What is the question mark for in a Typescript parameter name
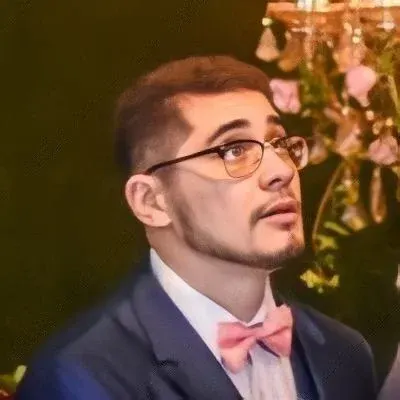
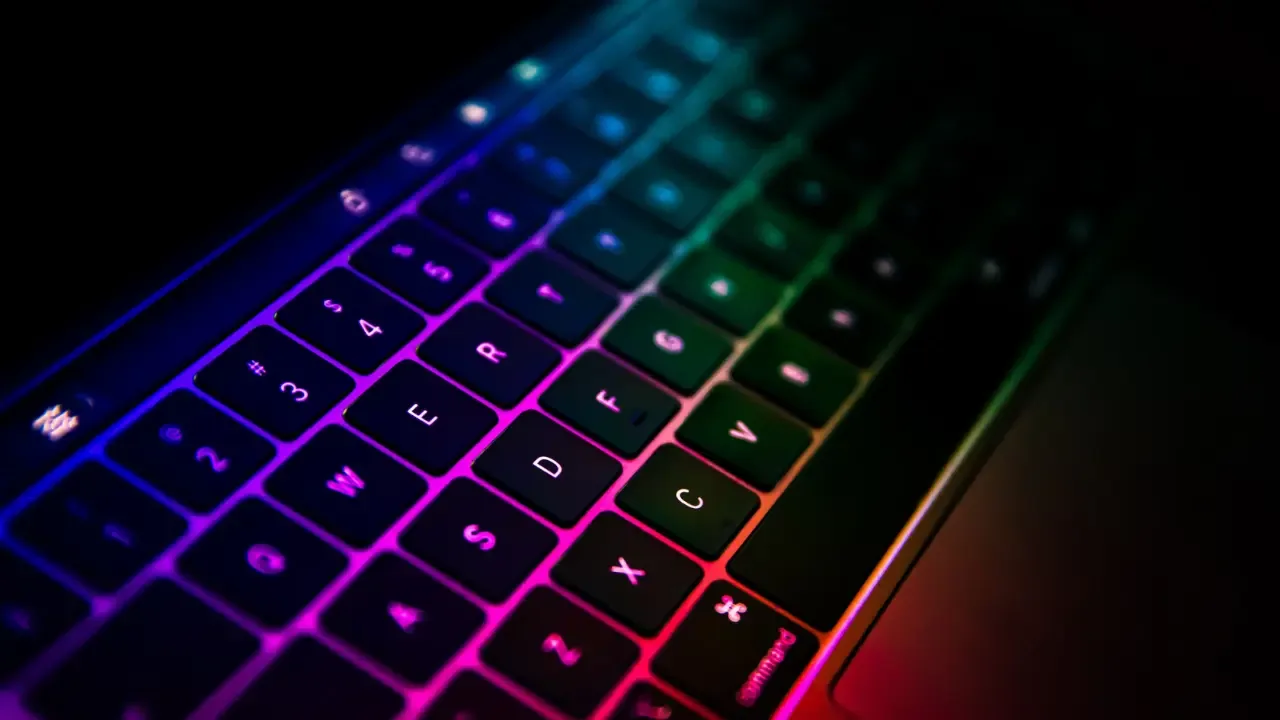
The Mystery of the Question Mark in Typescript Parameter Names 😕
Are you curious about the purpose of the question mark symbol "?" found in Typescript parameter names? 🤔 Don't worry, you're not alone! This seemingly mysterious symbol serves a specific purpose and understanding it can greatly improve your Typescript development skills. Let's dive in and unravel the mystery! 🔍
The Context:
To begin, let's examine a code snippet that will shed some light on the topic:
export class Thread {
id: string;
lastMessage: Message;
name: string;
avatarSrc: string;
constructor(id?: string,
name?: string,
avatarSrc?: string) {
this.id = id || uuid();
this.name = name;
this.avatarSrc = avatarSrc;
}
}
In the constructor
function of the Thread
class, you might notice the presence of the question mark symbol next to the parameter names id
, name
, and avatarSrc
. But what does it mean? Let's find out! 🚀
The Question Mark:
The question mark symbol in Typescript parameter names denotes that the corresponding parameter is optional. This means that the parameter does not have to be provided with a value when the constructor is called. By using the question mark, we are indicating that a value is not required for that parameter.
