Determining whether an object is a member of a collection in VBA
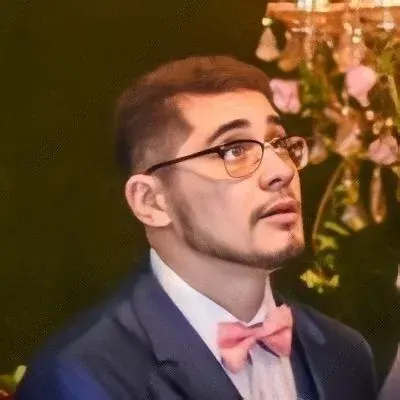
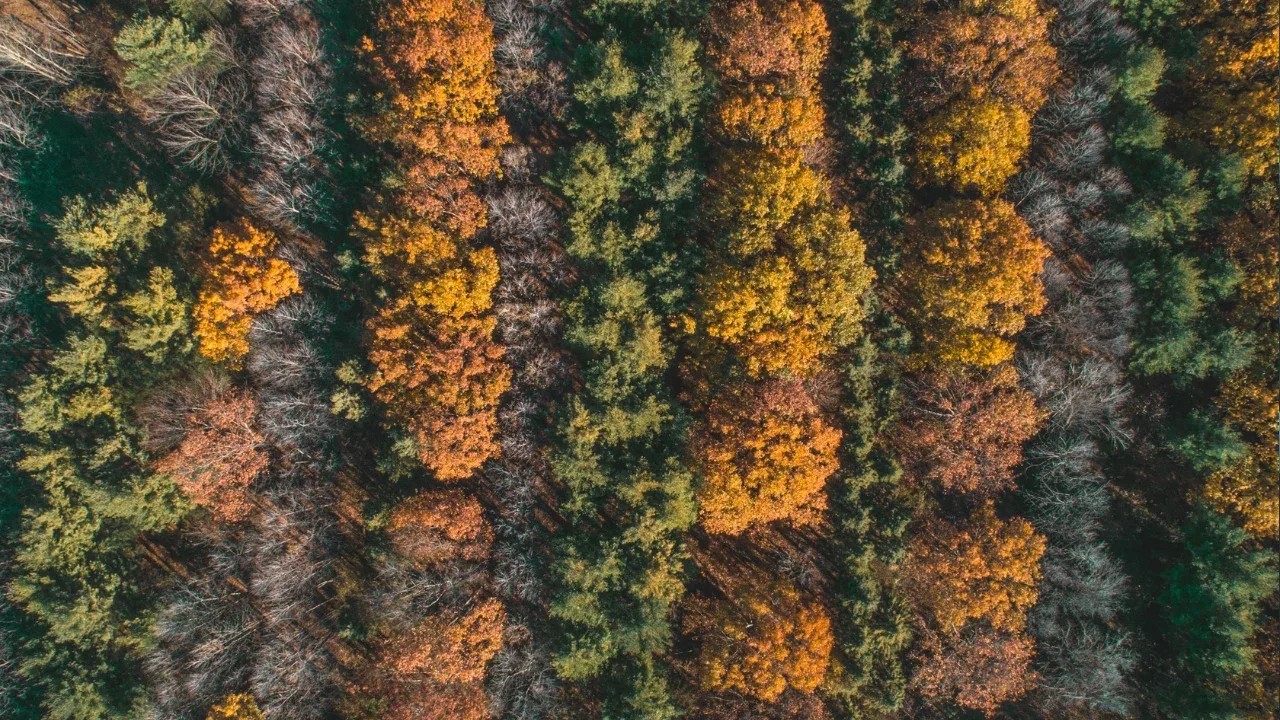
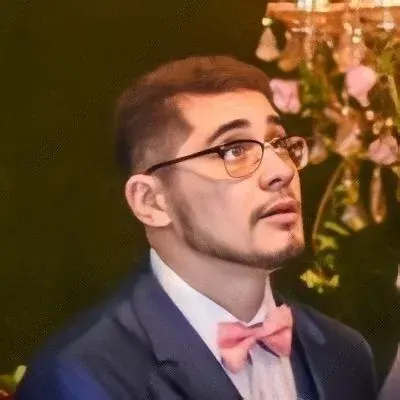
š Title: Mastering VBA Collections: How to Determine If an Object is a Member šµļøāāļøš
š Introduction:
So you're dabbling in the mystical world of VBA and find yourself stuck with the burning question: "How can I tell if an object is a member of a collection in VBA?" Fear not! š āāļø In this blog post, we will go on an adventure together to unravel this mystery and equip you with easy-to-implement solutions. š
š” Understanding the Problem:
Picture yourself standing at the entrance of a secret club, wondering if your name is on the guest list. In VBA, it's not much different. Our objective is to determine whether a specific object, such as a table definition, is a member of a collection, in this case, the TableDefs
collection. šŖš
šÆ Solution 1: Iteration Is Key š:
One way to solve this conundrum is by iterating through the collection and comparing each element to the object you're searching for. Let's get down to code:
Function IsMember(objectToFind As Object, collectionToSearch As Collection) As Boolean
Dim member As Object
For Each member In collectionToSearch
If member Is objectToFind Then
IsMember = True
Exit Function
End If
Next member
IsMember = False
End Function
In the code snippet above, we defined a function called IsMember
, which takes two parameters: the objectToFind
and the collectionToSearch
. We iterate through each member of the collection using a For Each
loop. If we find a match using the Is
keyword, we return True
and exit the function. If no match is found, we return False
.
Now, you can simply use the IsMember
function to check if a table definition is a member of the TableDefs
collection:
Dim myTable As TableDef
Dim isMember As Boolean
Set myTable = CurrentDb.TableDefs("YourTableName")
isMember = IsMember(myTable, CurrentDb.TableDefs)
š Solution 2: Call It by Its Name š¤:
If you prefer a more streamlined approach, VBA has your back! You can directly access a specific member of a collection by its name, without the need for iteration:
Sub Main()
Dim myTable As TableDef
Set myTable = CurrentDb.TableDefs("YourTableName")
On Error Resume Next
Err.Clear
isMember = Not (myTable Is Nothing)
On Error GoTo 0
If isMember Then
' Success! Your table definition is a proud member of the collection.
MsgBox "Yes! The table definition is a member!"
Else
' Alas! Your table definition is lonely and not part of the collection.
MsgBox "No! The table definition is not a member!"
End If
End Sub
In the example above, we try to set the myTable
variable equal to the desired table definition using its name. Then, we check if myTable
is Nothing
, indicating that the table definition does not exist in the collection.
š Conclusion:
Congratulations, my coding companion! š Now you possess the power to determine whether an object is a member of a collection in VBA. With these two simple solutions in your arsenal, no collection will be able to hide its secrets from you! šŖ
So go forth and unravel more VBA mysteries! š And don't forget to share your newfound knowledge with fellow coders in the comments below! Let's keep the conversation going! š¬š”