VBA - how to conditionally skip a for loop iteration
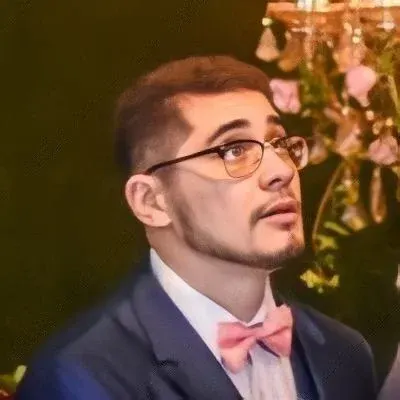
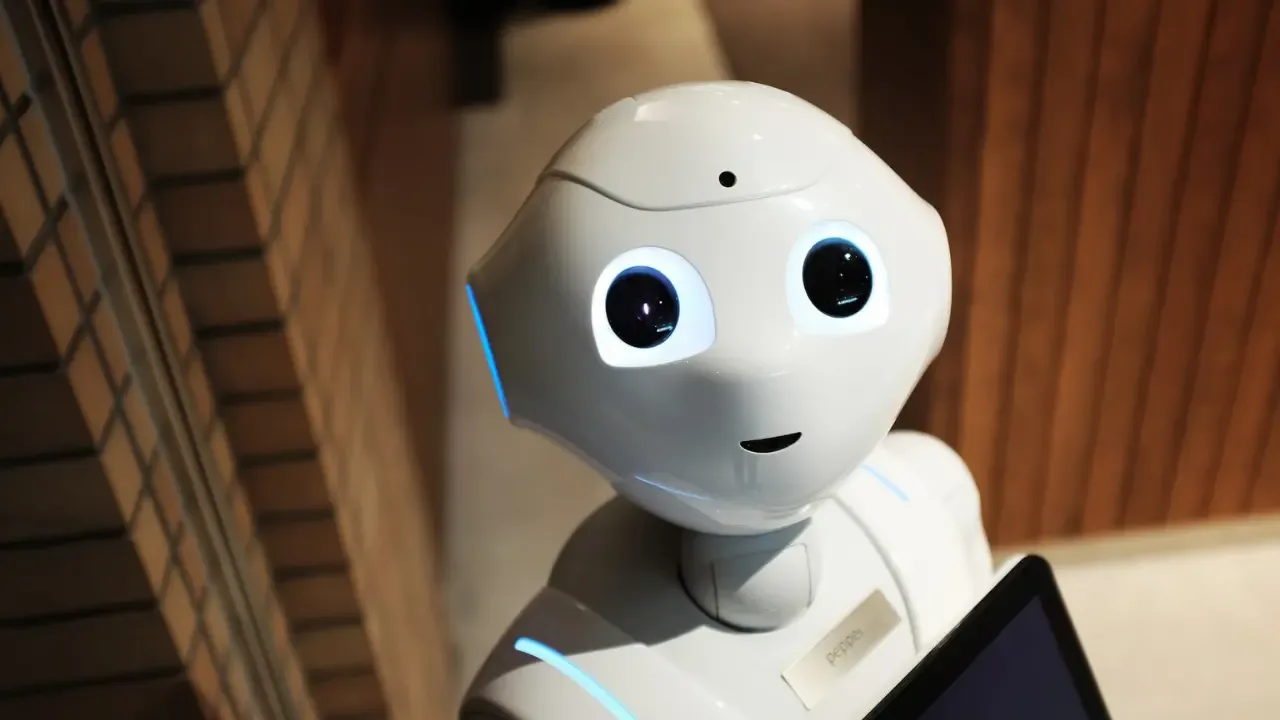
💡 VBA: Conditionally Skipping a For Loop Iteration
So, you're writing some VBA code, looping through an array, and you want to skip certain iterations based on a condition. But you also want to keep track of the last value of the iterator variable. 🔄
You've tried using Continue
and Next
, but unfortunately, they don't work like you expected. 😕
No worries, I've got some easy solutions for you! Let's tackle this problem step by step. 👣
Understanding the Issue 🧐
In VBA, we don't have a built-in Continue
statement like some other programming languages. Instead, we need to find alternative methods to achieve the desired behavior. 🔄
In the given code snippet, the line PrevCouponIndex = i
suggests that the variable PrevCouponIndex
needs to store the last value of i
before skipping the iteration. You also want to exit the loop gracefully if the condition is met.
