Pass arguments to Constructor in VBA
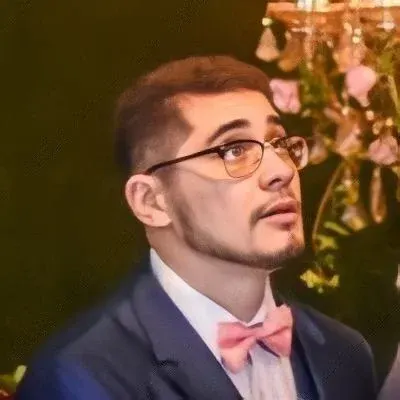
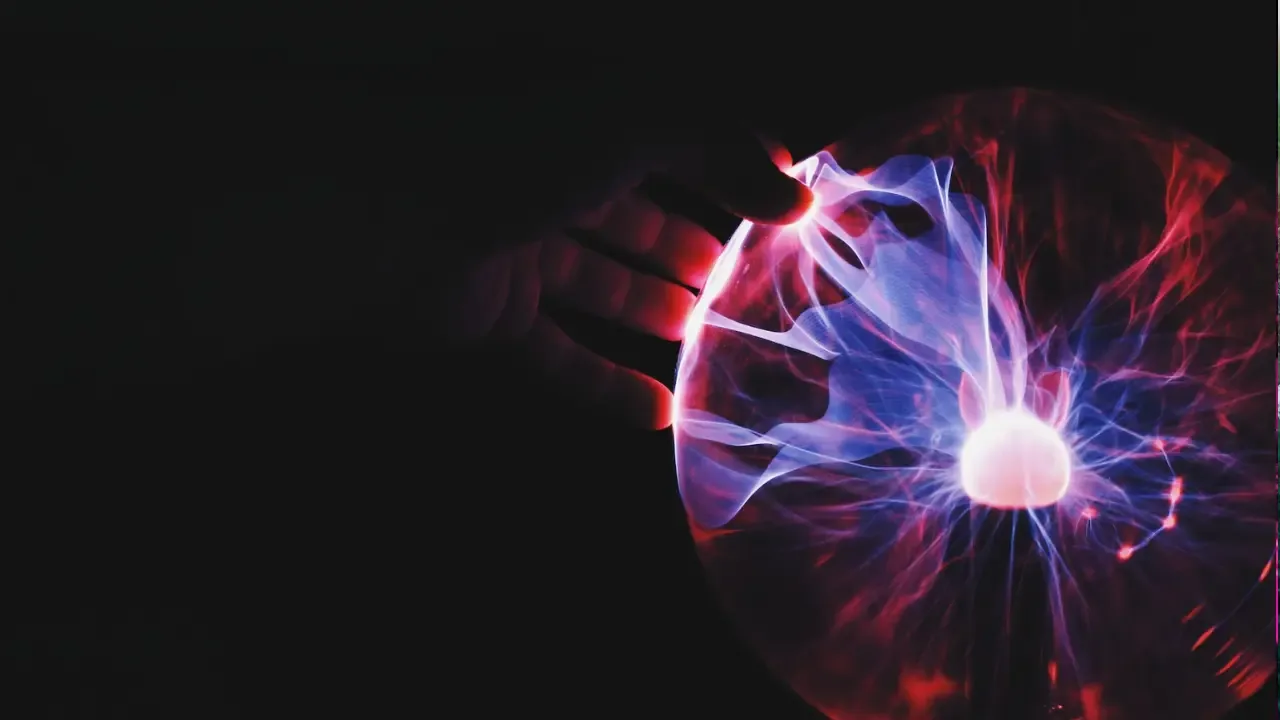
Construct Objects with Arguments in VBA: A Simple Solution 💻
Are you tired of not being able to pass arguments directly to your own classes in VBA? 😫 We feel your pain! It can be frustrating to work with dirty workarounds just to construct objects with arguments. But fear not! We have an easy solution that will save you time and energy. 🎉
The Problem: Annoying Limitations 😩
In VBA, constructing objects with arguments can be a real headache. By default, VBA only supports passing arguments to functions and subroutines. This means that when it comes to constructing objects, you're often left with limited options. 😓
The Solution: Named Arguments 🚀
Enter named arguments, the knight in shining armor of VBA developers! Using named arguments, you can conveniently pass arguments to constructors and simplify your code. 🌟
Let's take a look at how it works:
Dim this_employee As Employee
Set this_employee = New Employee(name:="Johnny", age:=69)
Voila! With named arguments, you can now pass the name
and age
arguments directly to the Employee
constructor. It's as simple as that! 😎
Example Code: Employee Class 🧑💼
To better illustrate the power of named arguments, let's create an example Employee
class.
