Typescript interface default values
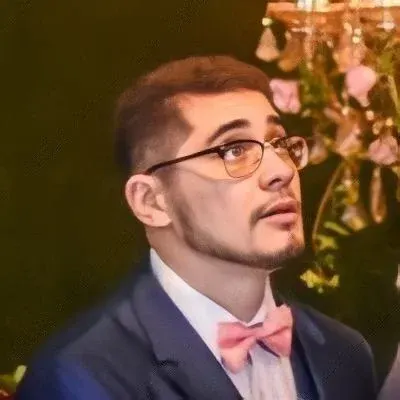
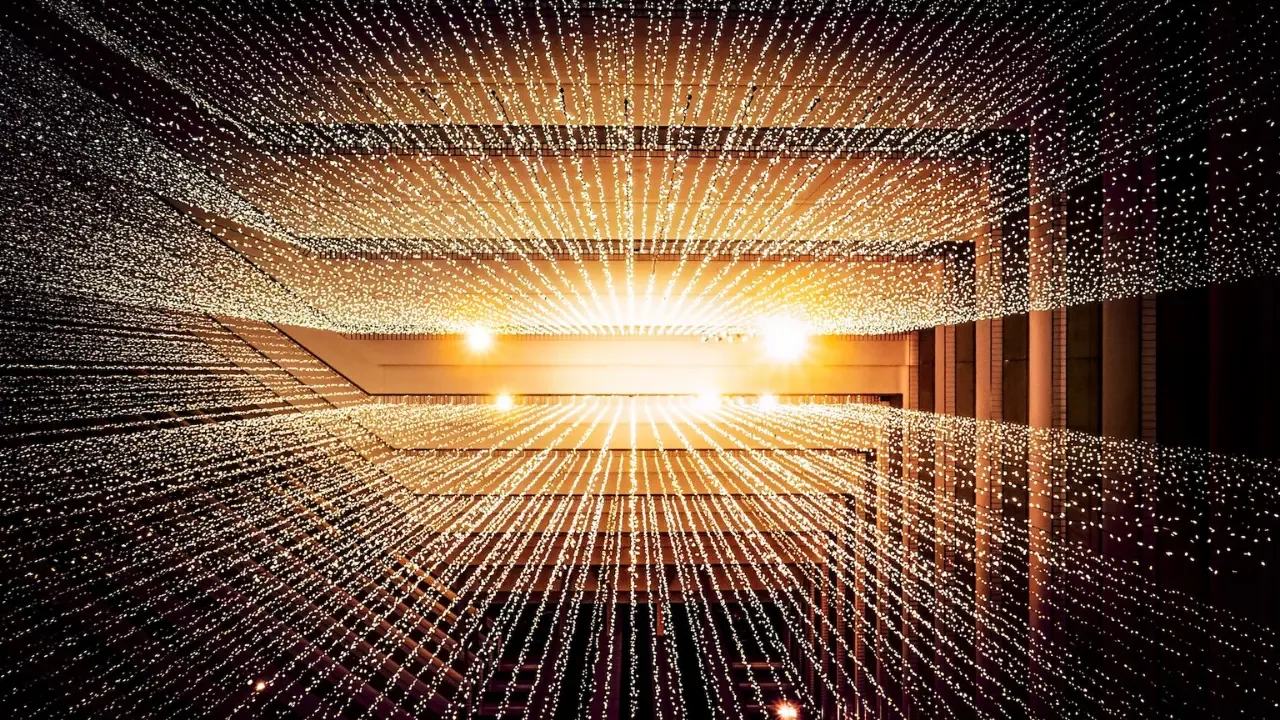
🚀 TypeScript Interface Default Values: Simplify Your Code 🚀
Have you ever found yourself declaring an interface in TypeScript, only to later assign values to its properties? How frustrating is it to have to specify default null values for each property, knowing that they will all be set to real values later on? 😫
Let me show you a simple trick to avoid this common issue and make your code more elegant. 🎩
The Problem
Let's start by understanding the problem at hand. 😮
Assume we have the following interface, IX
, defined in TypeScript:
interface IX {
a: string;
b: any;
c: AnotherType;
}
Then, we declare a variable, x
, of type IX
, and initialize all its properties:
let x: IX = {
a: 'abc',
b: null,
c: null
};
So far so good. But now, let's say we want to assign real values to these properties in an init function later:
x.a = 'xyz';
x.b = 123;
x.c = new AnotherType();
Here's the catch: we don't like having to specify those default null values when declaring the object if they're going to be set later to real values. Can we somehow have the interface default the properties we don't explicitly supply to null? 🤔
The Solution
Yes, there's a simple solution that will save you time and make your code cleaner. 🎉
Instead of explicitly declaring the types of all properties in the interface, you can make them optional by using the ?
