Typescript: TS7006: Parameter "xxx" implicitly has an "any" type
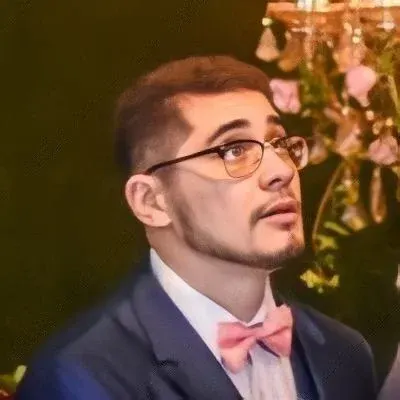
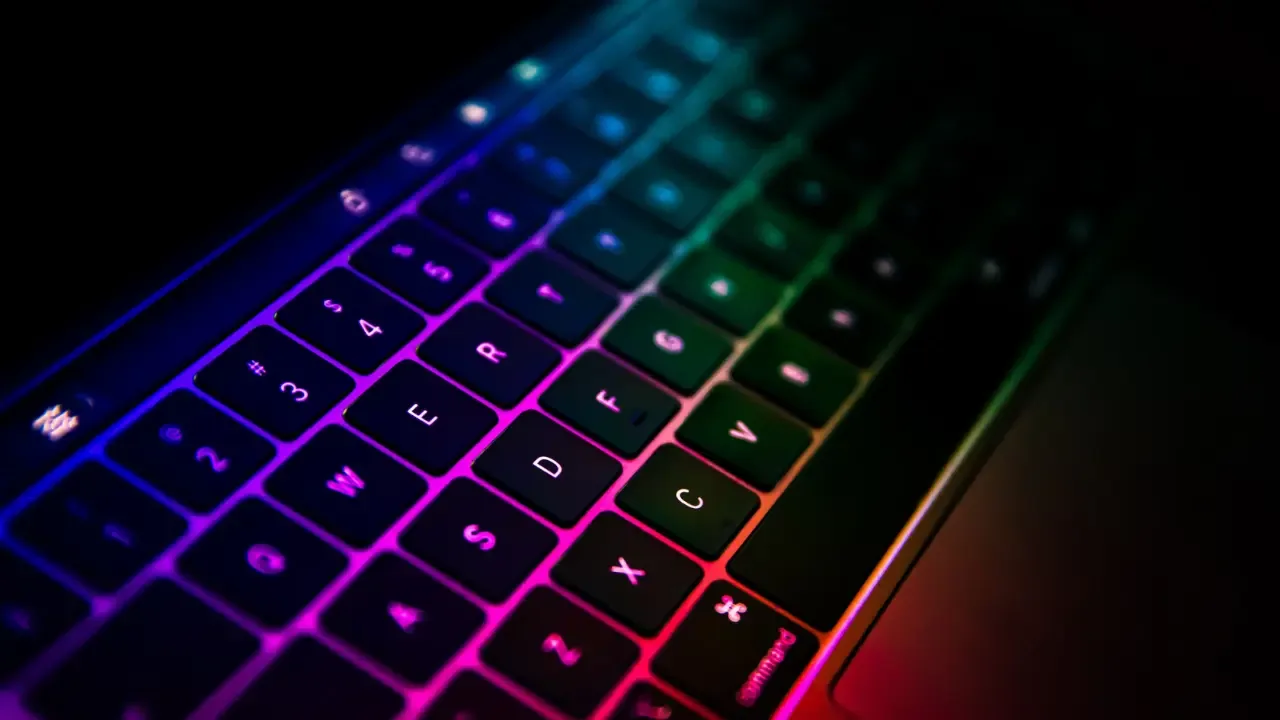
Solving the TS7006 Error: Parameter 'xxx' implicitly has an 'any' type
š Hey there tech enthusiasts! Are you working with TypeScript and encountered the dreaded TS7006 error: "Parameter 'xxx' implicitly has an 'any' type"? Don't worry, we've got your back! In this blog post, we'll walk you through common issues related to this error and provide you with easy solutions.
Understanding the Error
Let's start by understanding the context around this error. In our scenario, we have a UserRouter that fetches user data from a JSON file called data.json
. When building the app, TypeScript throws an error specifically in the UserRouter.ts
file, pointing us to line [30]
:
import {Router, Request, Response, NextFunction} from 'express';
const Users = require('../data');
export class UserRouter {
router: Router;
constructor() {
// ...
