What does the `is` keyword do in typescript?
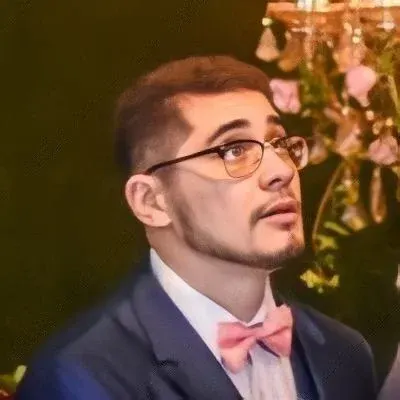
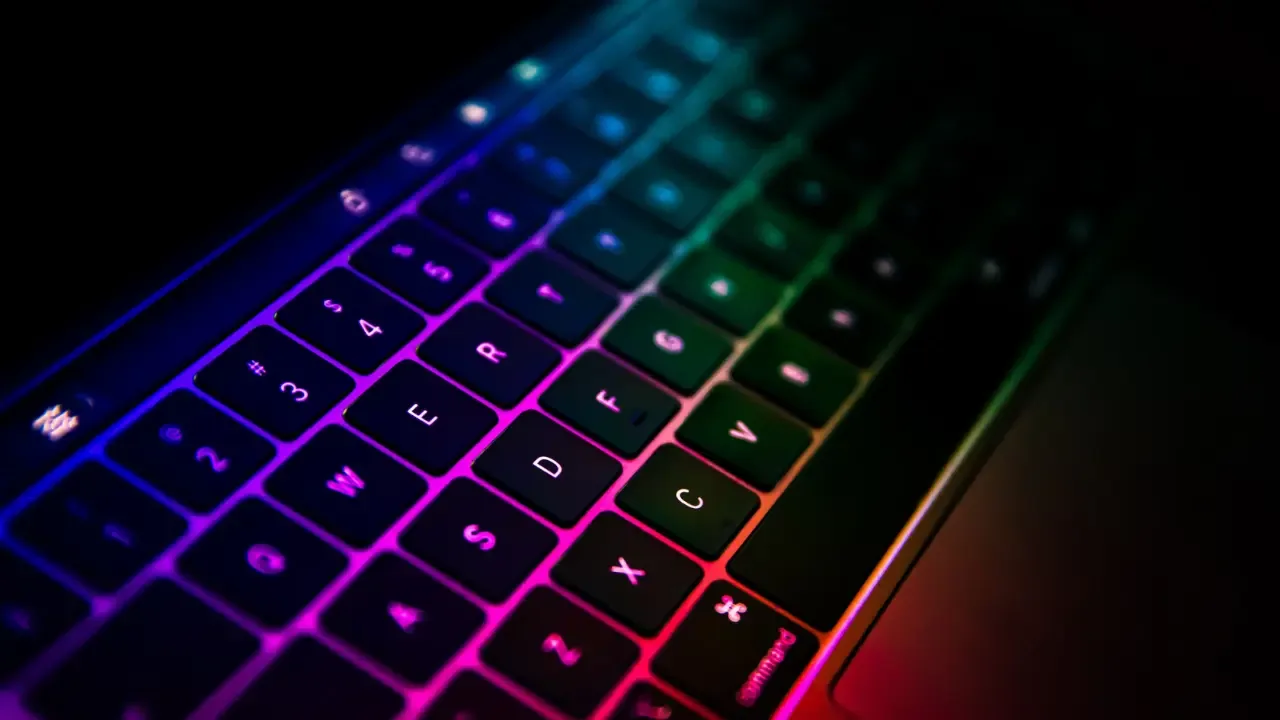
Understanding the is
Keyword in TypeScript: A Simple Explanation 🤔💡
Have you ever come across the mysterious is
keyword in TypeScript and found yourself scratching your head in confusion? You're not alone! 🤷♂️
In this blog post, we'll demystify the is
keyword and explore its purpose and usage. By the end, you'll have a crystal-clear understanding of how to use it in your code. Let's dive in! 🏊♀️
What is the is
Keyword? 🤔
The is
keyword in TypeScript is used for type guards. It allows you to narrow down the type of a variable within a conditional statement. In simpler terms, it helps you check if a value is of a specific type. 🦉
In the code snippet you provided:
export function foo(arg: string): arg is MyType {
return ...
}
The is
keyword is used to create a type guard for the variable arg
. It asserts that arg
is of type MyType
. The function returns true
if the condition is met, indicating that arg
is indeed of the specified type.
