How to check for empty array in vba macro
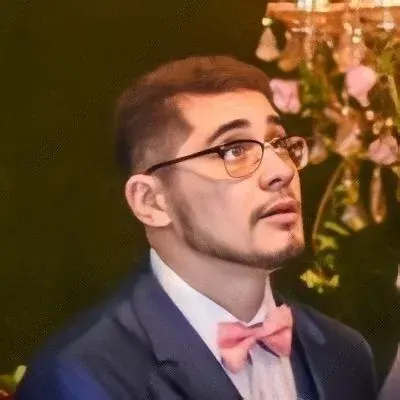
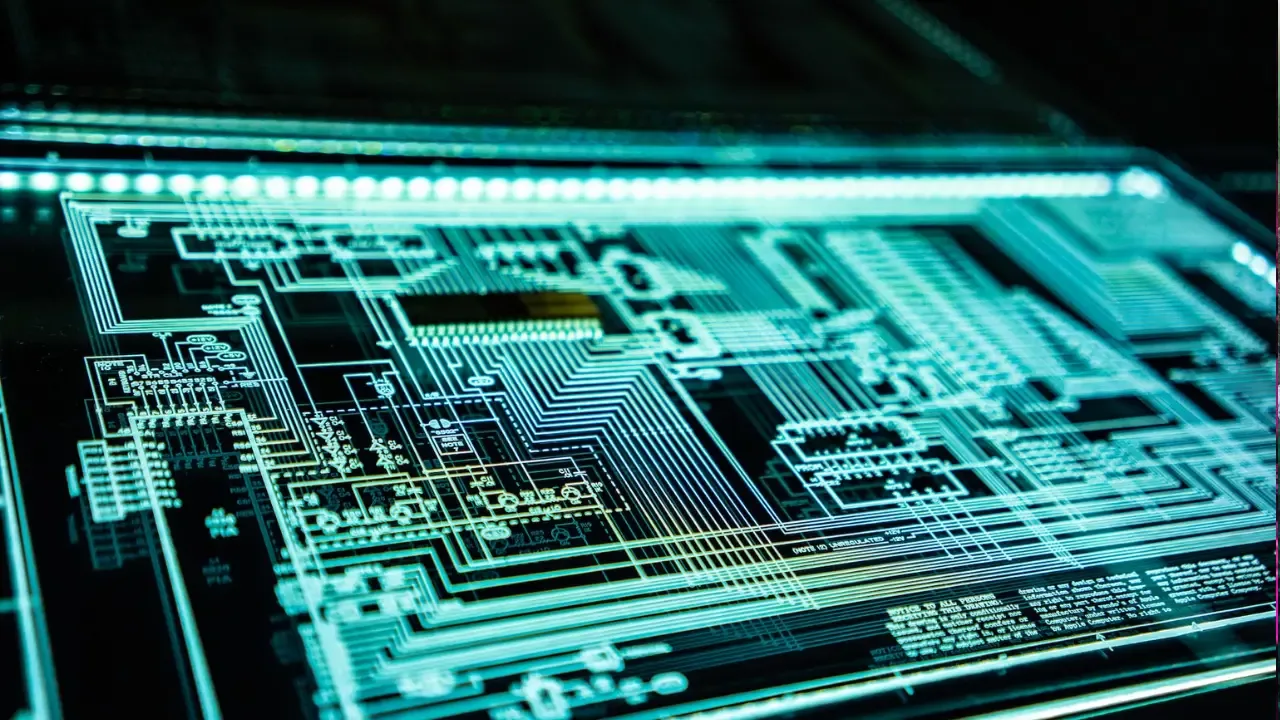
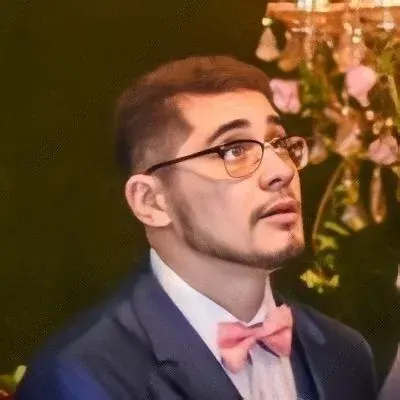
How to Check for Empty Arrays in VBA Macro
So, you want to check for empty arrays in VBA Macro, but you've tried various solutions from Google and none of them worked. Don't worry, you're not alone! Many people struggle with this issue because it can be a bit tricky. But fear not, we're here to help!
The Problem
Let's take a look at the code snippet you provided:
Dim FileNamesList As Variant, i As Integer
' activate the desired startfolder for the filesearch
FileNamesList = CreateFileList("*.*", False) ' Returns File names
' performs the filesearch, includes any subfolders
' present the result
' If there are Signatures then populate SigString
Range("A:A").ClearContents
For i = 1 To UBound(FileNamesList)
Cells(i + 1, 1).Formula = FileNamesList(i)
Next i
SigString = FileNamesList(3)
If Dir(SigString) <> "" Then
Signature = GetBoiler(SigString)
Else
Signature = ""
End If
The problem arises when the FileNamesList
array is empty. In this case, the SigString
variable also becomes empty, leading to an error when calling the GetBoiler()
function with an empty string.
The Solution
To avoid this error, we need to first check if the array is empty before proceeding with the GetBoiler()
function. Here's how you can modify your code to handle this situation:
Dim FileNamesList As Variant, i As Integer
' activate the desired startfolder for the filesearch
FileNamesList = CreateFileList("*.*", False) ' Returns File names
' performs the filesearch, includes any subfolders
' present the result
' If there are Signatures then populate SigString
Range("A:A").ClearContents
If Not IsArrayEmpty(FileNamesList) Then
For i = 1 To UBound(FileNamesList)
Cells(i + 1, 1).Formula = FileNamesList(i)
Next i
SigString = FileNamesList(3)
If Dir(SigString) <> "" Then
Signature = GetBoiler(SigString)
Else
Signature = ""
End If
End If
Checking if an Array is Empty
Now you might be wondering, what's this IsArrayEmpty()
function we used? Well, VBA doesn't have a built-in function to check for empty arrays, so we need to create our own.
Here's the IsArrayEmpty()
function that you can use in your code:
Function IsArrayEmpty(arr As Variant) As Boolean
On Error Resume Next
IsArrayEmpty = (UBound(arr) < LBound(arr))
End Function
This function checks if the upper bound of the array is less than the lower bound, which indicates that the array is empty.
Tying it All Together
To summarize, here's what you need to do to check for empty arrays in your VBA Macro:
Add the
IsArrayEmpty()
function to your code.Check if the array is empty using
If Not IsArrayEmpty(FileNamesList)
.If the array is not empty, proceed with your code as usual.
Conclusion
With the modifications we discussed, you can now check for empty arrays in your VBA Macro and avoid errors. Remember to always check if an array is empty before performing any operations on it, so you can handle such scenarios gracefully.
We hope this guide helps you solve your problem. If you have any further questions or need more assistance, feel free to reach out. Happy coding! 😊👍