Is there an equivalent to Thread.Sleep() in VBA
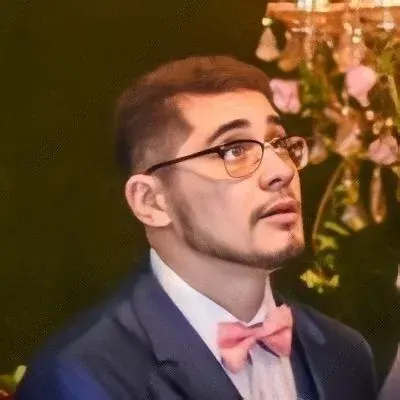
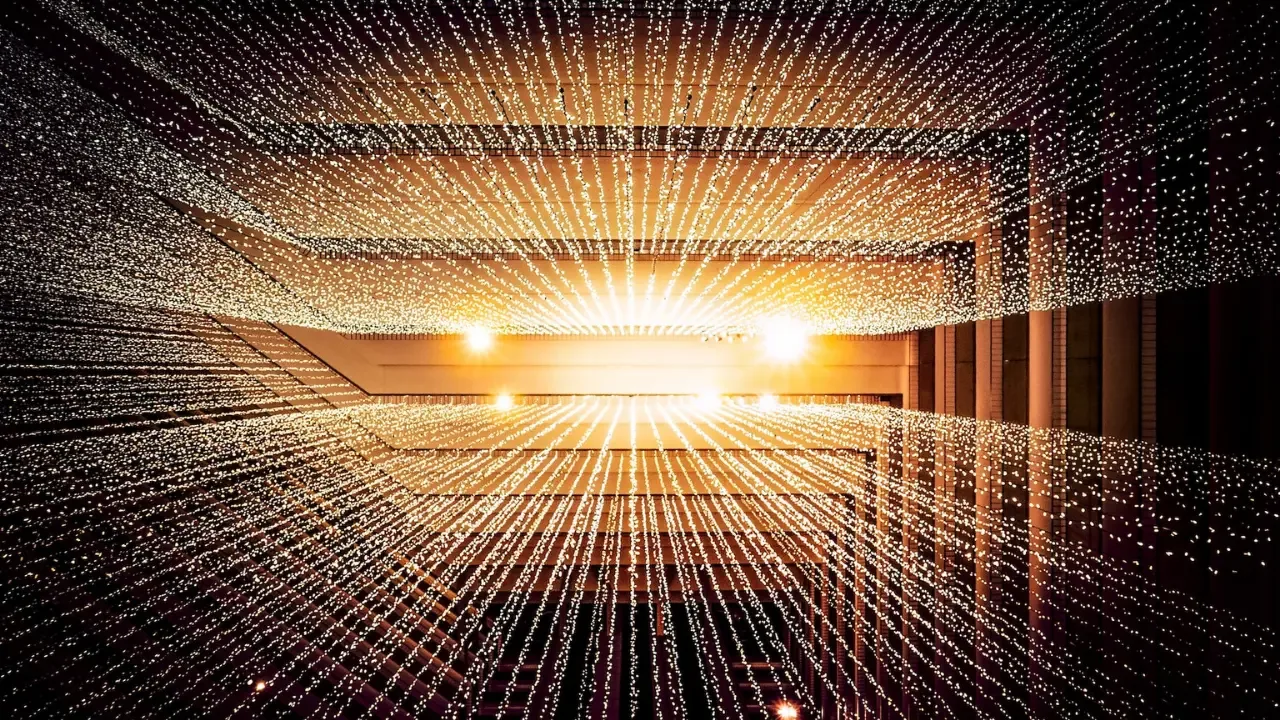
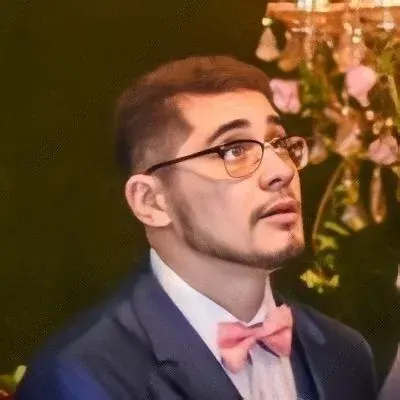
💤 Is there an equivalent to Thread.Sleep() in VBA? 💤
Have you ever found yourself in a situation where you needed to introduce a delay or pause in your VBA code? Maybe you wanted to create a more realistic simulation or ensure that certain actions happen in a specific order. If you're familiar with languages like C# or Java, you might be wondering if there's an equivalent to the convenient Thread.Sleep()
method in VBA.
Well, I have good news and bad news. The bad news is that VBA doesn't have a built-in equivalent to Thread.Sleep()
. The good news is that there are workarounds and alternative solutions that can help you achieve the desired effect. Let's explore some common issues and specific problems you might encounter, along with easy solutions.
🛑 Issue: VBA doesn't have Thread.Sleep()
In VBA, the lack of a direct equivalent to Thread.Sleep()
can be frustrating, especially when you're used to having this feature in other programming languages. However, instead of getting discouraged, let's look at some options to simulate a similar behavior.
📉 Solution 1: Application.Wait()
One way to introduce a delay in your VBA code is by using the Application.Wait()
method. This method allows you to pause the code execution for a specified duration, expressed in seconds.
Here's an example that demonstrates how to pause the code for 3 seconds:
Sub Example1()
' Do some operations
' Pause for 3 seconds
Application.Wait Now + TimeValue("00:00:03")
' Continue with the rest of the code
End Sub
By using Application.Wait()
, you can easily introduce delays in your VBA code.
🔄 Solution 2: DoEvents()
Another alternative to simulate a delayed execution in VBA is by using the DoEvents()
function. This function allows the code to yield control back to the operating system, giving it a chance to process other events in the application's message queue.
Here's an example that demonstrates how to introduce a 3-second delay using DoEvents()
:
Sub Example2()
' Do some operations
' Store the current time
Dim startTime As Double
startTime = Timer
' Loop until 3 seconds have passed
Do While Timer < startTime + 3
' Yield control to the operating system
DoEvents
Loop
' Continue with the rest of the code
End Sub
By calling DoEvents()
in a loop, you can create a delay similar to what Thread.Sleep()
provides in other programming languages.
💡 Conclusion
While VBA doesn't have a direct equivalent to Thread.Sleep()
, you can easily introduce delays in your code using alternatives like Application.Wait()
or DoEvents()
. These workarounds allow you to control the timing and flow of your VBA programs.
Next time you find yourself needing a pause or delay in your VBA code, remember these handy solutions. Experiment, have fun, and share your experiences!
Now it's your turn! Have you encountered any challenges with delays in VBA? How did you solve them? Share your thoughts and experiences in the comments below. Let's connect and learn from each other!