Loop Through All Subfolders Using VBA
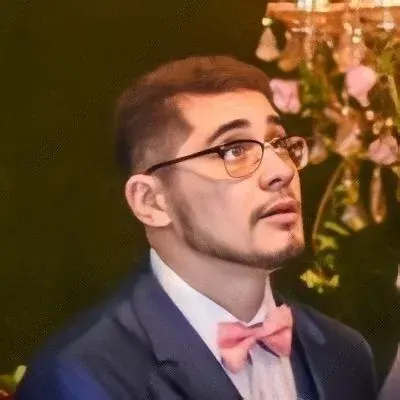
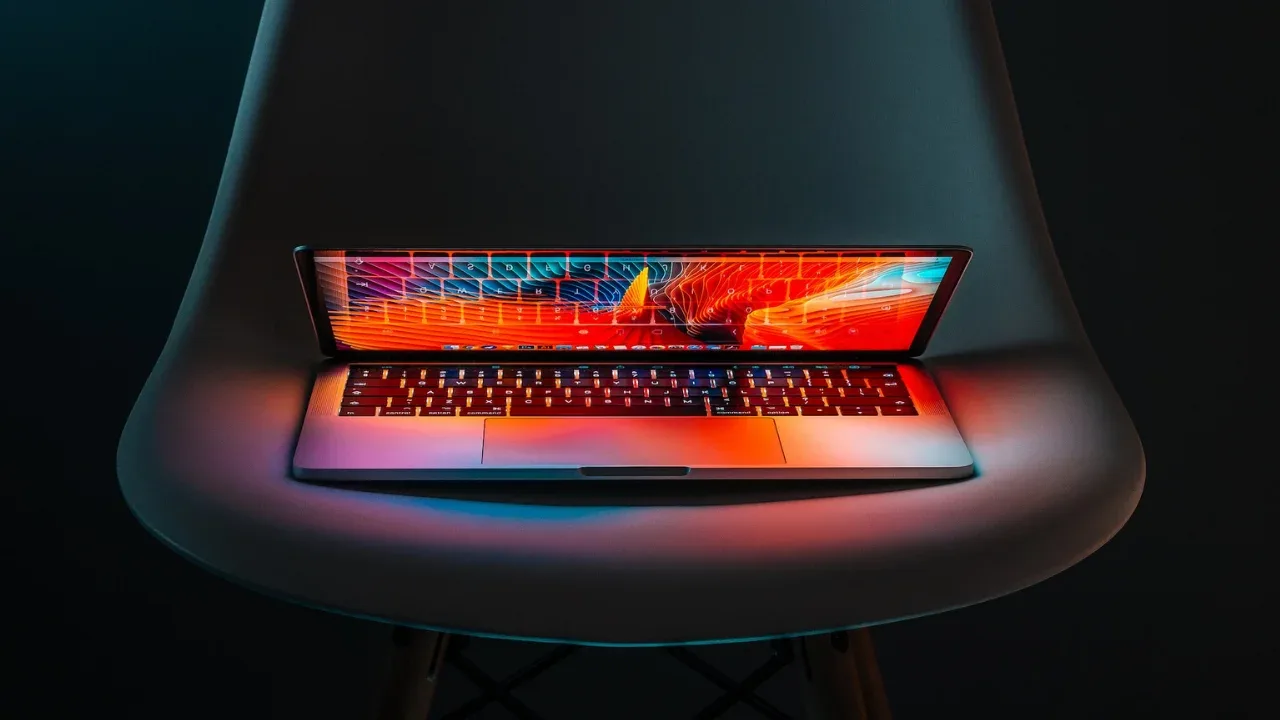
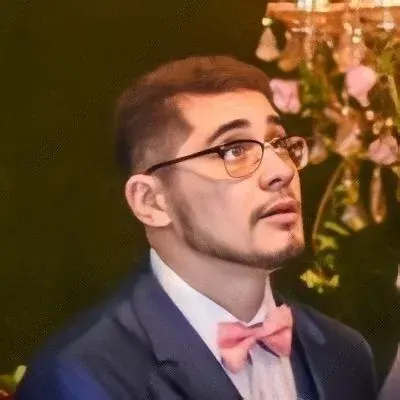
Easy Ways to Loop Through All Subfolders Using VBA 📂💻
Are you struggling to write a VBA script that will loop through all subfolders of a specified folder? Don't worry, we've got you covered! In this blog post, we'll address the common issues associated with this problem and provide you with easy solutions. So, let's dive in and conquer this challenge together! 💪
Understanding the Problem 🤔
Before we jump into the solutions, let's understand the problem at hand. As per the context provided, you need to loop through all subfolders of a specified folder, irrespective of the number of nested layers. Though the number of nested subfolders might not exceed 3 or 4, it's essential to consider infinite possibilities.
Solution 1: Recursion to the Rescue! 🔄
One way to tackle this problem is by using recursion. Recursion is a powerful technique where a function calls itself repeatedly until a specific condition is met. In our case, the function will loop through all the subfolders until no more subfolders are found.
Here's an example of how you can implement recursive subfolder looping using VBA:
Sub LoopThroughSubfolders(ByVal folderPath As String)
Dim fso As Object
Dim folder As Object
Dim subfolder As Object
Set fso = CreateObject("Scripting.FileSystemObject")
Set folder = fso.GetFolder(folderPath)
For Each subfolder In folder.Subfolders
' Perform your desired operations here on each subfolder
' Recursive call to process subfolders inside the current subfolder
LoopThroughSubfolders subfolder.Path
Next subfolder
End Sub
Now you can call the LoopThroughSubfolders
subroutine and provide the path of the main folder as the argument. The code will automatically loop through all the subfolders, no matter how many nested layers they have.
Sub Main()
LoopThroughSubfolders "C:\Your\Folder\Path"
End Sub
This recursive approach allows for flexible and efficient subfolder processing, regardless of the directory structure. 🚀
Solution 2: Using a Stack 📚
Another way to loop through all subfolders is by utilizing a stack data structure. This approach avoids recursion, which can sometimes lead to stack overflow errors if the number of subfolders is exceedingly large.
Here's an example of how you can implement the stack-based subfolder looping using VBA:
Sub LoopThroughSubfolders(ByVal folderPath As String)
Dim fso As Object
Dim folderStack As Collection
Dim currentFolder As Object
Dim subfolder As Object
Set fso = CreateObject("Scripting.FileSystemObject")
Set folderStack = New Collection
folderStack.Add fso.GetFolder(folderPath)
Do While folderStack.Count > 0
Set currentFolder = folderStack(1)
folderStack.Remove 1
For Each subfolder In currentFolder.Subfolders
' Perform your desired operations here on each subfolder
folderStack.Add subfolder
Next subfolder
Loop
End Sub
Similarly, you can call the LoopThroughSubfolders
subroutine with the desired folder path to start the subfolder looping process.
Call-to-Action: Share Your Success Story! 🎉
Now that you have easy solutions to loop through all subfolders using VBA, it's time to put them into action. Try out the provided code examples and let us know how it worked for you. Share your success story in the comments section below and help others facing similar problems. Together, we can make the coding world a better place! 🌟💬
That's it, folks! We hope this blog post has been helpful in empowering you to tackle the challenge of looping through all subfolders using VBA. Stay tuned for more tech tips, tricks, and solutions. Happy coding! 👩💻👨💻