}
/**
* GET one User by id
*/
public getOne(req: Request, res: Response, _next: NextFunction) {
let query = parseInt(req.params.id);
/* [30] -> */ let user = Users.find(user => user.id === query);
// ...
}
}
const userRouter = new UserRouter().router;
export default userRouter;
The error itself states:
ERROR in ...../UserRouter.ts
(30,27): error TS7006: Parameter 'user' implicitly has an 'any' type.
From this error message, we can deduce that the issue lies with the user
parameter in the Users.find()
method. TypeScript infers its type as any
because it cannot determine the correct type based on the provided information.
The Solution
To fix this error, we need to help TypeScript understand the correct type of the user
parameter. In our case, since we're fetching user data from a JSON file, we can define a type for the user
object based on the structure of our data in data.json
.
Let's assume our user objects have the following structure:
interface User {
id: number;
name: string;
aliases: string[];
occupation: string;
gender: string;
height: {
ft: number;
in: number;
};
hair: string;
eyes: string;
powers: string[];
}
Now, we can use this User
interface to explicitly type the user
parameter in the find()
method like so:
let user = Users.find((user: User) => user.id === query);
By providing the correct type information, TypeScript will no longer infer the any
type for the user
parameter, and the error TS7006 will vanish into thin air!
Take Action, Keep Coding!
š Amazing job! You've successfully resolved the TS7006 error. Remember, implicit 'any' types can lead to potential bugs and make code harder to maintain, so it's always better to be explicit about the types.
Now it's your turn! Take what you've learned and apply it to your own TypeScript projects. If you encounter any other TypeScript-related issues or have any questions, feel free to leave a comment below. We'd love to help you out!
Happy typing, folks! š©āš»šØāš»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
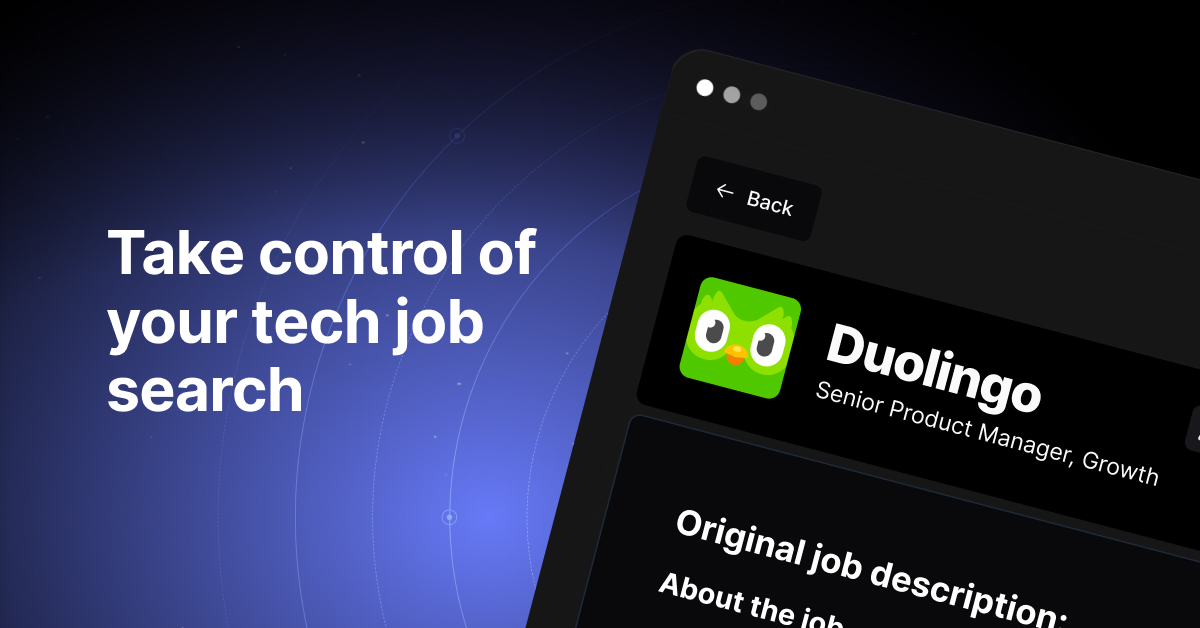