Call a Subroutine from a different Module in VBA
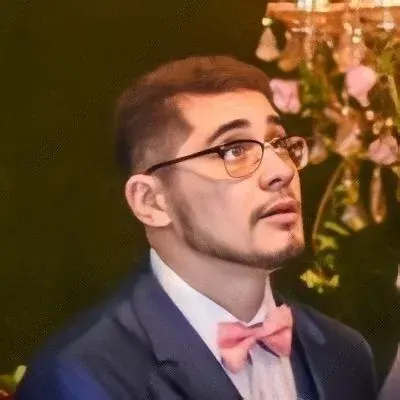
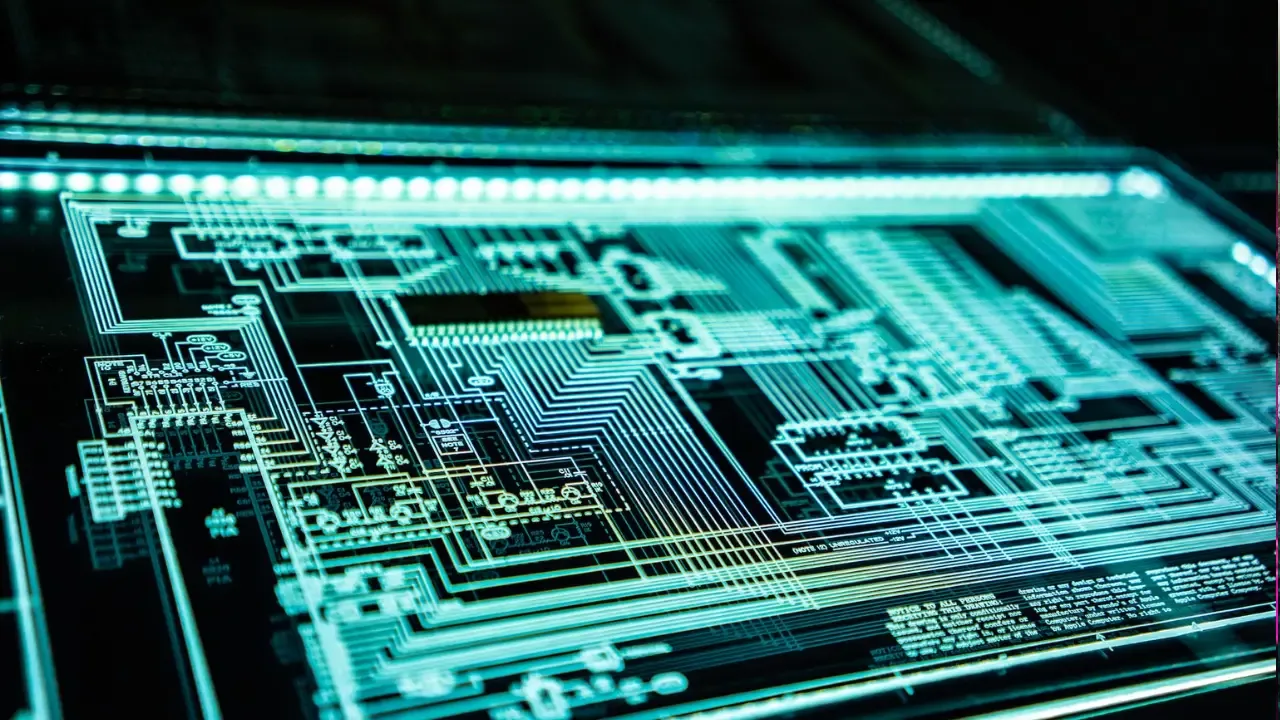
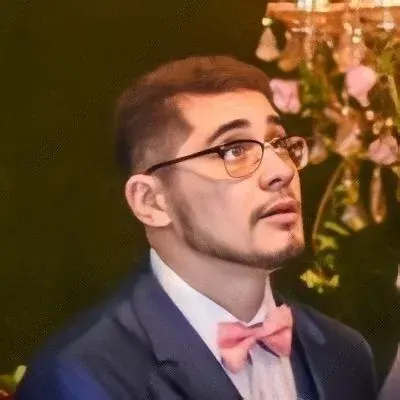
A Beginner's Guide to Calling a Subroutine from a Different Module in VBA
Are you struggling with calling a subroutine from a different module in VBA? 🤔 Don't worry, you're not alone! Many VBA beginners encounter this issue when trying to create a modular and organized code structure. In this blog post, we'll walk you through the common issues and provide easy solutions to help you conquer this problem. 💪
The Scenario
Let's imagine you have two modules, Module1
and Module2
, and you want to call a subroutine called IDLE
in Module2
from another subroutine called MAIN
in Module1
. Here's the code snippet for reference:
Sub MAIN()
Call IDLE
End Sub
Here's what you need to do to make this work:
Method 1: Using the Call
Statement
The simplest way to call a subroutine from a different module is by using the Call
statement followed by the name of the subroutine. Here's how you can modify your code:
Sub MAIN()
Call Module2.IDLE
End Sub
By prefixing Module2
(the name of the module where IDLE
is located) followed by a dot and then the name of the subroutine, you are effectively calling the IDLE
subroutine from Module2
.
Method 2: Using the Dot Notation
Alternatively, you can use the dot notation to call the subroutine directly. Here's an example:
Sub MAIN()
Module2.IDLE
End Sub
As you can see, you don't need to use the Call
statement when using the dot notation. This method can be more concise and is often preferred by experienced VBA programmers.
Common Issues and Troubleshooting Tips
Issue 1: "Expected Sub, Function, or Property" Error
If you encounter the "Expected Sub, Function, or Property" error message when calling a subroutine from a different module, it usually means that either the subroutine doesn't exist or there is a typo in the subroutine name. Double-check the names of your modules and subroutines to ensure they match exactly.
Issue 2: Difficulty Calling Nested Subroutines
In some cases, you may need to call a subroutine that is nested inside another subroutine within a different module. To do this, you can use the dot notation repeatedly to access the outer and inner subroutines. Here's an example:
Sub MAIN()
Module3.OuterSub.InnerSub
End Sub
Just make sure you have the correct module names and the correct hierarchy of nested subroutines.
Issue 3: Module Visibility
If you're still having trouble calling a subroutine from a different module, it could be due to module visibility. In VBA, modules have different levels of visibility (e.g., Public
, Private
, or Friend
). Ensure that both the calling and called subroutines have the appropriate visibility level for your specific requirements.
Take Your VBA Skills to the Next Level! 🚀
Now that you know how to call a subroutine from a different module in VBA, it's time to put this knowledge into practice! Try implementing this technique in your own VBA projects and see how it improves the modularity and organization of your code. 🎉
Remember, the more you practice, the better you'll get. So go ahead and start experimenting with different VBA subroutines and modules!
If you found this blog post helpful, feel free to share it with your fellow VBA enthusiasts. And don't forget to leave a comment below if you have any questions or additional tips to share. We love hearing from our readers! 😊