Here's the code for our Employee
class:
Private mName As String
Private mAge As Integer
Public Property Let Name(value As String)
mName = value
End Property
Public Property Let Age(value As Integer)
mAge = value
End Property
Public Sub PrintInfo()
Debug.Print "Name: " & mName
Debug.Print "Age: " & mAge
End Sub
With the named arguments approach, you can now easily create new employees and set their properties without any hassle. 🙌
Why You'll Love This Solution 💖
Saves Time: Say goodbye to convoluted workarounds! With named arguments, you can quickly and efficiently pass arguments to constructors.
Simplifies Code: Named arguments make your code more readable and maintainable. It's easier for anyone reading your code to understand the purpose of each argument.
Avoids Errors: By explicitly stating the argument name when passing values, you reduce the chance of accidentally swapping the order of arguments.
Your Turn to Shine! 🌟
Now that you know how to pass arguments to constructors in VBA using named arguments, it's time to level up your code! ✨
We encourage you to give it a try in your own projects and experience the benefits firsthand. If you come across any challenges or have questions, feel free to drop us a comment. We're here to help! 🤝
Happy coding! 💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
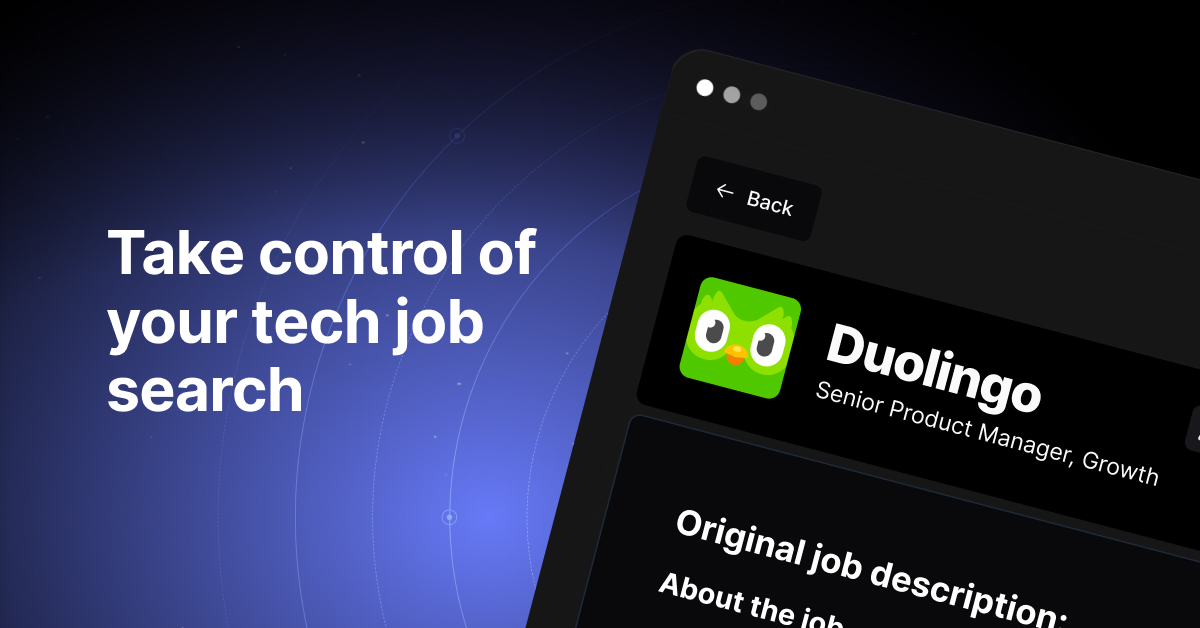