Using eslint with typescript - Unable to resolve path to module
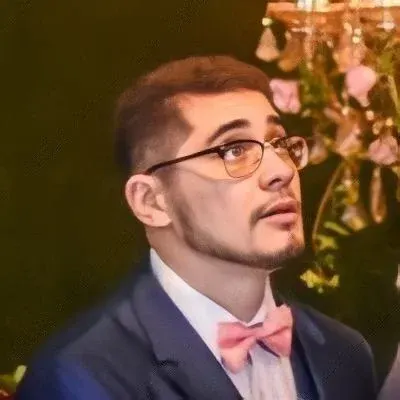
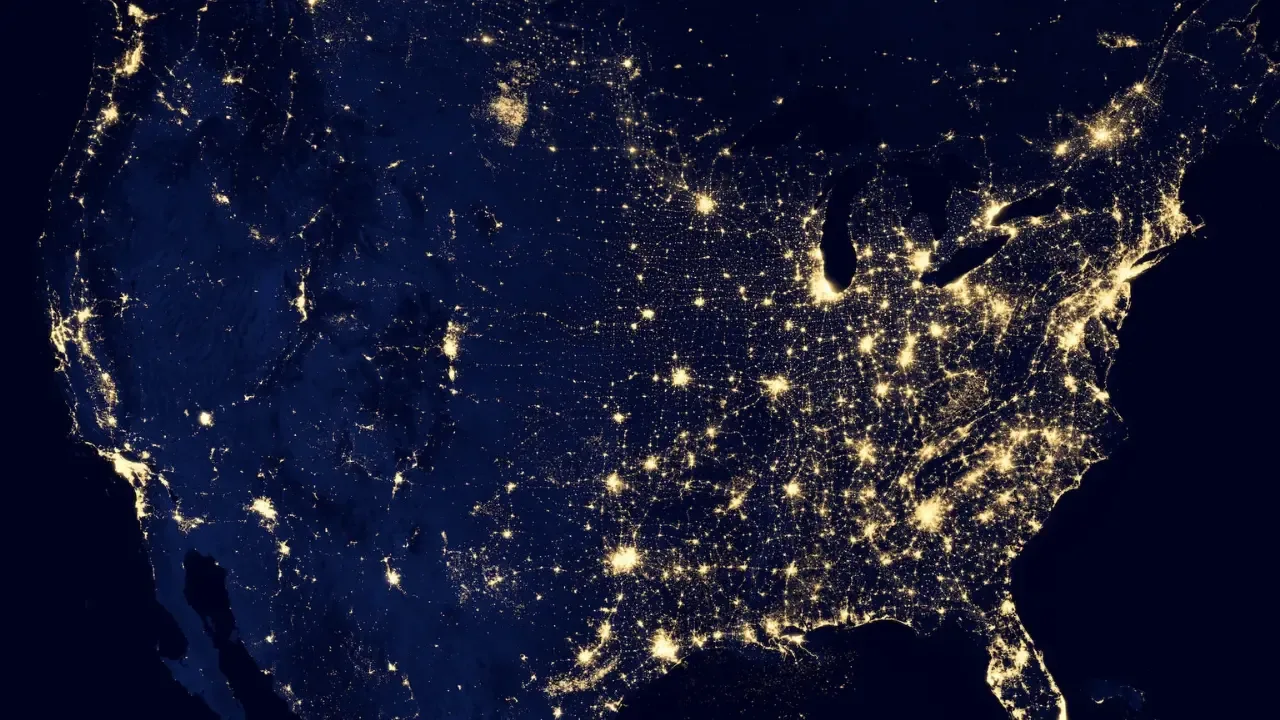
Using eslint with TypeScript: Unable to Resolve Path to Module 🚧
Have you ever encountered the frustrating TypeScript error "Unable to resolve path to module"? 🤔 It's a common issue when using eslint with TypeScript and can cause headaches for developers trying to import modules.
In this blog post, we'll dive into common reasons why this error occurs, provide easy solutions to resolve it, and share how to configure eslint to correctly resolve modules with the ".ts" extension. Let's get started! 💪
Understanding the Problem 📚
The error message "Unable to resolve path to module" usually occurs when eslint cannot locate the module you're trying to import. This can happen if eslint is configured to resolve modules with a ".js" extension instead of the desired ".ts" extension.
In your specific case, you're trying to import the "app" module from "./app.ts" in your "app.spec.ts" file. However, eslint is expecting a ".js" file, which leads to the error.
Solution #1: Compile the TypeScript File 🛠️
One quick solution is to compile the ".ts" file containing the module you want to import into a ".js" file. Once compiled, eslint will be able to resolve the module without any issues.