In our example, the id
, name
, and avatarSrc
parameters are all optional. When the constructor
function is invoked, you can choose whether or not to provide a value for these parameters. If no value is provided, a default value will be assigned. For example, the id
parameter defaults to a generated UUID using the uuid()
function.
Benefits of Optional Parameters:
Using optional parameters can bring several benefits to your Typescript code. Here are a few advantages:
Flexibility: Optional parameters allow you to have more flexibility in creating instances of a class. You can choose to provide specific values for certain parameters while leaving others undefined.
Readability: By marking parameters as optional, you make it clear to other developers that those values are not required, improving code readability and reducing confusion.
Ease of Maintenance: If you ever need to modify or extend a class, using optional parameters makes it easier to add new functionality without breaking existing code. You won't have to refactor constructors throughout your codebase to accommodate new parameters.
A Practical Example:
Let's demonstrate the use of optional parameters with a practical example. Suppose we want to create a Person
class that represents individuals. The Person
class has three optional parameters: firstName
, lastName
, and age
. Here's how the class could be implemented:
export class Person {
constructor(public firstName?: string,
public lastName?: string,
public age?: number) {
}
introduce(): string {
if (this.firstName && this.lastName) {
return `Hello, my name is ${this.firstName} ${this.lastName}!`;
} else {
return 'Hello, nice to meet you!';
}
}
}
By making the firstName
, lastName
, and age
parameters optional, we can now create Person
objects with different levels of detail:
const john = new Person('John', 'Doe');
const jane = new Person('Jane', 'Smith', 25);
const stranger = new Person();
console.log(john.introduce()); // Output: Hello, my name is John Doe!
console.log(jane.introduce()); // Output: Hello, my name is Jane Smith!
console.log(stranger.introduce()); // Output: Hello, nice to meet you!
The Call-to-Action:
Now that you've unraveled the mystery behind the question mark in Typescript parameter names, why not put this newfound knowledge into practice? Start incorporating optional parameters in your own code to enhance flexibility and readability. 🙌
Share your experiences and any cool tips you discover along the way in the comments section below. Let's learn and grow together! 👇
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
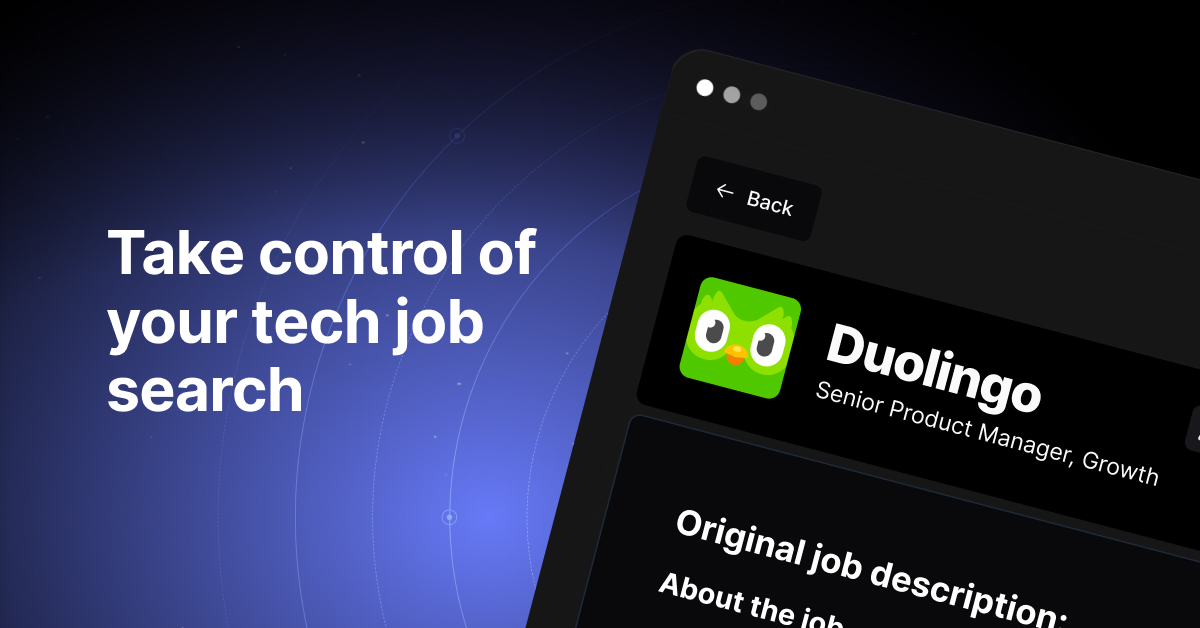