What are some good patterns for VBA error handling?
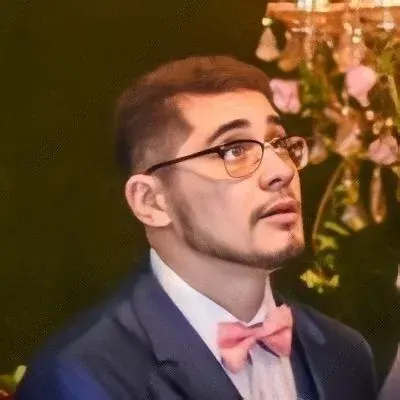
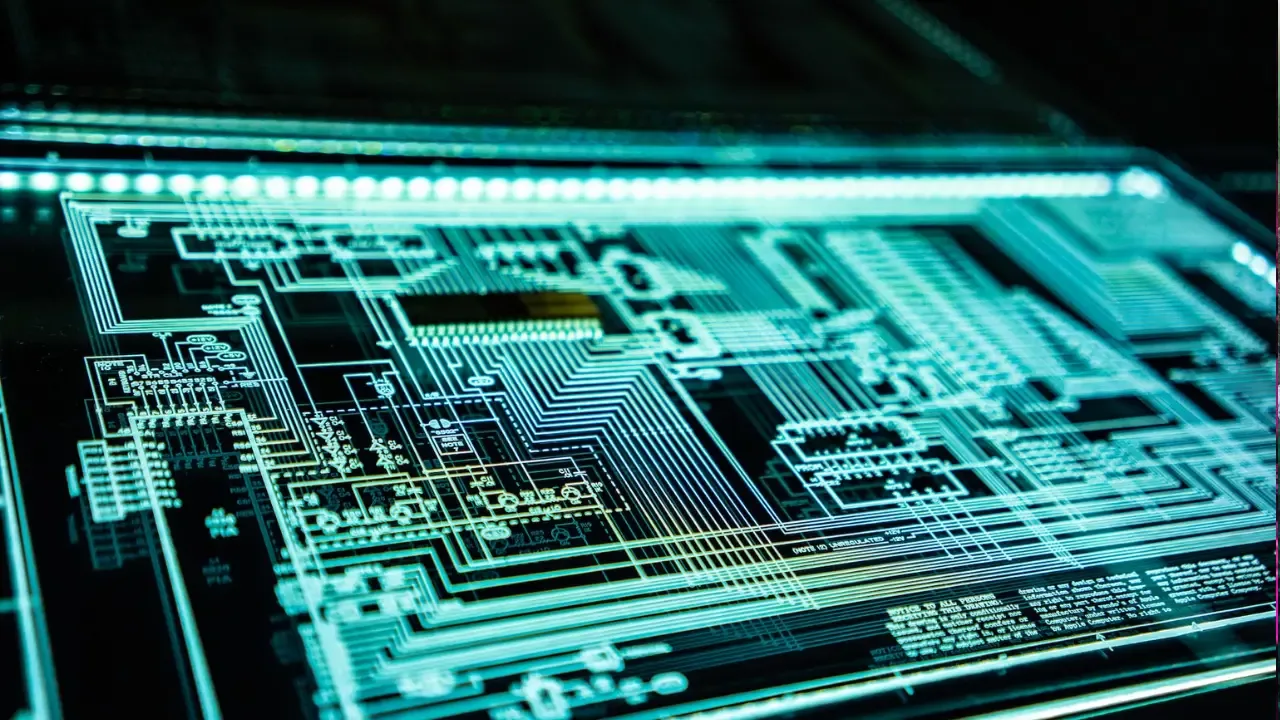
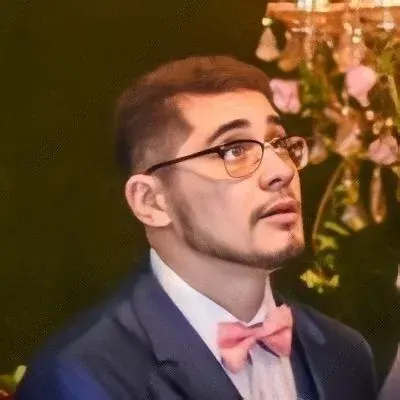
VBA Error Handling: A Guide to Handling Errors Like a Pro! ๐ ๏ธ
๐ Hey there, VBA enthusiasts! Are you struggling with error handling in your VBA code? ๐ค Don't worry, we've got you covered! In this blog post, we'll explore some good patterns for error handling in VBA and provide easy solutions to commonly encountered issues. So, let's dive right in and level up your error handling skills! ๐ช
The Scenario: Handling Errors and Running Finally Code
๐ค So, you have a code block where errors might occur, and you want to handle those errors. On top of that, you also want to ensure that a specific piece of code always runs, no matter what exceptions are thrown earlier. Sounds like a tricky situation, right? ๐ฎ But fret not, we have a way out!
The Solution: Using On Error, GoTo, and Resume
โก๏ธ In VBA, there are a few key concepts you should grasp to handle errors effectively. The On Error statement, GoTo statement, and Resume statement will be your best buddies in this journey! Let's see how they work together to achieve the desired outcome. ๐
Step 1: Enable Error Handling
๐น First things first, to enable error handling in your VBA code, include the following line at the beginning of your code module:
On Error GoTo ErrorHandler
This statement allows you to direct the flow of your code to a specific error handler when an error occurs.
Step 2: Define the Error Handler
๐น Next, you'll define your error handler. This is where you'll handle the error and resume code execution. Here's an example of how it could look:
ErrorHandler:
' Handle the error here
' ...
' Resume execution after the code where the error occurred
Resume Next
The ErrorHandler:
label marks the beginning of your error handler. Inside this block, you can handle the error using appropriate code, such as displaying a helpful message to the user or logging the error details. After you've handled the error, the Resume Next
statement will resume code execution after the line that caused the error.
Step 3: Run Finally Code
๐น Now, let's tackle the "finally" code that must always run, regardless of any exceptions. For this, we can utilize a CleanUp procedure in combination with the Finally pattern. Here's how it can be done:
Sub CleanUp()
' Code that must always run goes here
' ...
End Sub
Sub YourCode()
On Error GoTo ErrorHandler
' Your existing code here
' ...
' Your error handler code here
' ...
' Run finally code
CleanUp
Exit Sub
ErrorHandler:
' Error handling code here
' Resume code execution after the line that caused the error
Resume Next
End Sub
By encapsulating your "finally" code in a separate CleanUp procedure and calling it after error handling, you ensure that it runs every time, regardless of whether an error occurred or not. ๐งน
Engage, Share, and Level Up! ๐ฃ
๐ Congratulations, you've learned some good patterns for error handling in VBA! Now, it's time for you to practice and implement these techniques in your own projects. Remember, error handling is a crucial aspect of writing robust and reliable VBA code. ๐
๐ก If you found this guide helpful, don't keep it to yourself! Share it with your fellow coders who might be struggling with error handling. Let's spread the VBA love! ๐
๐ค We'd love to hear your thoughts and experiences with error handling in VBA. Have you encountered any tricky situations? How did you handle them? Let us know in the comments below! Let's learn from each other and level up our VBA game together! ๐
Keep coding with resilience and keep conquering those pesky errors! Happy VBAing! ๐ป๐ช