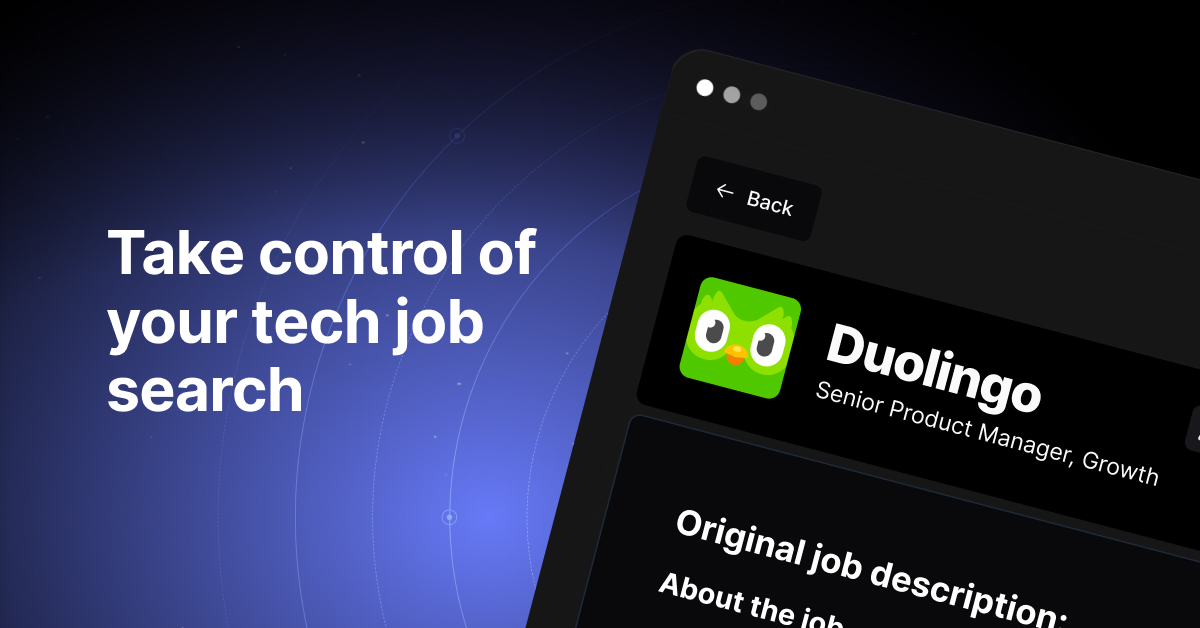