typescript interface require one of two properties to exist
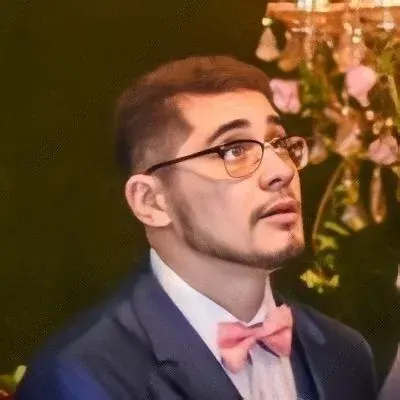
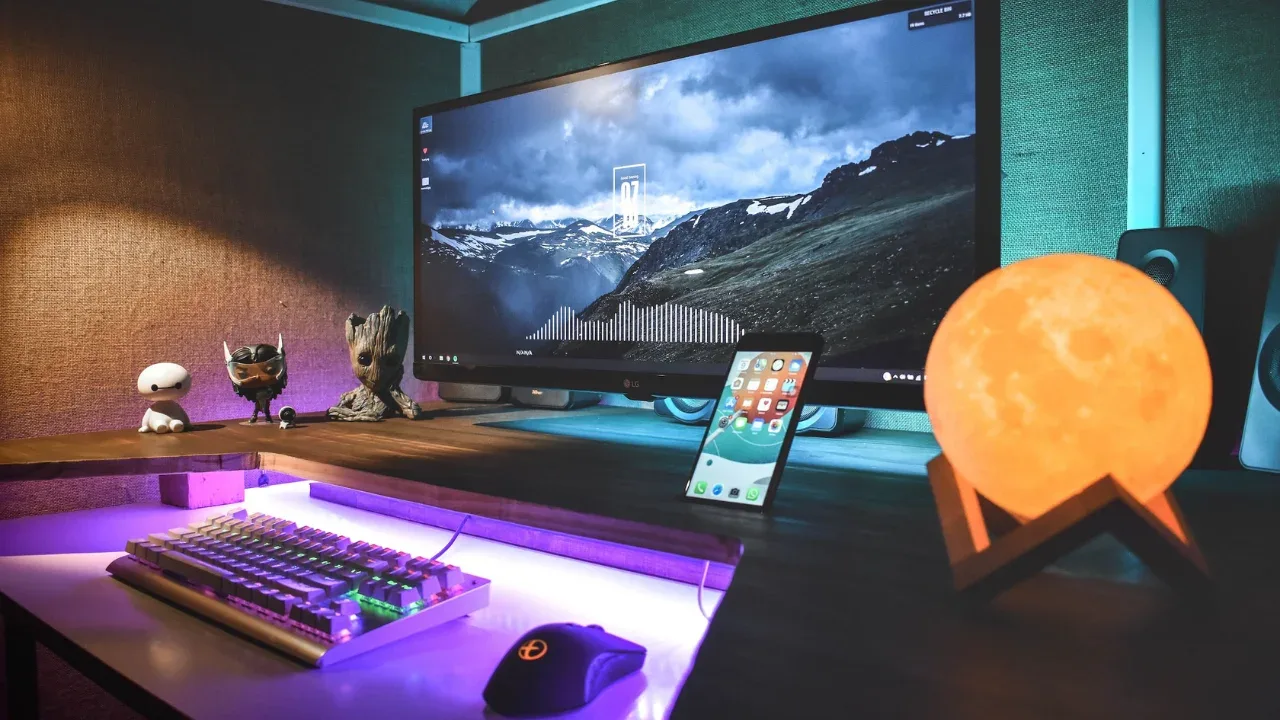
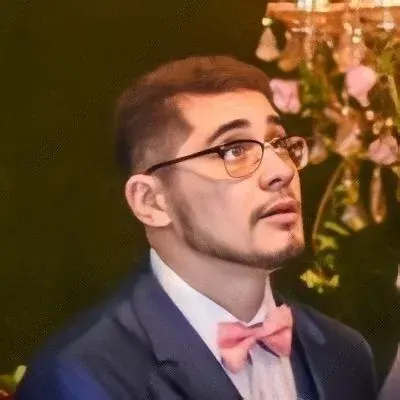
TypeScript Interface: Requiring One of Two Properties to Exist
ššØāš»š
Are you struggling with creating a TypeScript interface that requires one of two properties to exist? š¤ Don't worry, you're not alone! It's a common issue that developers face when designing interfaces that offer flexibility without sacrificing consistency. In this blog post, we will address this specific problem and provide you with easy solutions. Let's dive in! šŖ
The Interface Conundrum
The provided code snippet showcases an interface called MenuItem
which represents a menu item in an application. The properties title
and icon
are required, while component
and click
are optional.
export interface MenuItem {
title: string;
component?: any;
click?: any;
icon: string;
}
Now, let's explore the two questions at hand:
1ļøā£ Requiring Either component
or click
to be Set
If you want to ensure that either component
or click
is set, but not both, TypeScript allows you to achieve this by utilizing the exclusive OR (xor) operator.
export interface MenuItem {
title: string;
component?: any;
click?: any;
icon: string;
__exclusivity?: 'component' | 'click';
}
š The magic lies in the newly introduced __exclusivity
property. By creating this additional property, TypeScript ensures that either component
or click
is present, while the other property remains undefined.
Here's how this can be used:
const menuItem1: MenuItem = {
title: 'Example 1',
component: ExampleComponent,
icon: 'example-icon',
__exclusivity: 'component',
};
const menuItem2: MenuItem = {
title: 'Example 2',
click: handleClick,
icon: 'example-icon',
__exclusivity: 'click',
};
With this approach, you have the flexibility to require either component
or click
to be set, providing a clear and defined structure for your code.
2ļøā£ Requiring That Both Properties Can't be Set
In some cases, you may want to enforce a rule where both component
and click
cannot be set simultaneously. TypeScript's conditional types come to the rescue!
export interface MenuItem {
title: string;
component?: any;
click?: any;
icon: string;
__exclusivity?: 'component' extends keyof this ? never : 'click' extends keyof this ? never : 'component' | 'click';
}
š By leveraging conditional types, we can define the __exclusivity
property based on whether component
or click
is present. If component
exists, we make click
ineligible, and vice versa.
Here's how this can be used:
const menuItem1: MenuItem = {
title: 'Example 1',
component: ExampleComponent,
icon: 'example-icon',
};
// This will result in a TypeScript error, as both `component` and `click` are set simultaneously.
const menuItem2: MenuItem = {
title: 'Example 2',
component: ExampleComponent,
click: handleClick,
icon: 'example-icon',
};
With this solution, you can ensure that either component
or click
is set, never both.
Conclusion
Requiring one of two properties to exist in a TypeScript interface might initially seem challenging, but with the tips provided above, you can conquer this hurdle effortlessly. āØšŖ
Remember, TypeScript offers powerful features like exclusive OR
operations and conditional types that enable you to design precise and flexible interfaces.
Now it's your turn! Have you encountered this interface conundrum before? How did you solve it? Share your experiences and solutions in the comments below. Let's learn and grow together! š±š„š¬
Keep coding, keep exploring, and never stop creating amazing interfaces! šš©āš»
š Don't forget to subscribe to our newsletter for more TypeScript tips and tricks delivered straight to your inbox! š
#typescript #interface #typescriptinterface #webdevelopment #programming #codenewbie