VBA to copy a file from one directory to another
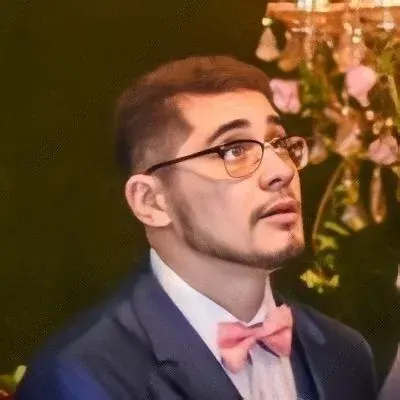
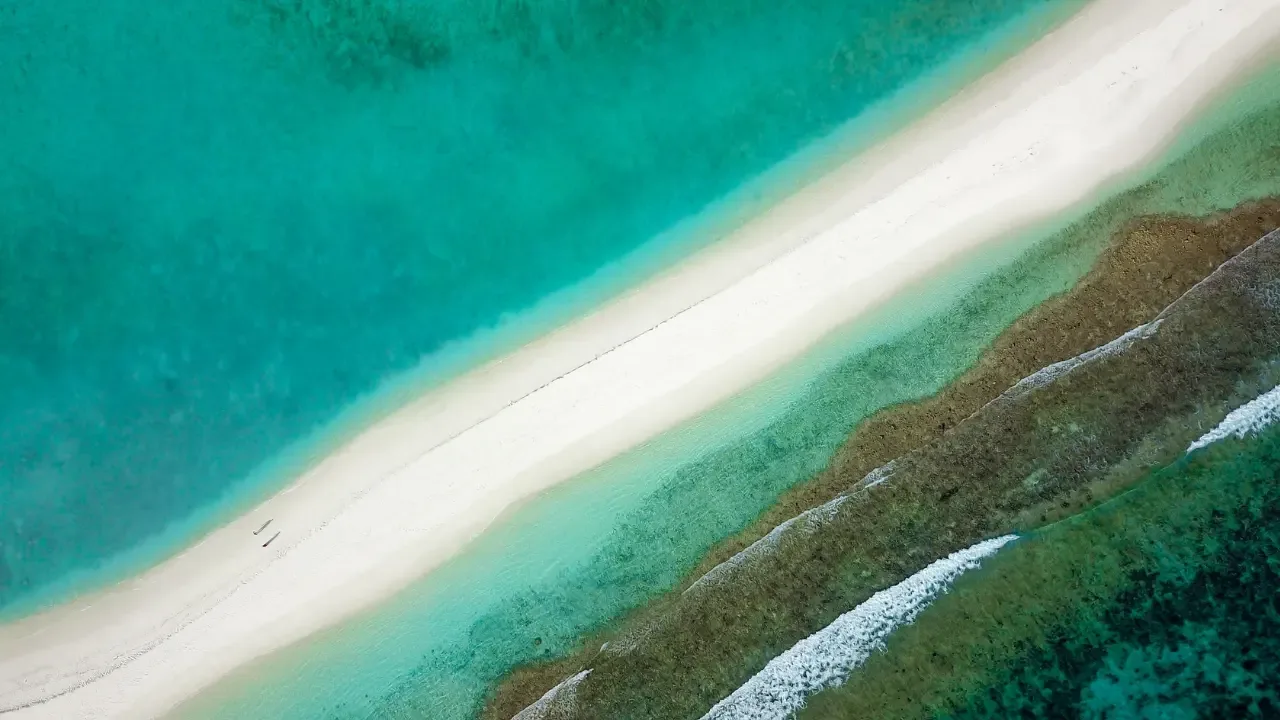
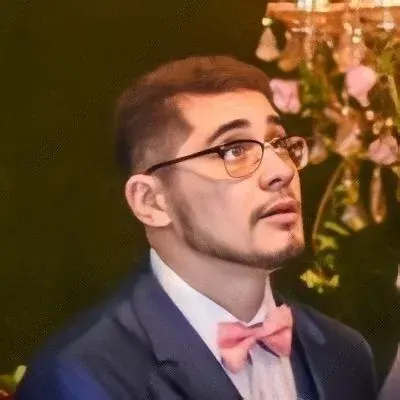
📝 VBA to Copy a File from One Directory to Another: A Complete Guide
Are you tired of manually copying and renaming files in different directories? Look no further! In this guide, we'll walk you through the process of using VBA (Visual Basic for Applications) to automate file copying and renaming in just a few simple steps. 🚀
💡 Understanding the Problem
Let's break down the problem statement first. You have an Access file that needs to be regularly copied to another directory. Additionally, you want to rename the file during the copying process.
Here's an example of the initial setup:
fileName = "X:\Database\oldName.accdb"
copyDestination = "Y:\dbstore\"
newName = "newName.accdb"
🛠️ The Solution
To tackle this problem, we'll be using VBA's FileSystemObject
to handle file operations. This object provides a convenient way to interact with files and folders programmatically.
Without further ado, let's dive into the code! Here's a step-by-step implementation of the solution:
Open the Visual Basic Editor in Excel by pressing
Alt + F11
.Insert a new module by clicking
Insert
➡️Module
.In the module, paste the following code:
Sub CopyAndRenameFile()
Dim FSO As Object
Dim sourceFilePath As String
Dim destinationFilePath As String
' Create a FileSystemObject
Set FSO = CreateObject("Scripting.FileSystemObject")
' Define the source file path
sourceFilePath = "X:\Database\oldName.accdb"
' Define the destination file path
destinationFilePath = "Y:\dbstore\" & "newName.accdb"
' Check if the source file exists
If FSO.FileExists(sourceFilePath) Then
' Check if the destination file already exists and delete it
If FSO.FileExists(destinationFilePath) Then
FSO.DeleteFile destinationFilePath
End If
' Copy the file to the destination directory
FSO.CopyFile sourceFilePath, destinationFilePath
' Notify the user that the file has been copied and renamed
MsgBox "File copied and renamed successfully!"
Else
' Notify the user if the source file does not exist
MsgBox "Source file does not exist!"
End If
' Clean up the FileSystemObject
Set FSO = Nothing
End Sub
Modify the
sourceFilePath
,destinationFilePath
, andnewName
variables to fit your specific file paths and desired file name.
💥 Common Issues and Troubleshooting
While the provided solution should work seamlessly, you might encounter a few common issues. Here are a couple of troubleshooting tips to help you out:
File Not Found: Ensure that the source file exists at the specified path. Double-check the file name and directory to eliminate any typos.
Permission Denied: Make sure you have the necessary permissions to access and modify both the source and destination folders. Contact your system administrator if needed.
📣 Your Turn to Automate!
Now that you have the code and understand the process, it's time to put it into action! Don't dread the repetitive file copying and renaming tasks anymore. Use this VBA solution to streamline your workflow and save valuable time. 🎉
Give it a try and let us know how it worked for you! If you have any questions or come across any issues, feel free to leave a comment below. We're always here to help you out. 😊
Happy automating!