To compile a TypeScript file into a JavaScript file, you can use a tool like Babel or run the TypeScript compiler command (tsc
) in your project's root directory:
tsc app.ts
By executing this command, TypeScript will compile the "app.ts" file into a corresponding "app.js" file. After the compilation, the error should go away.
However, let's explore another solution that allows eslint to resolve modules using the ".ts" extension.
Solution #2: Configure eslint to Resolve ".ts" Modules 📝
To make eslint resolve modules with the ".ts" extension, you need to configure the parser options in your ".eslintrc" file.
Make sure you have the following configuration in your ".eslintrc" file:
{
"parser": "@typescript-eslint/parser",
"parserOptions": {
"project": "./tsconfig.json"
}
}
By specifying "parser": "@typescript-eslint/parser"
, you're telling eslint to use the TypeScript parser for analyzing and resolving TypeScript files.
The "parserOptions"
section, with the "project": "./tsconfig.json"
configuration, helps eslint understand the project's settings defined in the "tsconfig.json" file. It includes important information about module resolution, allowing eslint to resolve ".ts" modules correctly.
Putting It All Together 👨💻
Now, let's review the code snippet from your "app.ts" file to ensure we have a complete understanding of the context:
import bodyParser from 'body-parser';
import express from 'express';
import graphqlHTTP from 'express-graphql';
import { buildSchema } from 'graphql';
const app = express();
const schema = buildSchema(`
type Query {
hello: String
}
`);
const root = { hello: () => 'Hello world!' };
app.use(bodyParser());
app.use('/graphql', graphqlHTTP({
schema,
rootValue: root,
graphiql: true,
}));
export default app;
Please note that your "app.ts" file seems to be correctly written, and the issue lies in eslint's module resolution. By following solution #2 and configuring eslint correctly, you should be able to use the import statement without any issues.
Conclusion and Call to Action ✅
We hope that this blog post has provided valuable insights into resolving the "Unable to resolve path to module" error when using eslint with TypeScript. Remember, you can either compile the TypeScript file or configure eslint to resolve modules using the ".ts" extension.
If you found this post helpful, share it with your fellow developers who might be facing the same issue. Let's spread the word and save developers from unnecessary troubleshooting headaches! 🚀
If you have any questions or need further assistance, leave a comment below. We'd love to help you out! 😊
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
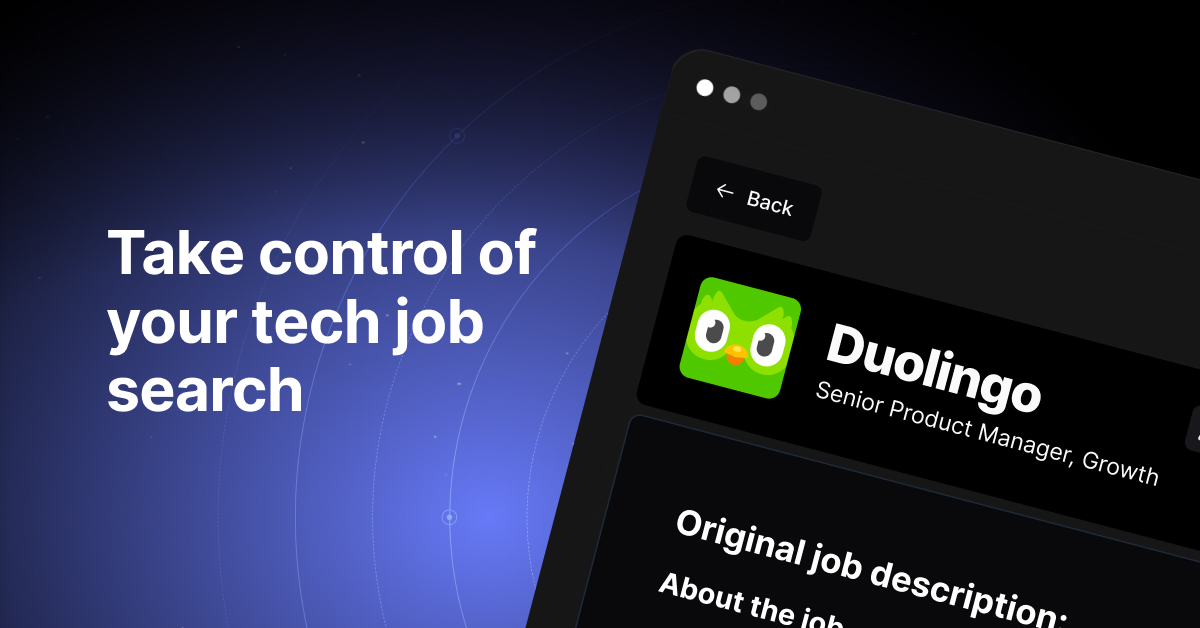