Hash Table/Associative Array in VBA
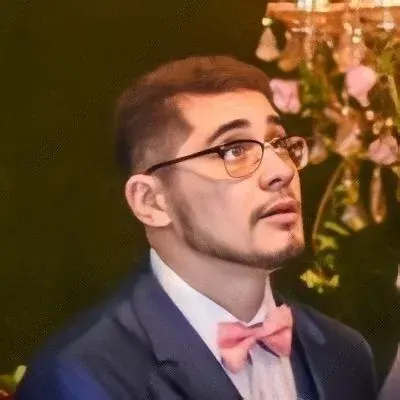
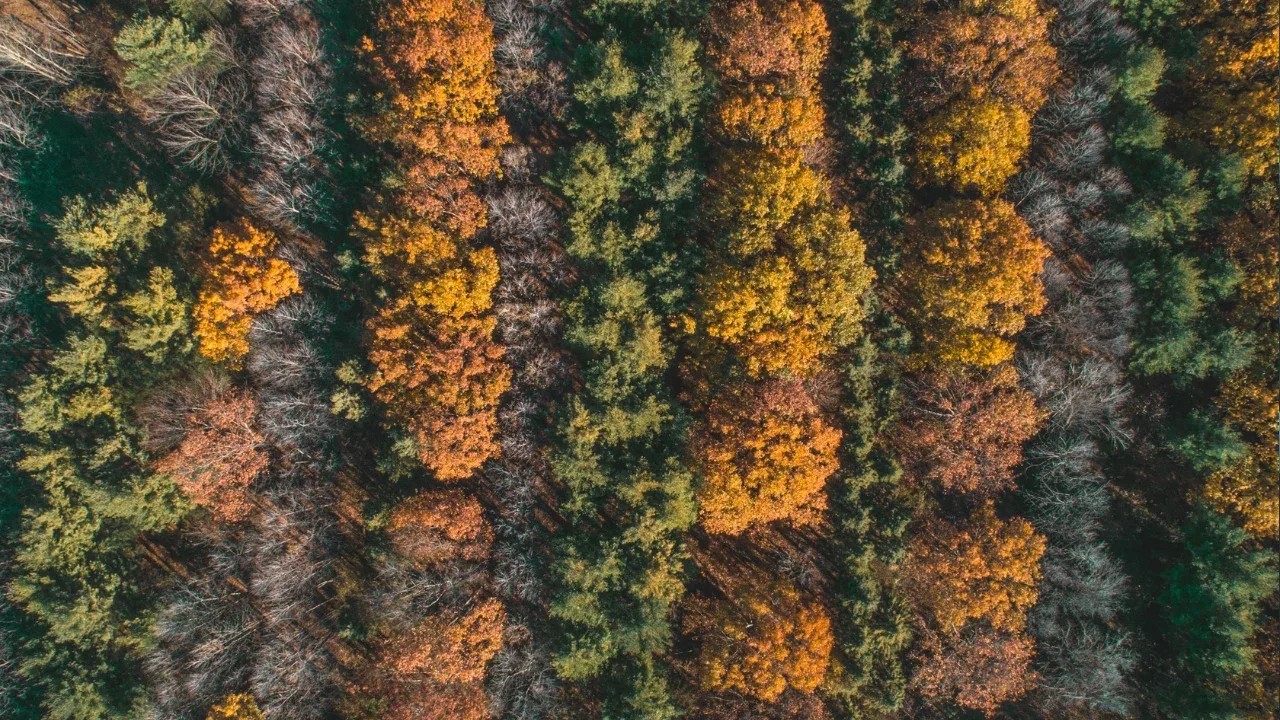
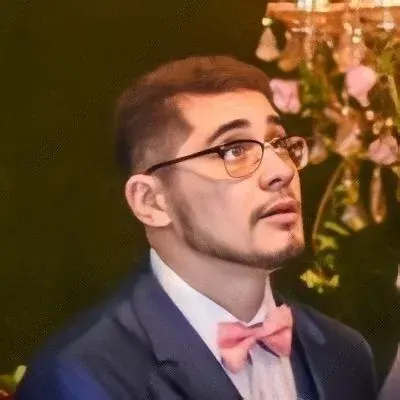
📝 The Ultimate Guide to Hash Tables/Associative Arrays in VBA 🗂
Hello, tech enthusiasts and coding wizards! 😄
Today, we are diving into the fascinating world of Hash Tables/Associative Arrays in VBA! 📚
The Mystery of Hash Tables/Associative Arrays in VBA
So, you're looking to create a Hash Table or Associative Array in VBA, but struggling to find the right documentation. 😔 Fear not, my friend! We're here to shed light on this topic and provide you with easy solutions. Let's get started! 🚀
Understanding the Basics
First things first, let's clear up any confusion. In VBA, there isn't a built-in data structure called Hash Table or Associative Array, like you might find in other programming languages. However, fear not, as we can replicate the functionality of these data structures in VBA. 🛠️
Creating a Hash Table in VBA
To create a Hash Table, we can make use of the Scripting.Dictionary object, which acts as a key-value pair collection. It allows you to store and retrieve values efficiently using unique keys. 🗝️ Here's a simple example to get you started:
Dim myHashTable As Object
Set myHashTable = CreateObject("Scripting.Dictionary")
' Adding items to the Hash Table
myHashTable.Add "Apple", "Red"
myHashTable.Add "Banana", "Yellow"
myHashTable.Add "Grapes", "Green"
' Accessing items in the Hash Table
MsgBox myHashTable("Apple") ' Output: Red
MsgBox myHashTable("Grapes") ' Output: Green
In the code snippet above, we create a new instance of the Scripting.Dictionary object and add items to it using the Add method. We can then access the values using unique keys using the MsgBox function.
Implementing an Associative Array in VBA
For an Associative Array, we can use the same Scripting.Dictionary object. Its key-value pair structure allows us to associate data elements, similar to an Associative Array in other programming languages. Let's take a look at an example:
Dim myAssociativeArray As Object
Set myAssociativeArray = CreateObject("Scripting.Dictionary")
' Adding items to the Associative Array
myAssociativeArray.Add "John", "Manager"
myAssociativeArray.Add "Lisa", "Developer"
myAssociativeArray.Add "Mike", "Designer"
' Accessing items in the Associative Array
MsgBox myAssociativeArray("John") ' Output: Manager
MsgBox myAssociativeArray("Mike") ' Output: Designer
Here, we create a new instance of the Scripting.Dictionary object and populate it with key-value pairs. We can then retrieve the values associated with each key using the MsgBox function.
Conclusion
And there you have it! While VBA doesn't have built-in Hash Tables or Associative Arrays, we can leverage the power of the Scripting.Dictionary object to achieve the same functionality. 💪
Now, it's your turn to put your newfound knowledge into action! Try implementing Hash Tables or Associative Arrays in your VBA projects and see the magic unfold. ✨
Have any questions or suggestions? Share them in the comments below! We'd love to hear from you. 😊
Happy coding! 🎉