TypeScript function overloading
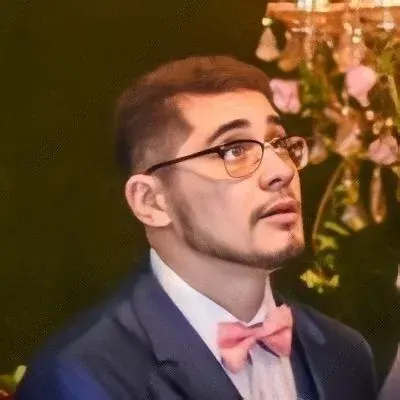
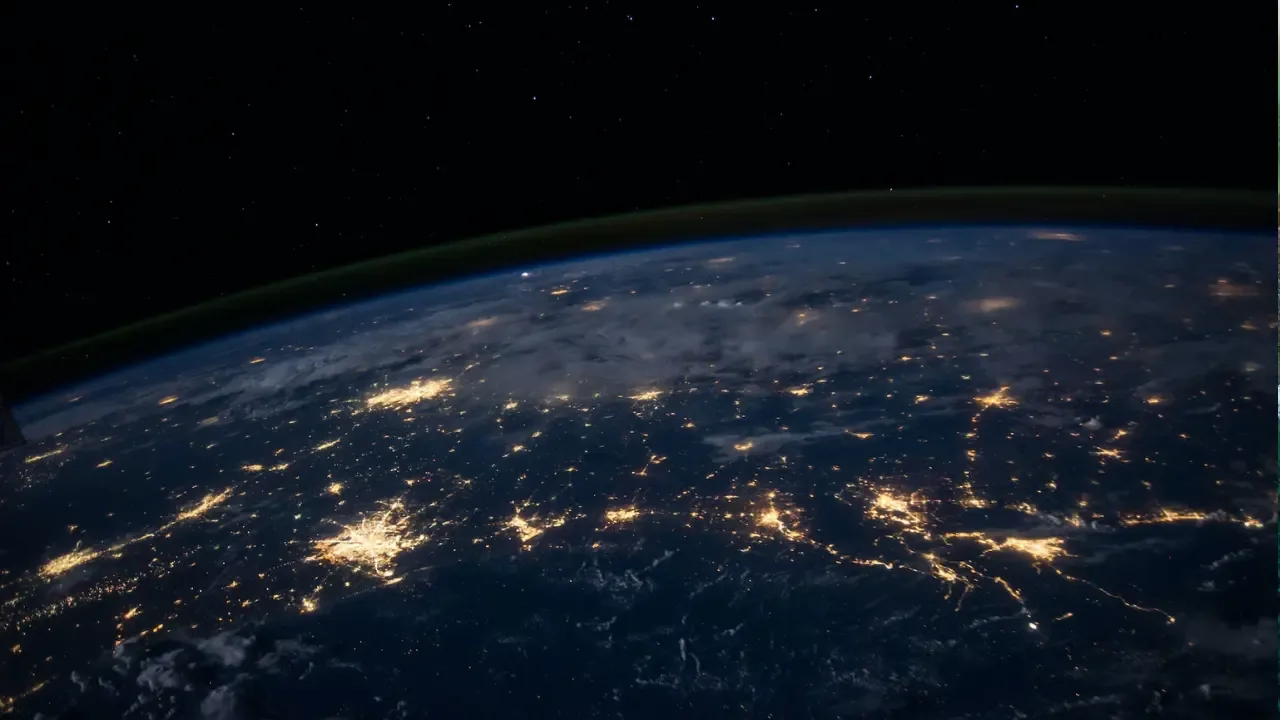
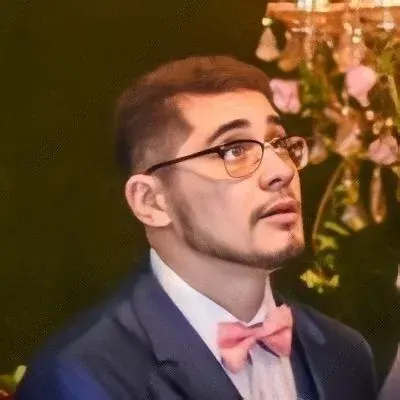
💡 Understanding TypeScript Function Overloading
Do you ever find yourself writing multiple functions with the same name but different parameter types? If you're using TypeScript, you might have encountered a common issue when trying to implement function overloading. But fear not! This blog post will guide you through the problem, provide easy solutions, and leave you feeling like a TypeScript pro.
🤔 The Duplicate Identifier Error
Let's address the specific problem mentioned in the context. When defining multiple functions with the same name but different parameter types, you might encounter a "duplicate identifier" error, even though the functions do have distinct parameters.
export class LayerFactory {
createFeatureLayer(userContext: Model.UserContext, mapWrapperObj: MapWrapperBase): any {
throw "not implemented";
}
createFeatureLayer(layerName: string, style: any): any {
throw "not implemented";
}
}
🔎 Why Does This Happen?
TypeScript is a statically-typed language, meaning it needs to know the exact signature of each function at compile-time. When you define multiple functions with the same name, TypeScript attempts to give each function a unique signature based on its parameters.
In the example above, TypeScript sees that both createFeatureLayer
functions have distinct parameter types. However, TypeScript determines the function signature solely based on the function name, ignoring the parameter types in the process.
✅ Easy Solutions
Here are a few solutions you can employ to resolve the "duplicate identifier" error:
1️⃣ Solution 1: Function Overloading
TypeScript has a special syntax for function overloading that allows you to define multiple function signatures for the same function name. To apply this concept to our example, you can rewrite the createFeatureLayer
function using function overloading:
export class LayerFactory {
createFeatureLayer(userContext: Model.UserContext, mapWrapperObj: MapWrapperBase): any;
createFeatureLayer(layerName: string, style: any): any;
createFeatureLayer(arg1: any, arg2: any): any {
// Implementation goes here
throw "not implemented";
}
}
By defining the function signature without implementation in the first two lines, you specify the allowed combinations of argument types. The actual implementation follows in the subsequent line.
2️⃣ Solution 2: Optional Parameters
If your functions have a single required parameter and additional optional parameters, you can use TypeScript's optional parameter syntax to avoid the duplicate identifier error. Here's an example:
export class LayerFactory {
createFeatureLayer(userContext: Model.UserContext, mapWrapperObj: MapWrapperBase): any;
createFeatureLayer(layerName: string, style?: any): any {
// Implementation goes here
throw "not implemented";
}
}
In this modified version, the style
parameter is marked as optional by appending a ?
after its name. This allows you to call the function with or without the optional parameter.
📣 Engage with the Community
Have you encountered any other challenging TypeScript issues? Do you have alternative solutions for function overloading? Share your thoughts, experiences, and solutions in the comments below. Let's learn from each other and make TypeScript programming a breeze!
So remember, function overloading in TypeScript doesn't have to be a confusing struggle. With the right approach and some creative problem-solving, you can harness the full power of TypeScript's flexible function signature handling.
Now it's your turn to take on the challenge! Let's break free from the "duplicate identifier" error and unlock the potential of TypeScript function overloading. 💪
Happy coding! 👩💻👨💻