Levenshtein Distance in VBA
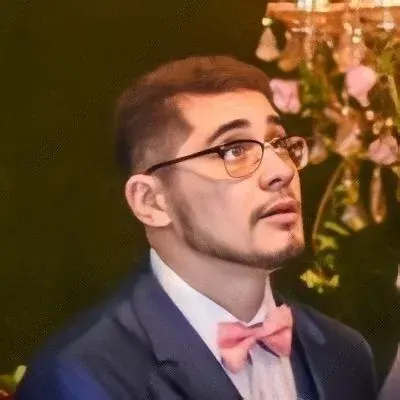
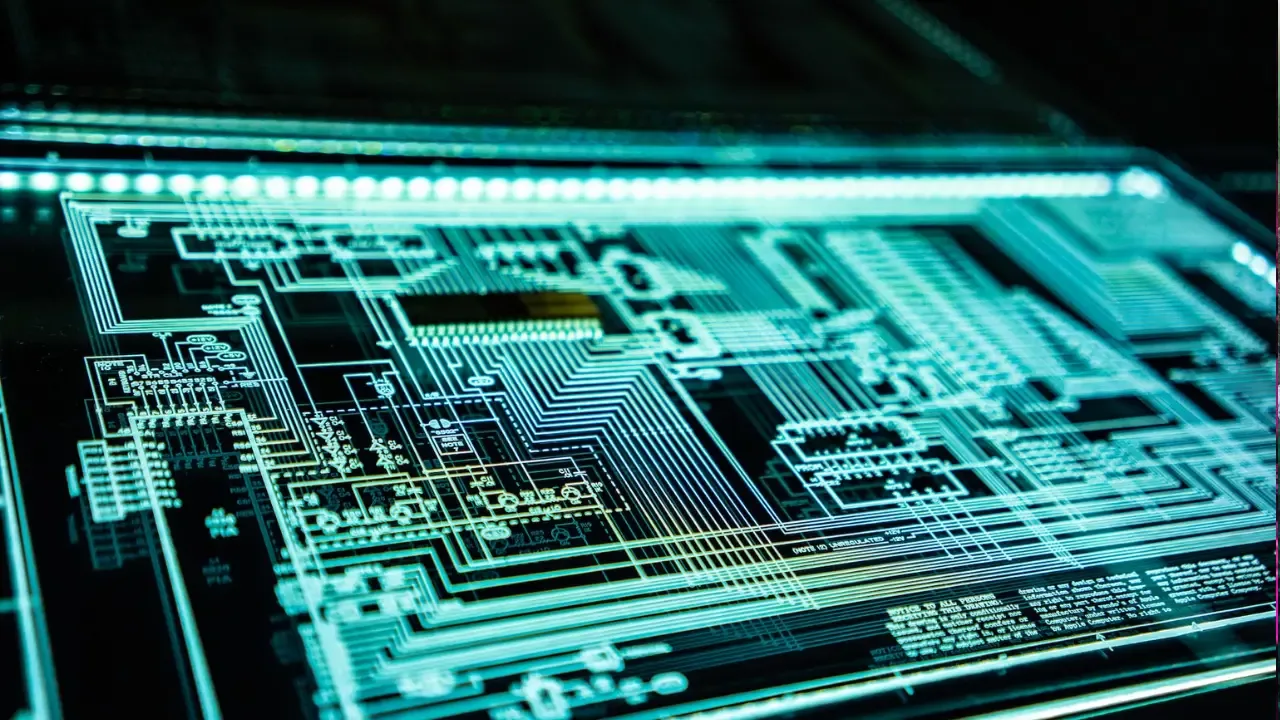
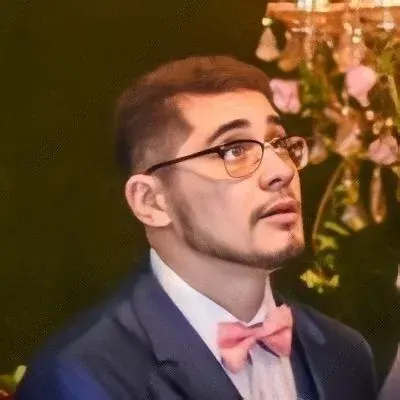
Calculating Levenshtein Distance in VBA: A Complete Guide 📝
Have you ever found yourself in a situation where you needed to calculate the Levenshtein Distance between two strings in VBA? Perhaps you have a large Excel sheet with data and you want to determine the similarity between different values. Fear not! In this blog post, we will explore how you can easily programatically calculate the Levenshtein Distance in VBA. Let's dive in!
Understanding the Levenshtein Distance 📏
The Levenshtein Distance is a measure of the difference between two strings. It quantifies the minimum number of edits (insertions, deletions, or substitutions) needed to transform one string into another. This distance can be a useful metric for tasks such as spell checking, DNA sequence alignment, and fuzzy string matching.
Common Approaches and Challenges ✋
Before we jump into the solution, let's take a moment to discuss some common approaches and challenges you may encounter when calculating Levenshtein Distance in VBA.
Approach 1: Brute Force Method 🔨
One straightforward approach is to use a brute force method that recursively calculates the Levenshtein Distance for each subproblem. However, this approach can be computationally expensive and inefficient for large strings. It is important to optimize our solution to handle such scenarios effectively.
Approach 2: Dynamic Programming 🚀
Dynamic programming offers a more efficient solution for calculating the Levenshtein Distance. This technique involves breaking down the problem into smaller, overlapping subproblems and solving them systematically. By leveraging memoization, we can store intermediate results to avoid redundant calculations and improve performance.
Now that we understand the challenges, let's move on to the solution.
Solutions for Calculating Levenshtein Distance in VBA ✔️
To calculate the Levenshtein Distance in VBA, we can implement the dynamic programming approach described earlier. Here's an example function that does exactly that:
Function LevenshteinDistance(ByVal str1 As String, ByVal str2 As String) As Integer
Dim m As Integer
Dim n As Integer
Dim i As Integer
Dim j As Integer
Dim cost As Integer
Dim d() As Integer
m = Len(str1)
n = Len(str2)
ReDim d(0 To m, 0 To n)
For i = 0 To m
d(i, 0) = i
Next i
For j = 0 To n
d(0, j) = j
Next j
For i = 1 To m
For j = 1 To n
cost = IIf(Mid(str1, i, 1) = Mid(str2, j, 1), 0, 1)
d(i, j) = WorksheetFunction.Min3( _
d(i - 1, j) + 1, _
d(i, j - 1) + 1, _
d(i - 1, j - 1) + cost)
Next j
Next i
LevenshteinDistance = d(m, n)
End Function
The LevenshteinDistance
function takes two string parameters, str1
and str2
, and returns the calculated Levenshtein Distance as an integer. This code utilizes a dynamic programming table d
to store the calculated distances for each prefix of the strings.
To calculate the distance, we iterate over the characters of both strings and update the table accordingly. The WorksheetFunction.Min3
function is used to find the minimum value among three possibilities: deletion, insertion, or substitution.
Call-to-Action: Share Your Experience! 💬
Now that you have a working solution to calculate the Levenshtein Distance in VBA, give it a try in your own projects and let us know how it worked for you! Do you have any other tips or tricks for handling string similarity in VBA? Share your experiences and insights in the comments below.
Remember, understanding how to calculate the Levenshtein Distance can be crucial when working with string comparisons, data cleaning, or text analysis tasks. So bookmark this guide for future reference and share it with your friends who are looking for an easy solution in VBA!
Happy coding! 💻✨