Solution 1: Using GoTo Label 🏷
One way to achieve this behavior is by using a label and the GoTo
statement. 😮
Here's an updated code snippet:
For i = LBound(Schedule, 1) To UBound(Schedule, 1)
If (Schedule(i, 1) < ReferenceDate) Then
PrevCouponIndex = i
GoTo SkipIteration
End If
DF = Application.Run("SomeFunction"....)
PV = PV + (DF * Coupon / CouponFrequency)
SkipIteration:
Next
In this approach, we introduce a label SkipIteration
right above the Next
statement. When the condition is met, the code jumps to the label, skipping the rest of the loop and continuing with the next iteration. Meanwhile, the value of i
is held by PrevCouponIndex
. 🎶
Solution 2: Using Another Loop 🔄
Another approach involves utilizing an additional loop in combination with an Exit For
statement. 😮
Here's an alternative code snippet:
For i = LBound(Schedule, 1) To UBound(Schedule, 1)
If (Schedule(i, 1) < ReferenceDate) Then
PrevCouponIndex = i
Exit For
Else
DF = Application.Run("SomeFunction"....)
PV = PV + (DF * Coupon / CouponFrequency)
End If
Next i
In this solution, we exit the loop prematurely using Exit For
when the condition is met, effectively skipping the remaining iterations. By checking the condition again within the loop, we ensure that the desired code inside the loop is executed for other cases. 🔄
Conclusion and Your Turn 🤓🖊
And there you have it! Two simple yet effective solutions to conditionally skip a for loop iteration in VBA. Now you can choose the approach that suits your needs. 🎉
But wait, before you go, I'd love to hear from you! Have you encountered this problem before, or do you have any other VBA questions? Feel free to share your thoughts, experiences, or further questions in the comments below. Let's geek out together! 🤓💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
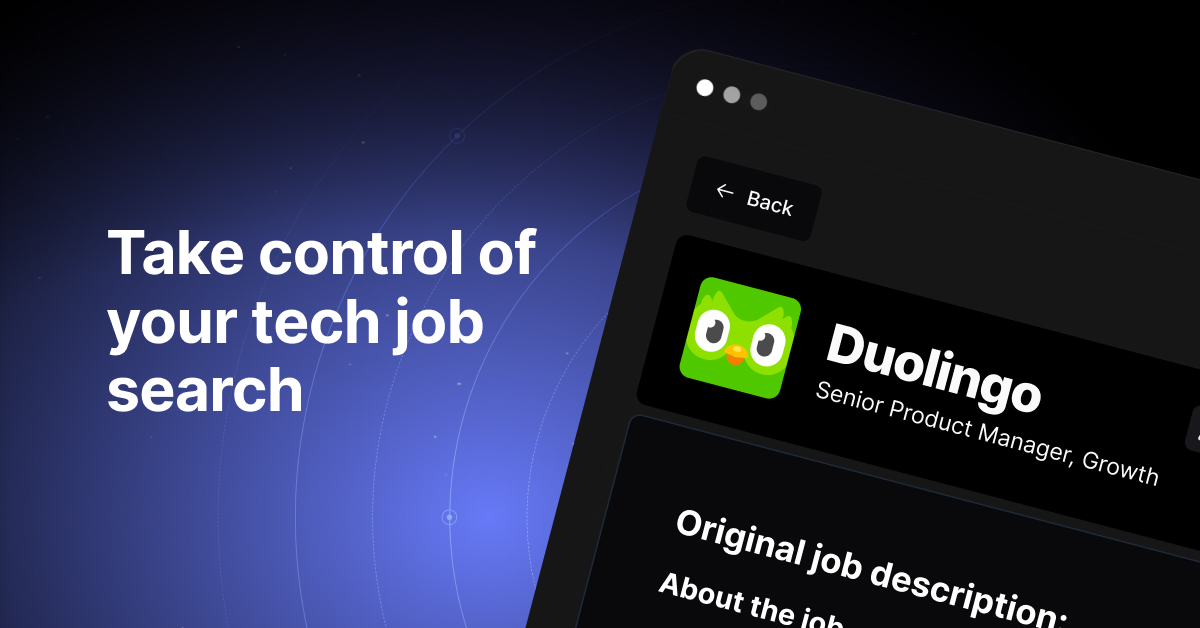