symbol. This way, TypeScript will automatically assign a default value of null
to the properties you don't provide during initialization. 🌟
Let's rewrite our interface using optional properties:
interface IX {
a: string;
b?: any;
c?: AnotherType;
}
Now, you can initialize your variable x
without specifying all the properties:
let x: IX = {
a: 'abc'
};
No more compiler errors! TypeScript understands that b
and c
are optional and will default them to null
. 🎉
Conclusion
By making properties optional in your TypeScript interfaces, you can simplify your code and avoid specifying default values for properties that will be assigned later. 🙌
Next time you find yourself in a similar situation, just remember the ?
symbol and let TypeScript handle the default values for you! ✨
Now I want to hear from you! 📣 Have you encountered this issue before? Do you have any other tricks or tips related to TypeScript interfaces? Let's start a discussion in the comments below! 👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
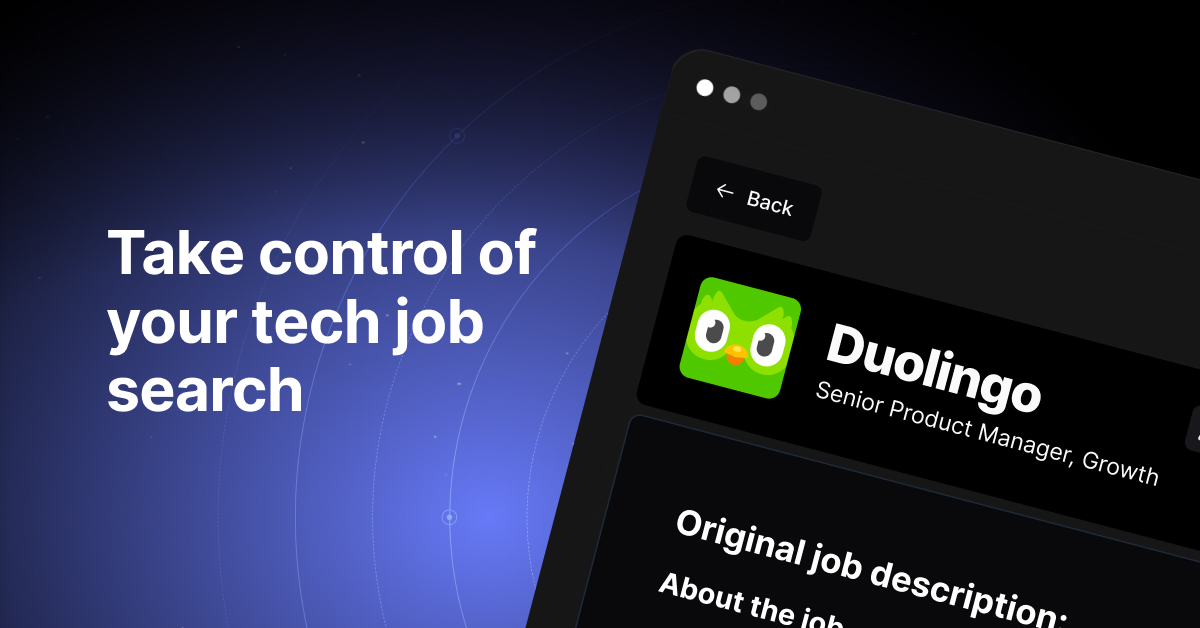