On the other hand, if the condition evaluates to false
, it means arg
is not of the expected type, and the function returns false
. Simple, right? 😉
Why Use the is
Keyword? 🧐
TypeScript is all about static types and type safety. By utilizing the is
keyword, you can ensure that your code handles different types in a more robust and predictable way.
The is
keyword becomes particularly useful when dealing with complex codebases or third-party libraries that expose multiple types for a given variable. It allows you to perform type-specific actions based on runtime inspections.
Example Usage ✨
To further clarify the usage of the is
keyword, let's look at an example. Suppose we have the following types:
type Square = {
sideLength: number;
};
type Circle = {
radius: number;
};
Now, we want to create a function that calculates the area of either a Square
or a Circle
. We can leverage the is
keyword to achieve this:
function calculateArea(shape: Square | Circle): number {
if ('sideLength' in shape) {
// We can safely assume shape is of type Square here
return shape.sideLength ** 2;
}
if ('radius' in shape) {
// We can safely assume shape is of type Circle here
return Math.PI * shape.radius ** 2;
}
// Fall back to a default value
return 0;
}
In this example, we use the in
operator to check if shape
has the property sideLength
or radius
. If it has sideLength
, TypeScript understands that shape
is a Square
and performs the necessary calculations. Similarly, if it has radius
, TypeScript treats shape
as a Circle
.
Wrapping Up 🎁
Congratulations! You've just unlocked the secrets of the is
keyword in TypeScript. 🎉🔓
By using the is
keyword, you can create type guards and confidently handle different types within your code. Remember, it's an invaluable tool when working with complex code or third-party libraries.
Now it's your turn to put your newfound knowledge into practice! Start using the is
keyword to make your TypeScript code more robust and type-safe. If you have any questions or interesting use cases, feel free to share them in the comments below. Let's learn together! 🚀💪
📢 Call-to-Action: Do you want to level up your TypeScript skills? Check out our comprehensive TypeScript tutorial series [link to your tutorial series], where you'll find more in-depth explanations and practical examples to enhance your knowledge.
Happy coding! 👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
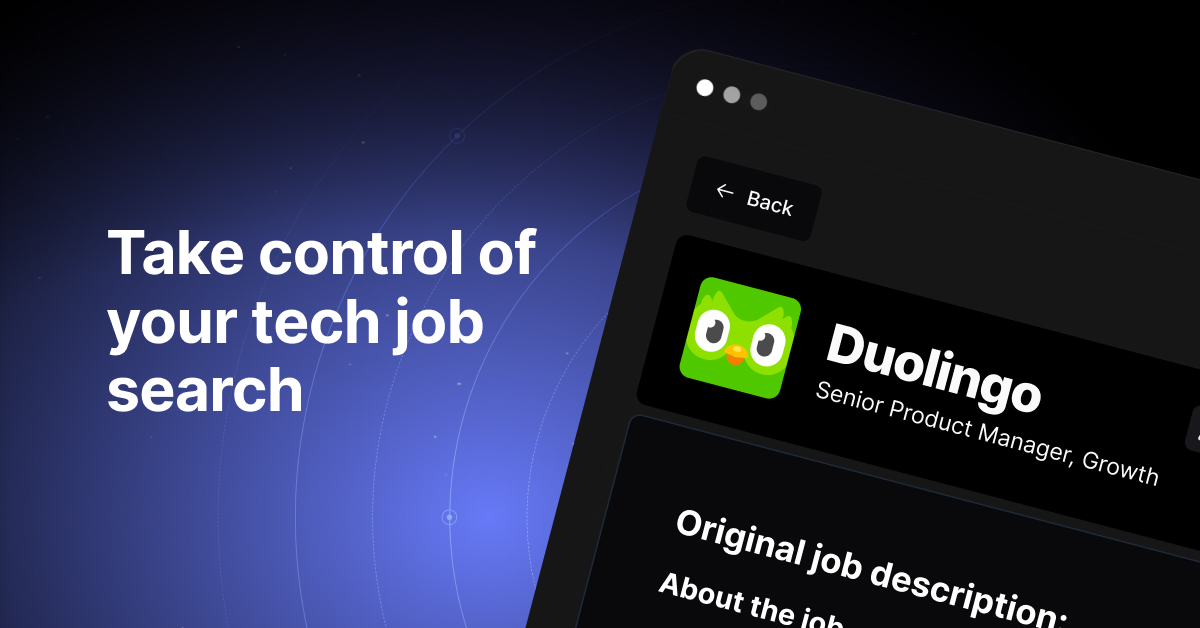